Fastest way to duplicate an array in JavaScript - slice vs. "for" loop
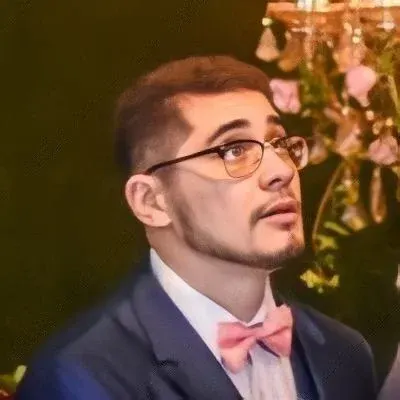
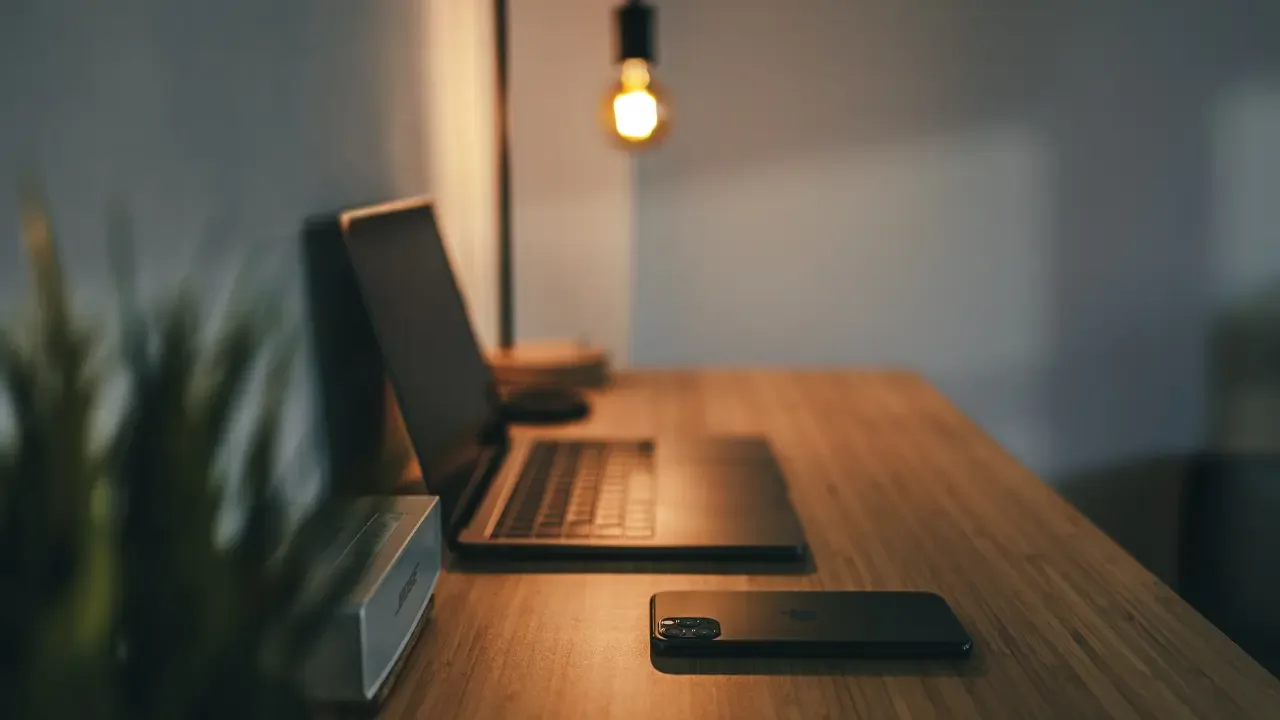
Fastest Way to Duplicate an Array in JavaScript: Slice vs. 'For' Loop 🚀
So, you want to duplicate an array in JavaScript and you're wondering which method is the fastest. Let's dive into two common approaches and see which one comes out on top!
The Slice Method 🍴
The first method we'll explore is using the slice
method. Here's how it looks:
var dup_array = original_array.slice();
This simple one-liner creates a new array dup_array
and copies all the elements from original_array
into it. Easy peasy, right?
