Fastest way to copy a file in Node.js
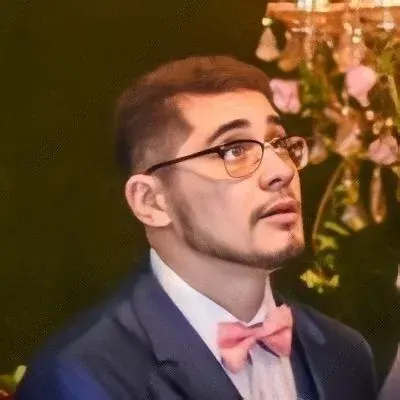
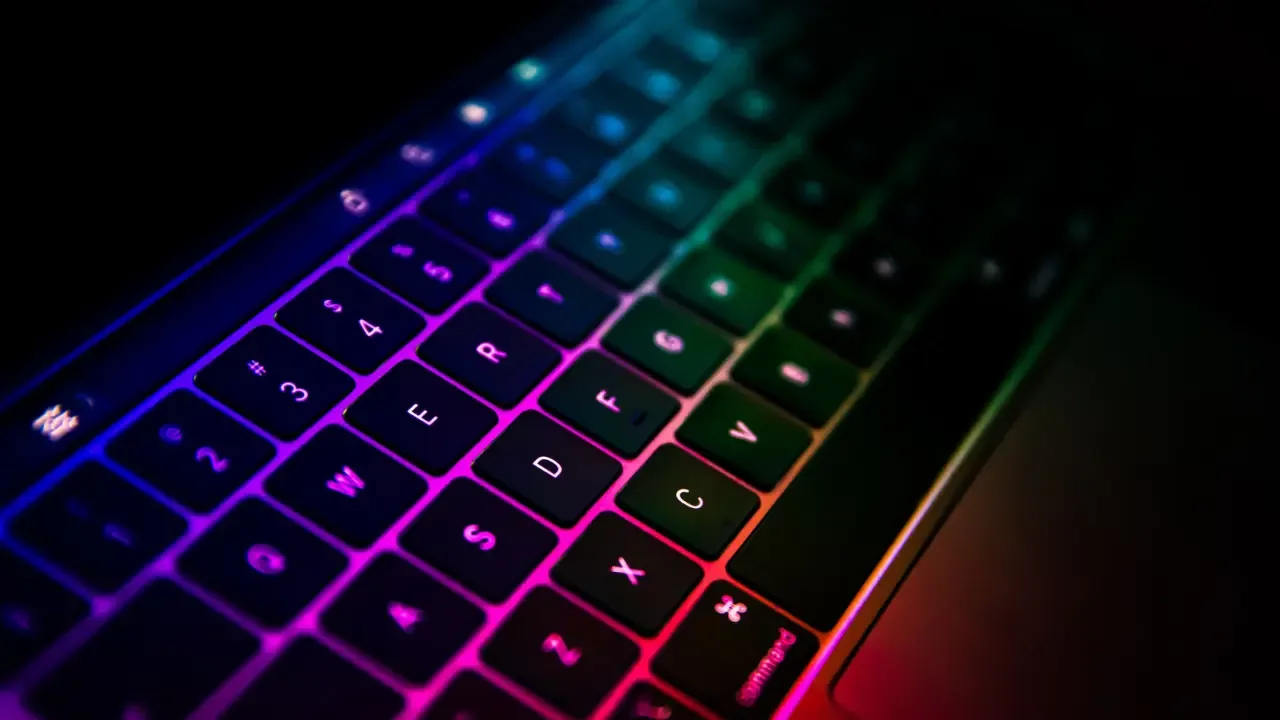
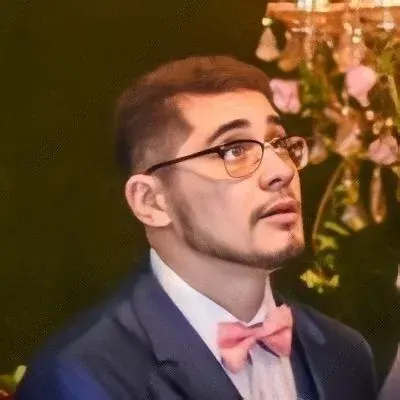
Fastest way to copy a file in Node.js 🚀
If you're working on a Node.js project that involves frequent file system operations like copying, reading, and writing files, you might be wondering which method is the fastest. In this article, we'll explore different methods for copying files in Node.js and discuss their pros and cons. By the end of this guide, you'll have the knowledge to choose the most efficient method for your file copying needs. Let's dive in! 💪
👉 Method 1: fs.copyFile
One of the simplest ways to copy a file in Node.js is by using the fs.copyFile
method. This method is provided by the built-in fs
module and is quite straightforward to use. Here's an example:
const fs = require('fs');
fs.copyFile('source.txt', 'destination.txt', (err) => {
if (err) throw err;
console.log('File copied successfully!');
});
The fs.copyFile
method takes three arguments: the source file path, the destination file path, and a callback function. The callback function is invoked once the file is copied or if an error occurs.
While fs.copyFile
is easy to use, it may not be the fastest method for large files or when high performance is crucial.
👉 Method 2: fs.createReadStream and fs.createWriteStream
Another way to copy a file in Node.js is by using the fs.createReadStream
and fs.createWriteStream
methods. These methods provide more control and can be faster for larger files. Here's an example:
const fs = require('fs');
const sourceStream = fs.createReadStream('source.txt');
const destinationStream = fs.createWriteStream('destination.txt');
sourceStream.pipe(destinationStream);
sourceStream.on('end', () => {
console.log('File copied successfully!');
});
sourceStream.on('error', (err) => {
throw err;
});
In this method, we create read and write streams for the source and destination files, respectively. We then use pipe
to directly transfer the contents from the source stream to the destination stream. This method can be more performant than fs.copyFile
for large files.
👉 Method 3: third-party libraries
If you're looking for even more speed and flexibility, you can consider using third-party libraries specifically designed for file operations in Node.js. A popular choice is the fast-copy
library. Here's an example:
const fastCopy = require('fast-copy');
fastCopy('source.txt', 'destination.txt', (err) => {
if (err) throw err;
console.log('File copied successfully!');
});
Third-party libraries like fast-copy
often provide optimized algorithms for file copying, making them faster than the built-in methods.
⚡️ Conclusion
When it comes to copying files in Node.js, you have several options to choose from. For simple copying needs, the fs.copyFile
method works just fine. But if you're dealing with larger files or require better performance, consider using the fs.createReadStream
and fs.createWriteStream
method or try out third-party libraries like fast-copy
.
Remember, always benchmark and test your code to determine the best approach for your specific use case. Happy coding! 👩💻👨💻
Do you have any other tips or tricks for copying files in Node.js? Let us know in the comments below! 😊