Finding the max value of an attribute in an array of objects
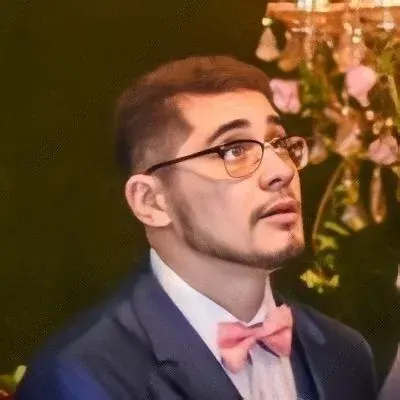
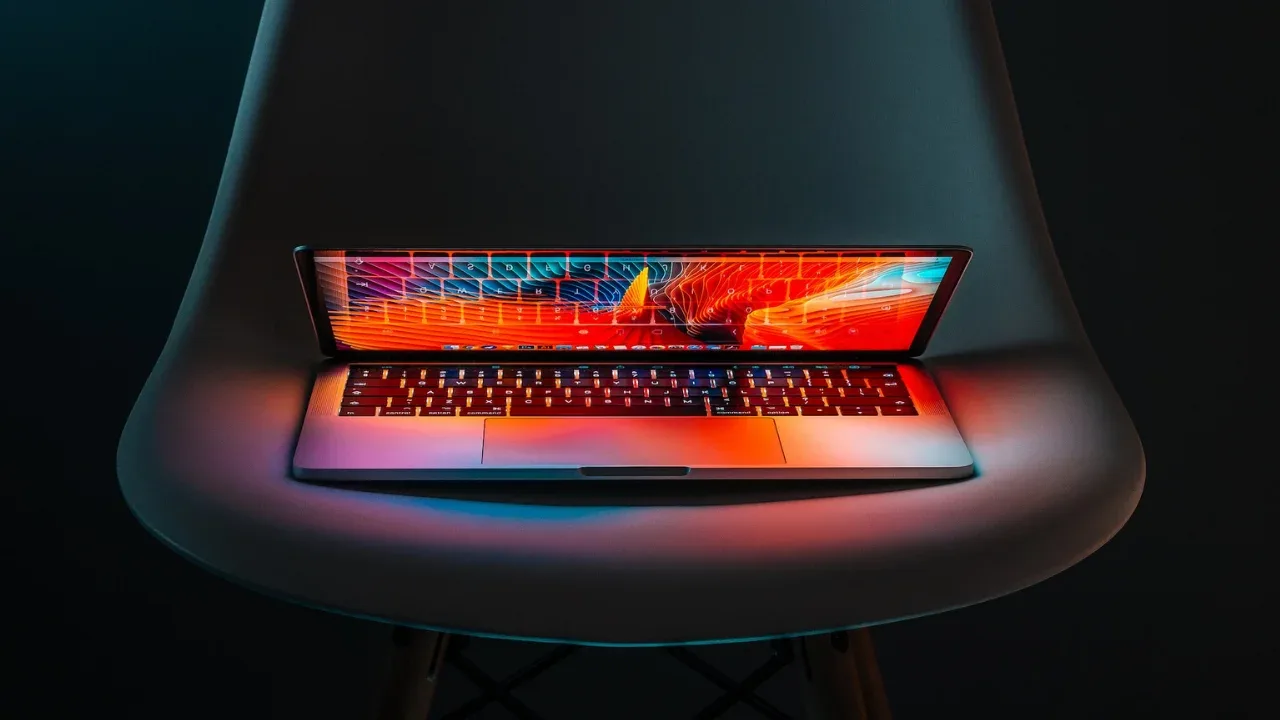
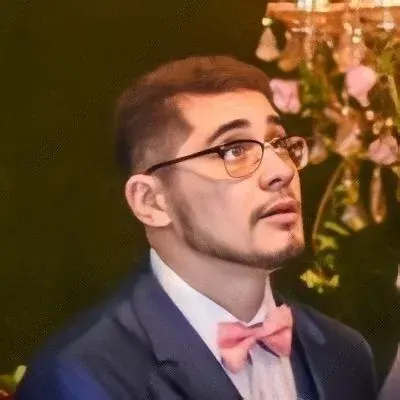
Finding the Max Value in an Array of Objects: A Quick and Efficient Solution! 💪
Are you grappling with finding the maximum value of a specific attribute in an array of objects? 🔄 Look no further! In this article, we'll explore an efficient and clean solution that will have you commanding your data like a pro. 😎
Here's the scoop: you have a JSON slice, with each object containing an "x" and "y" attribute. You need to find the maximum value of "y" in the array. Let's dive in and solve this puzzle together! 🧩
The Traditional Approach: The For-Loop 🔄
The most common approach to solve this problem is by using a for-loop. It's a classic method, but may seem a bit tedious, especially when you're striving for elegance and efficiency. Here's how you might tackle it:
let jsonArray = [
{
"x": "8/11/2009",
"y": 0.026572007
},
{
"x": "8/12/2009",
"y": 0.025057454
},
{
"x": "8/13/2009",
"y": 0.024530916
},
{
"x": "8/14/2009",
"y": 0.031004457
}
];
let maxValue = -Infinity;
for (let obj of jsonArray) {
if (obj.y > maxValue) {
maxValue = obj.y;
}
}
console.log(maxValue);
In the above code, we start with an initial value of -Infinity for the max value. Then, we iterate through each object in the array and compare its "y" value with the current maximum value. If we find a larger value, we update the maxValue variable.
The Elegant Solution: Utilizing Math.max and Spread Operator! 🌟
Now, let's level up our game and find a more efficient and cleaner solution! Luckily, we can leverage JavaScript's Math.max function along with the spread operator (...) to achieve this. Here's how it's done:
const jsonArray = [
{
"x": "8/11/2009",
"y": 0.026572007
},
{
"x": "8/12/2009",
"y": 0.025057454
},
{
"x": "8/13/2009",
"y": 0.024530916
},
{
"x": "8/14/2009",
"y": 0.031004457
}
];
const max = Math.max(...jsonArray.map(obj => obj.y));
console.log(max);
In this elegant solution, we use the map function to extract all the "y" values from the array of objects. Then, we utilize the spread operator to pass each value as an argument to the Math.max function. Finally, we obtain the maximum value effortlessly!
Embrace Efficiency and Enjoy the Results! 😍
With this efficient solution in your arsenal, finding the maximum value of an attribute in an array of objects becomes a breeze! No more pesky for-loops or repetitive code. 🙅♂️
So, what are you waiting for? Give this solution a try and witness the magic! Share your thoughts, questions, or favorite approaches in the comments below. Let's unravel the secrets of max values together! 🚀