Fastest way to check a string contain another substring in JavaScript?
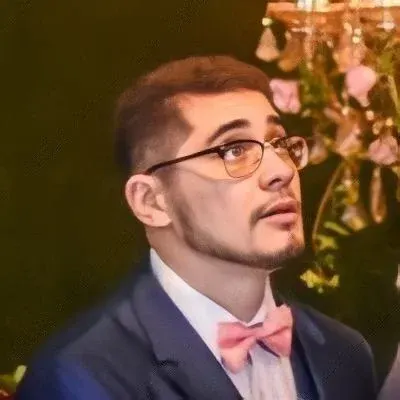
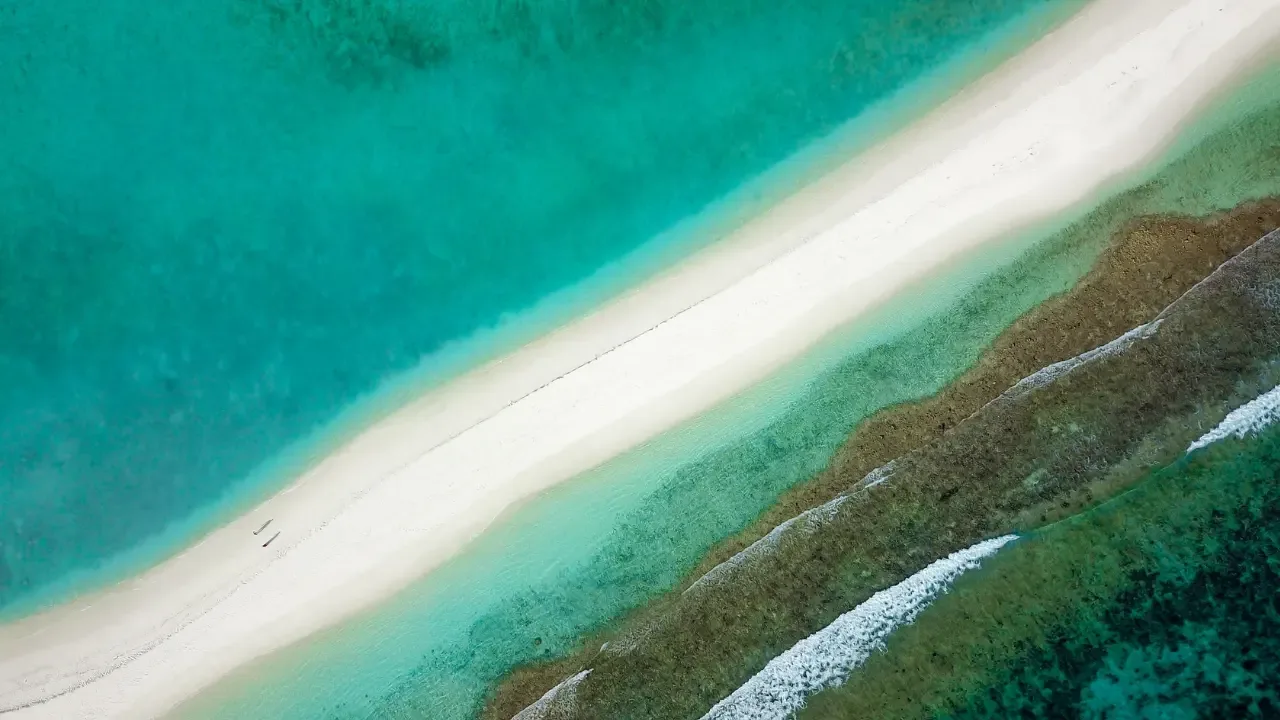
๐ Finding Substrings in JavaScript - The Need for Speed! ๐
So you're faced with a performance issue in JavaScript and need to determine the fastest way to check whether a string contains another substring? Look no further! We've got you covered with some blazing-fast solutions ๐๏ธ
The Challenge ๐ช
Let's break down the problem first: you have a string and you want to check if it contains a specific substring. Easy, right? Well, not quite. Performance can become a concern when dealing with large strings or implementing intensive algorithms.
Solution 1: String.includes() method ๐ง
The most straightforward and beginner-friendly way to check for substrings is by using the built-in includes()
method. It returns a boolean value indicating whether the given substring exists within the string:
const string = "Hello World!";
const substring = "Hello";
const containsSubstring = string.includes(substring);
console.log(containsSubstring);
This approach is concise, readable, and performs reasonably well in most scenarios. However, it may not provide optimal performance in hyper-optimized scenarios or when dealing with really long strings.
