Fetch API request timeout?
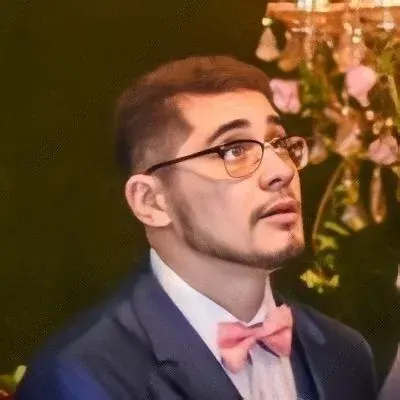
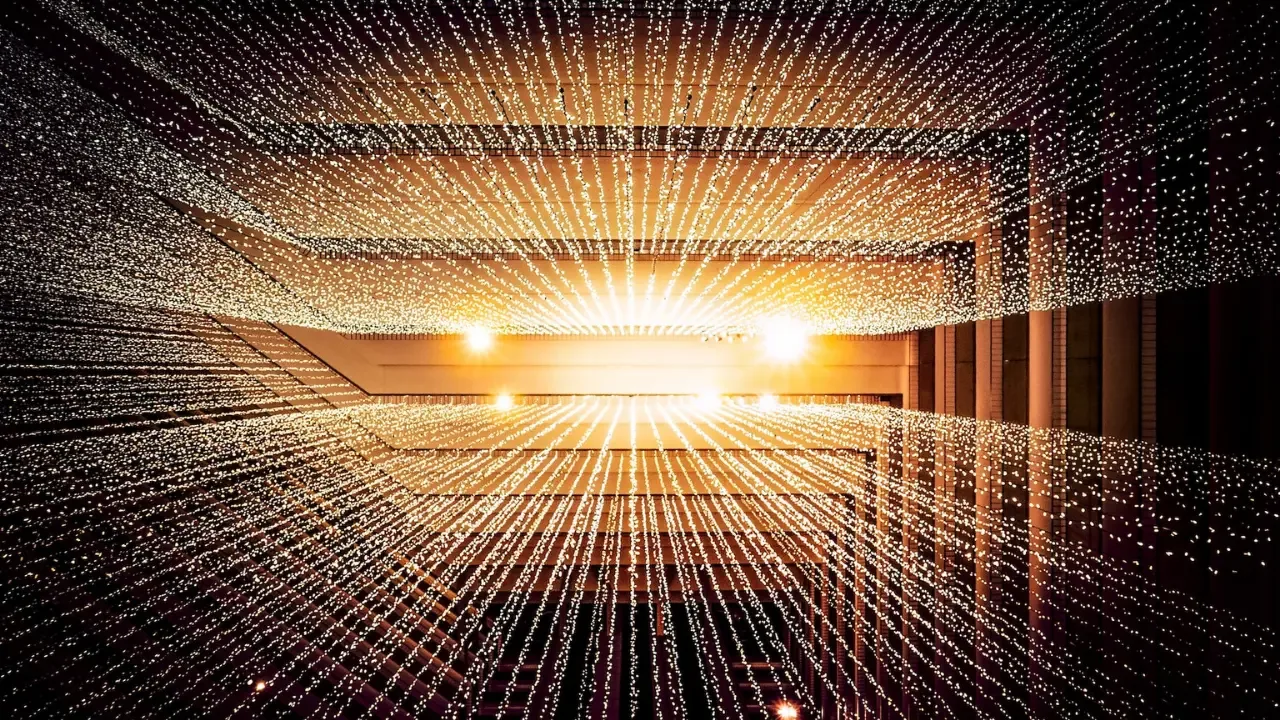
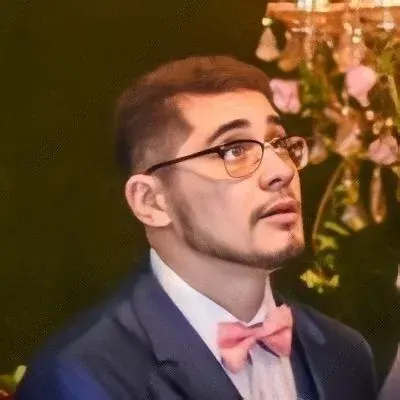
🔍 What's the Timeout?
So you're using the 🔥 Fetch API 🔥 for your POST request, huh? But wait, have you ever wondered what happens if the 🌐 network takes too long to respond? 🤔 That's where the concept of timeout comes into play! ⏰
By default, the Fetch API doesn't have a built-in timeout value. This means that if your request hangs or takes forever to receive a response, it might leave your application waiting indefinitely. 😱
But fear not, fellow developer! I've got your back with easy solutions to this 🕰️ timeout predicament!
⏳ Setting a Timeout Value
To set a timeout for your Fetch API request, you can take advantage of one of JavaScript's coolest features: Promises. 🌈 By creating a Promise that rejects after a certain period of time, you can effectively create a timeout mechanism.
Let's take a look at an example of how to set a timeout of 3 seconds for your Fetch API request:
const timeoutPromise = new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error('Request timed out')) // reject the promise after 3 seconds
}, 3000)
})
const fetchPromise = fetch(url, {
method: 'POST',
body: formData,
credentials: 'include'
})
Promise.race([fetchPromise, timeoutPromise])
.then(response => {
// handle the successful fetch response
})
.catch(error => {
// handle the timeout error or any other fetch errors
})
In the example above, we create a timeoutPromise
that will reject after the specified time (3 seconds in this case). Then, using Promise.race()
, we can wait until either the Fetch API request or the timeout promise is settled. Whichever settles first will determine the outcome.
If the Fetch API request resolves before the timeout, you can handle the response in the .then()
block. But if the timeoutPromise rejects first, the control flow will enter the .catch()
block, allowing you to handle the timeout error or any other fetch errors.
🛠️ Handling an Indefinite Timeout
What if you don't want your Fetch API request to time out at all? Sometimes, you might want to wait indefinitely for a response. 🔄 In such cases, you can set the timeout value to a really large number or even Infinity. This ensures that the request doesn't time out unless there's an issue with the network or the server itself.
Here's an example of how to set an indefinite timeout:
const timeoutPromise = new Promise((resolve, reject) => {})
// Since the timeoutPromise never rejects, the fetchPromise will continue indefinitely
const fetchPromise = fetch(url, {
method: 'POST',
body: formData,
credentials: 'include'
})
Promise.race([fetchPromise, timeoutPromise])
.then(response => {
// handle the successful fetch response
})
.catch(error => {
// handle any other fetch errors
})
In this case, the timeoutPromise
is an empty Promise that never rejects. Therefore, the fetchPromise
will continue indefinitely until it resolves or encounters an error.
📣 Calling Out for Engagement!
Now that you're well-equipped with knowledge about setting timeouts for your Fetch API requests, it's time to give it a try! Implement it in your code and see the magic happen! ✨
Did you encounter any issues? Or do you have anything to add to this guide? Let me know in the comments below! Let's help each other out and make the web development community stronger together! 🤝💪
🚀 Time's Up!
Now you know how to add that ⌛ extra oomph to your Fetch API requests by setting up timeouts. Whether you have a specific timeout value in mind or want to wait indefinitely, you're now ready to tackle any timeout challenge. 🤓
Remember, timeouts are important to ensure your application doesn't hang forever, waiting for a response that might never come. So go ahead, implement these timeout techniques, and save your application from those annoyingly long waits!⚡
Happy coding! ✌️