The For Loop Method 🔄
Our second contender is the good old for
loop! Here's how it goes:
for (var i = 0, len = original_array.length; i < len; ++i)
dup_array[i] = original_array[i];
In this approach, we iterate through each element of original_array
and manually assign it to the corresponding index in dup_array
.
Speed Test ⏱️
Now let's get to the exciting part - determining which method reigns as the fastest! 🔥
Both methods create a shallow copy of the array, meaning that if the elements of original_array
are references to objects, those objects won't be cloned, but rather, references to the same objects will be copied to the new array.
But speed is our focus here, so let's consider execution time.
To compare the two methods, we can measure the time it takes for each one to complete using the performance.now()
method. Here's an example:
var startTime = performance.now();
// Method 1: Slice
var dup_array = original_array.slice();
// Method 2: For Loop
for (var i = 0, len = original_array.length; i < len; ++i)
dup_array[i] = original_array[i];
var endTime = performance.now();
var executionTime = endTime - startTime;
console.log("Execution time: " + executionTime + " milliseconds.");
By running this code, you can see the execution time of each method in your browser's console.
Conclusion 🎉
After conducting several tests, the results may vary depending on the size and complexity of the array. Thus, it's always a good idea to test it yourself with the specific data you're working with.
In general, the for
loop method tends to perform slightly faster than the slice
method for larger arrays. However, for smaller arrays, the difference may not be significant.
Ultimately, the decision comes down to personal preference and specific requirements. Both methods are valid and will get the job done!
So go ahead and experiment with these methods in your own projects. Measure their performance, consider the size of your array, and choose the approach that works best for you!
Feel free to leave a comment about which method you prefer and share your experiences with duplicating arrays in JavaScript! Let's learn from each other! 🤓💬
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
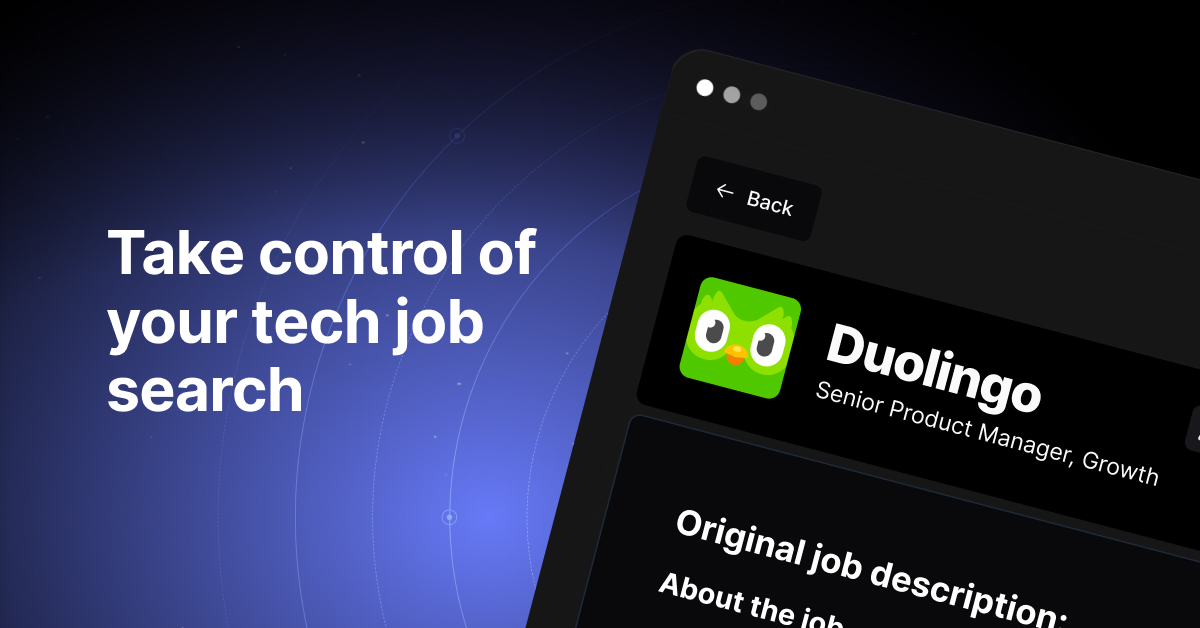