Solution 2: Regular Expressions ๐งช
Regular expressions are powerful tools when it comes to string manipulation and pattern matching. They allow for more complex matching scenarios and can be lightning-fast:
const string = "Hello World!";
const substring = /hello/i;
const containsSubstring = substring.test(string);
console.log(containsSubstring);
In this example, we use a case-insensitive regular expression to search for the substring. While regular expressions can yield great results, they can be overkill for simple substring checks and may introduce unnecessary complexity.
Solution 3: IndexOf or Search methods ๐
The indexOf()
and search()
methods are old-school but still valuable when it comes to substring checks. They return the index of the first occurrence of the substring, allowing us to determine whether it exists or not:
const string = "Hello World!";
const substring = "World";
const containsSubstring = string.indexOf(substring) !== -1;
// or
const containsSubstring = string.search(substring) !== -1;
console.log(containsSubstring);
Both approaches return a boolean by checking if the index is not equal to -1. However, it's important to note that indexOf()
and search()
have slight differences, such as the ability to use regular expressions with search()
.
Making the Right Choice ๐ค
Now that you have multiple options for checking substrings in JavaScript, which one should you choose?
If performance is your primary concern, you may want to consider using indexOf()
or search()
. These methods tend to outperform includes()
or regular expressions in certain scenarios.
However, always remember to balance performance optimizations with code readability and maintainability. Choose the approach that fits the specific requirements of your project and aligns with your overall codebase.
Your Turn! ๐
Now that you have the tools and knowledge, it's time to put them into action! Try out these different approaches in your own code and see which one works best for your specific use cases.
Have you encountered any performance issues before? What strategies did you implement to overcome them? Share your experiences and insights in the comments below! Let's learn and grow together as a community! ๐ก
That's all for now, folks! Happy coding and may your substrings always be found efficiently! ๐
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
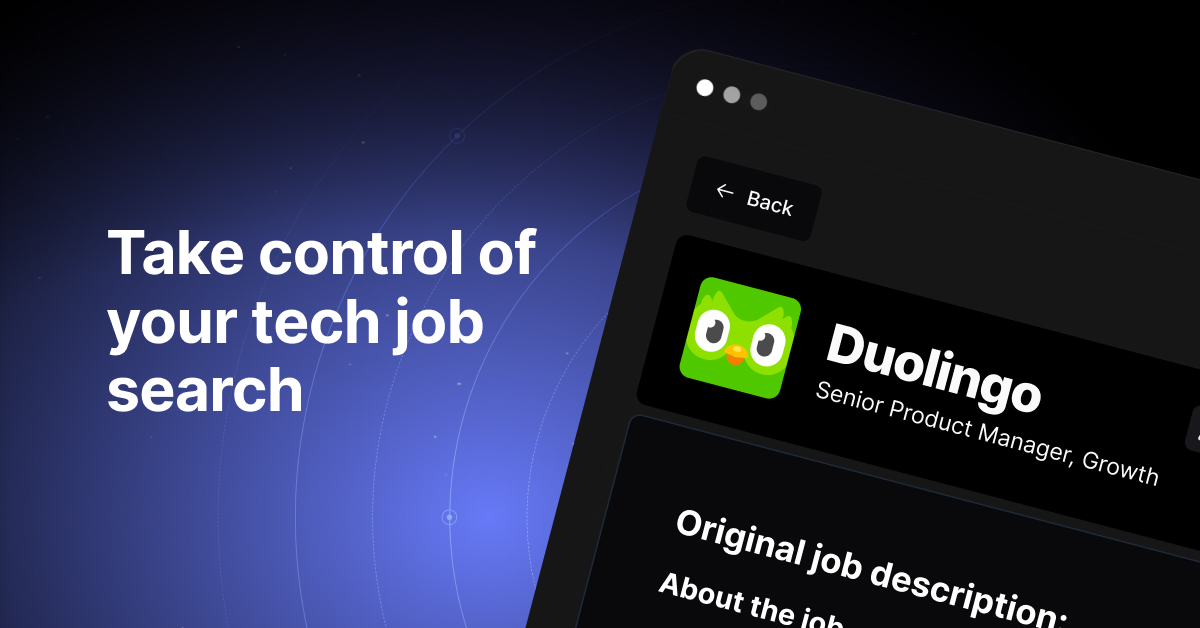