Find object by id in an array of JavaScript objects
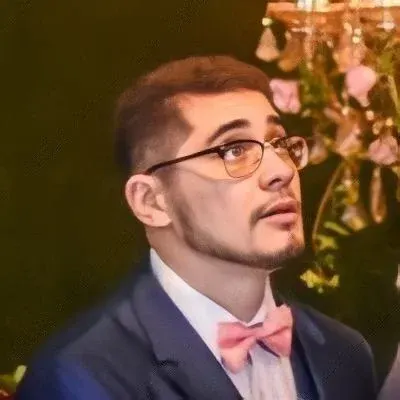
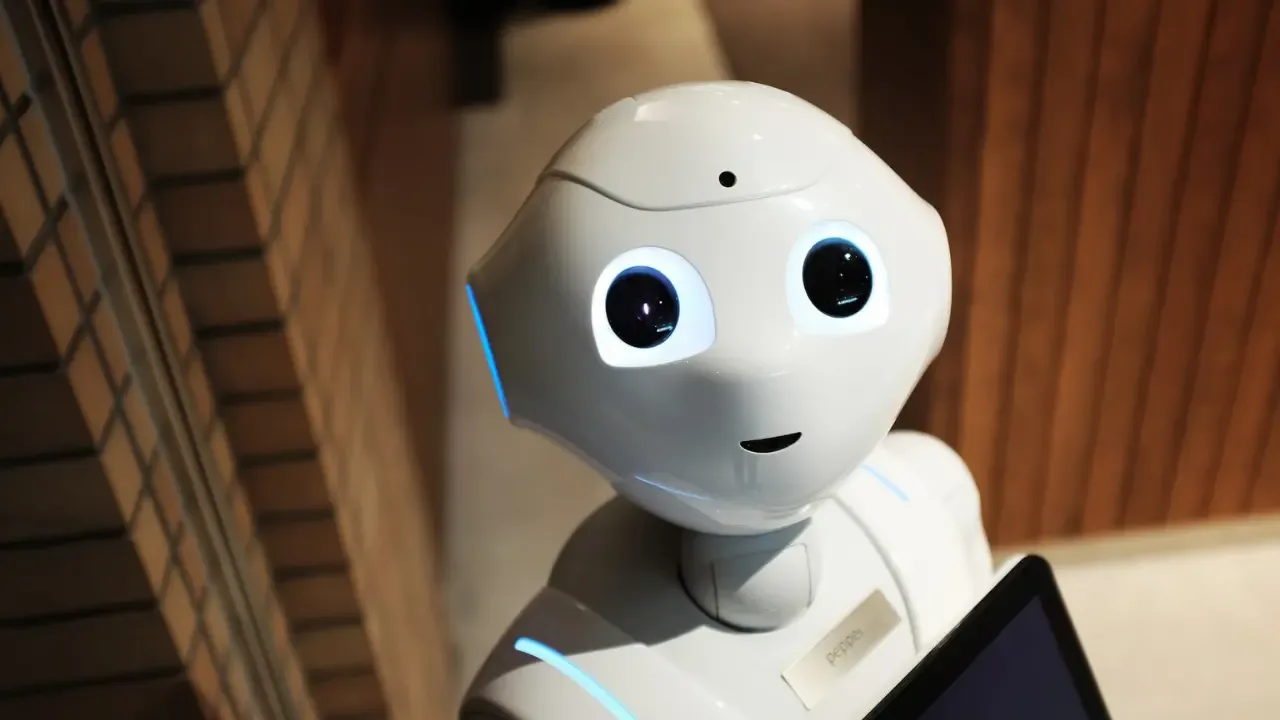
Finding an Object by ID in a JavaScript Array: A Simple Guide
Are you struggling to find an object in a JavaScript array based on its ID? Don't worry, you're not alone! In this post, we'll explore a common issue faced by developers and provide easy solutions using JavaScript or jQuery. So, let's dive right in and find that elusive object! 💪🔍
The Problem: Finding an Object by ID in an Array
Imagine you have an array of JavaScript objects like this:
myArray = [{'id':'73','foo':'bar'},{'id':'45','foo':'bar'}, etc.]
You are given an ID (in this case, 45
) and you want to retrieve the corresponding object from the array.
