From an array of objects, extract value of a property as array
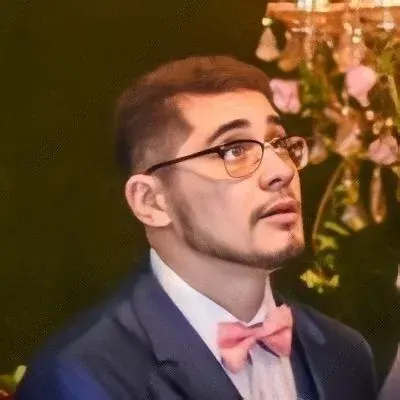
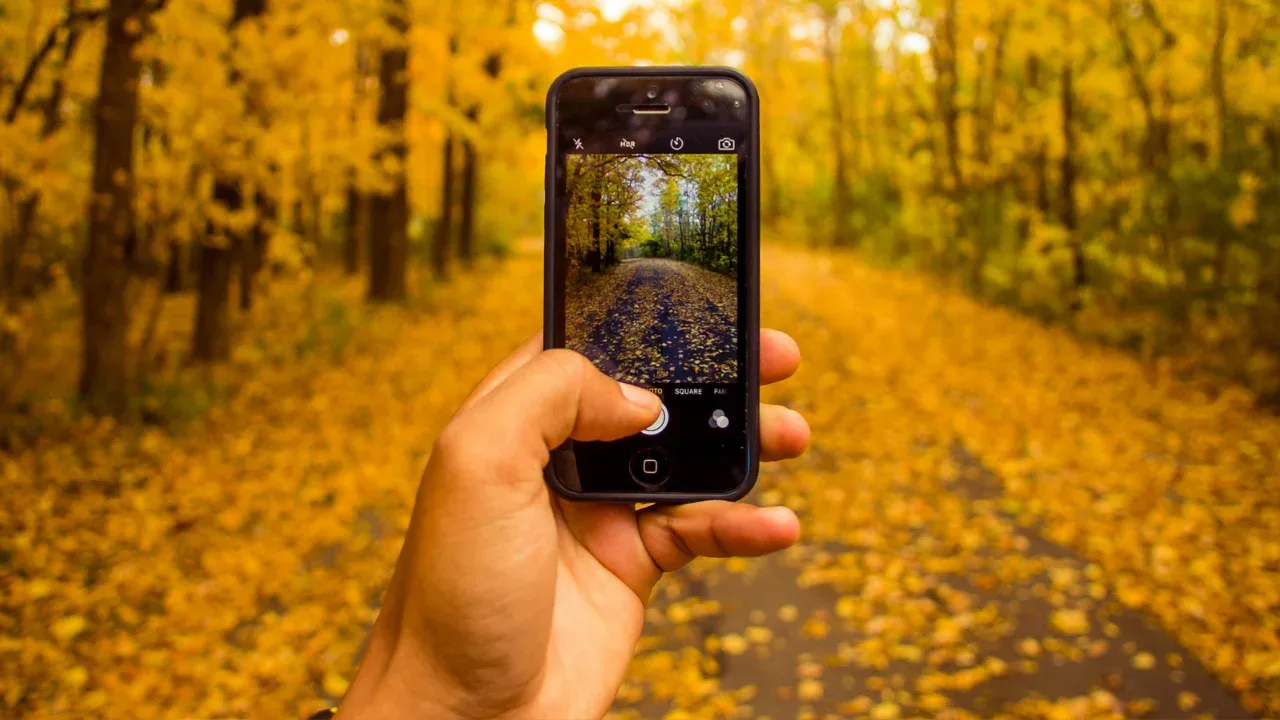
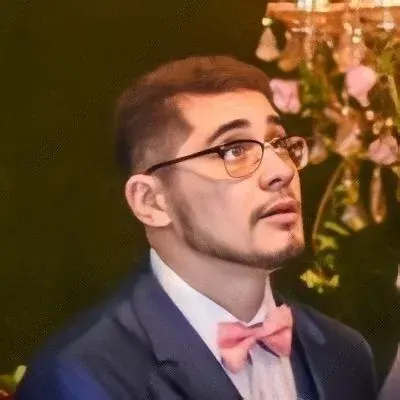
🎉🚀 Extracting values of a property from an array of objects made easy! 🎉🚀
Do you have a JavaScript object array and want to extract a specific field from each object, and get an array containing the values? 🤔 Don't worry, we've got you covered! In this blog post, we'll discuss a common issue and provide you with easy solutions to accomplish this task. Let's dive in! 💪
The Problem 😫
Let's take a look at the context around this question:
objArray = [ { foo: 1, bar: 2}, { foo: 3, bar: 4}, { foo: 5, bar: 6} ];
You want to extract the values of a specific field (e.g., foo
) from each object in the objArray
and create a new array containing those values. So, in this case, the expected result would be [ 1, 3, 5 ]
.
The Trivial Approach 🙄
The question already provides a trivial approach to solve this problem using a custom utility function:
function getFields(input, field) {
var output = [];
for (var i=0; i < input.length; ++i)
output.push(input[i][field]);
return output;
}
var result = getFields(objArray, "foo"); // returns [ 1, 3, 5 ]
This approach works perfectly fine, but is there a more elegant or idiomatic way to achieve the same result? Let's find out! 😎
The Elegant Solution 💡
Fortunately, JavaScript provides some powerful built-in functions that can make our lives easier. One such function is map()
. The map()
method creates a new array by calling a provided function on every element in the existing array. This makes it a perfect fit for our scenario!
Here's how you can use map()
to extract the field values in a more elegant way:
const result = objArray.map(obj => obj.foo); // returns [ 1, 3, 5 ]
Isn't that cool? 😄 By using map()
, we can simplify our code and achieve the desired result without the need for a custom utility function.
Your Turn! ✨
Now that you've learned a more elegant solution to extract values of a property from an array of objects, it's time to put it into practice! Try using the map()
method to solve similar problems in your projects and see how it can make your code cleaner and more efficient. Happy coding! 💻🎉
Have any questions or suggestions? Let us know in the comments below! We love hearing from our readers. Stay tuned for more informative and fun tech content! Don't forget to share this post if you found it helpful. Keep spreading the knowledge! 👍📢
<hr>
Note about the suggested duplicate, it covers how to convert a single object to an array.
<hr>