Fetch: POST JSON data
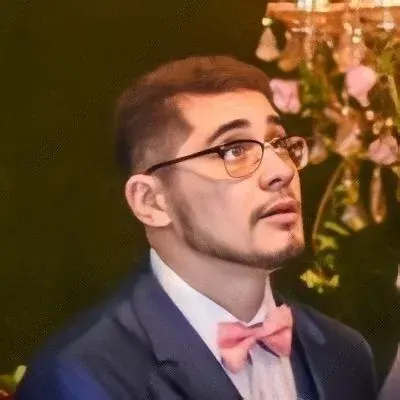
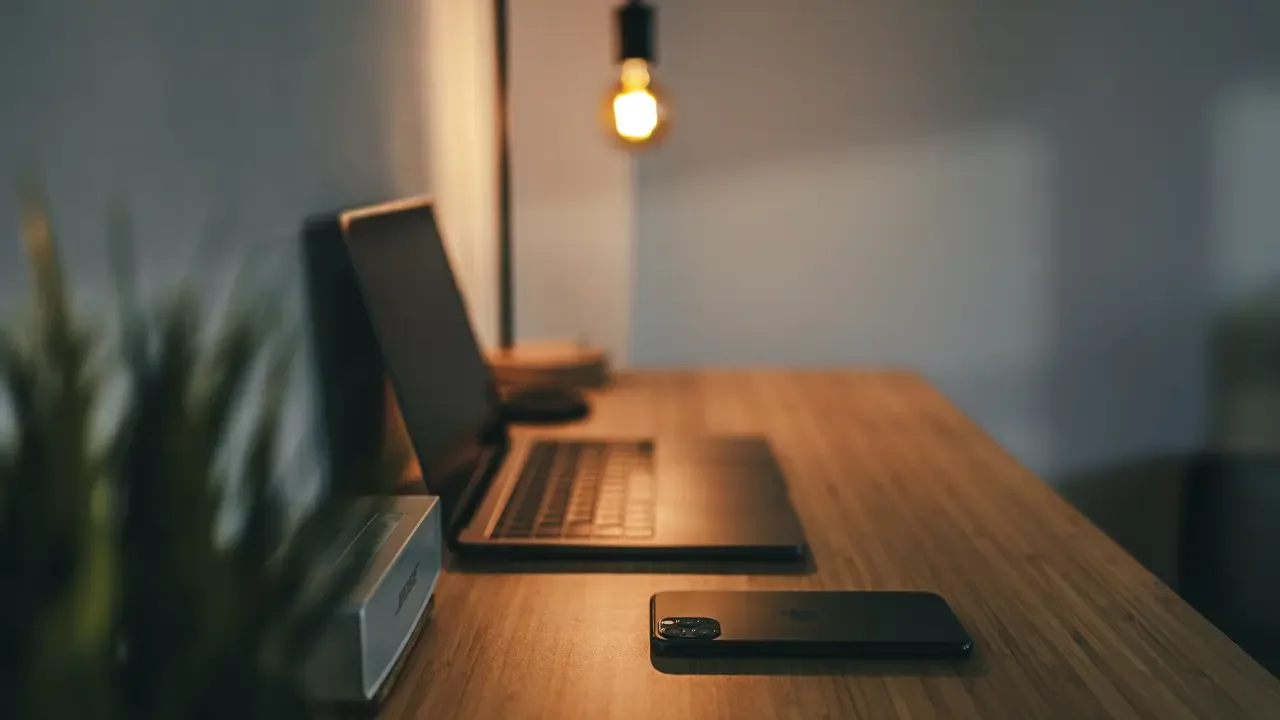
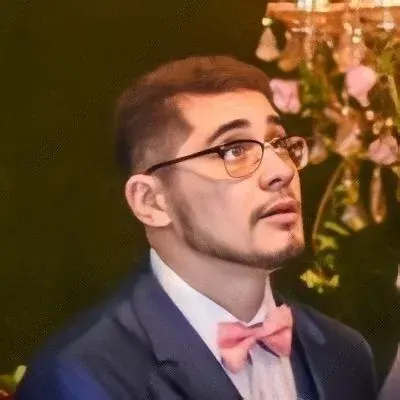
📢 🔍 Fetch: POST JSON data 📩
So, you're trying to send a JSON object using the fetch function? 🤔 Sounds tricky, but fear not! I'm here to guide you through it and help you troubleshoot any issues you might encounter along the way. Let's get started! 💪
✨ The problem: You've got your fetch request set up, with the correct headers, method, and body. However, when you check the network tab in Chrome DevTools, you notice that the JSON object is not being sent. It's nowhere to be found! 😱
🔑 The solution: Don't worry, you're not alone. Many developers face this issue, but luckily, there's a simple solution. All you need to do is ensure that the server is correctly configured to handle the JSON data. 🖥️
Here's what you can do:
1️⃣ Check the server configuration: Double-check that the server is expecting JSON data in the request body. It should be able to parse and handle it properly.
2️⃣ Validate your JSON: Verify that your JSON object is valid. Use an online JSON validator or a linter to ensure there are no syntax errors. 🧐
3️⃣ Update your request code: If everything seems fine with the server and the JSON, it's time to revisit the fetch code. Make sure you're attaching the stringified JSON object to the body of the request correctly.
Here's an updated example based on your code:
fetch("/echo/json/", {
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
},
method: "POST",
body: JSON.stringify({a: 1, b: 2})
})
.then(function(res) { console.log(res) })
.catch(function(res) { console.log(res) })
Make sure you're using the correct URL for your server. 🌍
💡 Pro tips:
Double-check the server response: If the JSON object is still not showing up in the network tab after following the steps above, take a look at the server's response. There might be some clues there.
Test with a different server: If possible, try sending the JSON object to a different server or API that you trust to see if the issue persists. This can help narrow down the problem.
Consult the server-side documentation: If you're still stuck, consult the documentation or seek help from the maintainers of the server or API you're using. They might have specific requirements or recommendations for sending JSON data.
💪 Now you're armed with the knowledge to tackle the common issue of fetching and posting JSON data using the fetch function. Go ahead and try it out in your project! 🚀
📢 I'd love to hear from you! Have you ever encountered any issues when sending JSON data? How did you solve them? Share your experiences in the comments below and let's help each other out! 👇