Solution 1: Using JavaScript's Array.find()
JavaScript provides an elegant solution to this problem using the Array.find()
method. This method searches the array for the first element that satisfies a given condition and returns that element. Here's how you can use it to find an object by ID:
const objectId = '45';
const foundObject = myArray.find(obj => obj.id === objectId);
In the code above, we define a constant objectId
with the desired ID value (45
). Then, we use the Array.find()
method on myArray
and provide a callback function that checks if the id
property of each object matches objectId
. If a match is found, the corresponding object is returned and stored in the foundObject
variable.
Solution 2: Using jQuery's $.grep() Method
If you're working with jQuery, you can achieve the same result using the $.grep()
method. This method searches through an array and returns an array containing only the elements that match a specific condition. Here's how you can use it to find an object by ID:
const objectId = '45';
const foundObjects = $.grep(myArray, function(obj) {
return obj.id === objectId;
});
In the code above, we define the objectId
constant as before, and then we use the $.grep()
method on myArray
. The callback function checks if the id
property of each object matches objectId
. If a match is found, the object is included in the foundObjects
array.
🎉 Congratulations, You Found the Object!
By following one of the solutions above, you have successfully found the object in the array based on its ID. Now you can access any property of the found object, such as foo
in this case, and work with its value as needed.
Take It Further: Handle Object Not Found
In some cases, the object you are looking for may not exist in the array. To handle this scenario, you can enhance the solutions by adding an additional step to check if the object was found or not. For example:
if (foundObject) {
// Object found, do something with it
console.log(foundObject.foo);
} else {
// Object not found, handle the error
console.log('Object not found. Please try again.');
}
This way, you can gracefully handle situations where the object is not found and provide appropriate feedback or fallback options.
Engage with Us!
We hope this guide has helped you locate objects by ID in JavaScript arrays. Did you find a different solution? Or do you have any other JavaScript issues you'd like us to address? We'd love to hear from you! Leave a comment below and let's start a conversation. 👇💬
Happy coding! 😄🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
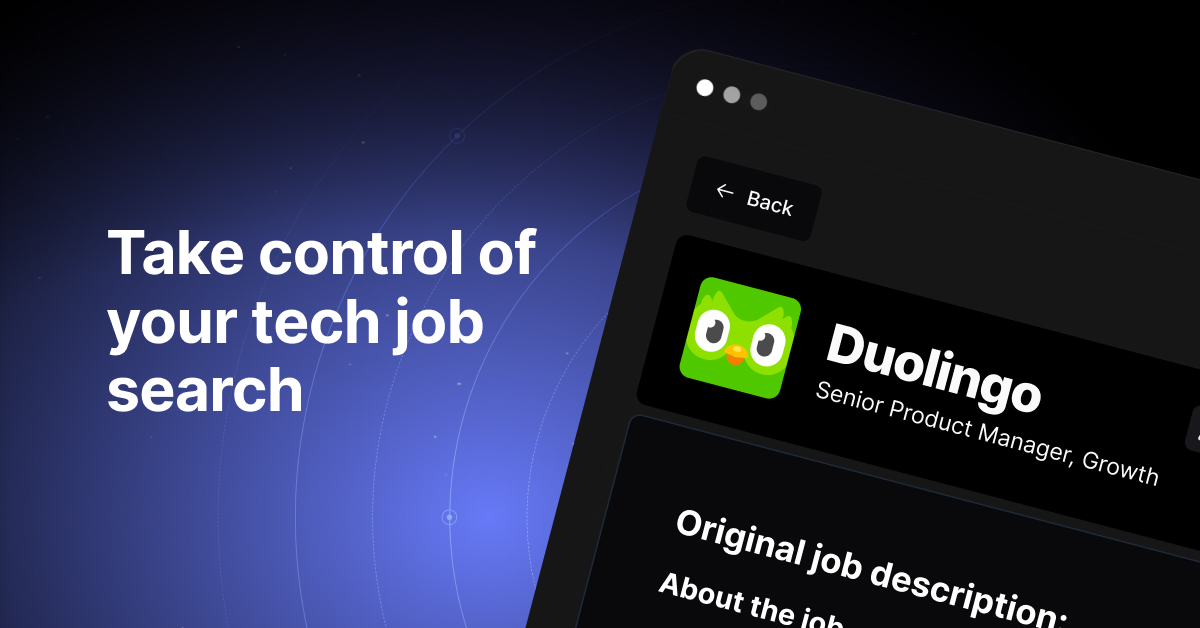