Fastest method to replace all instances of a character in a string
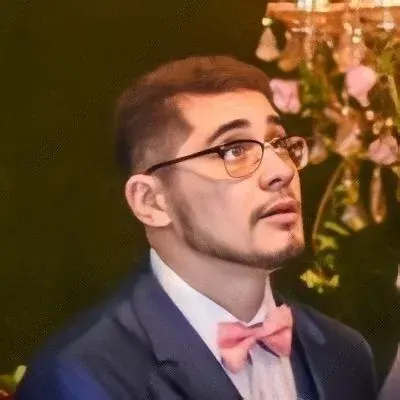
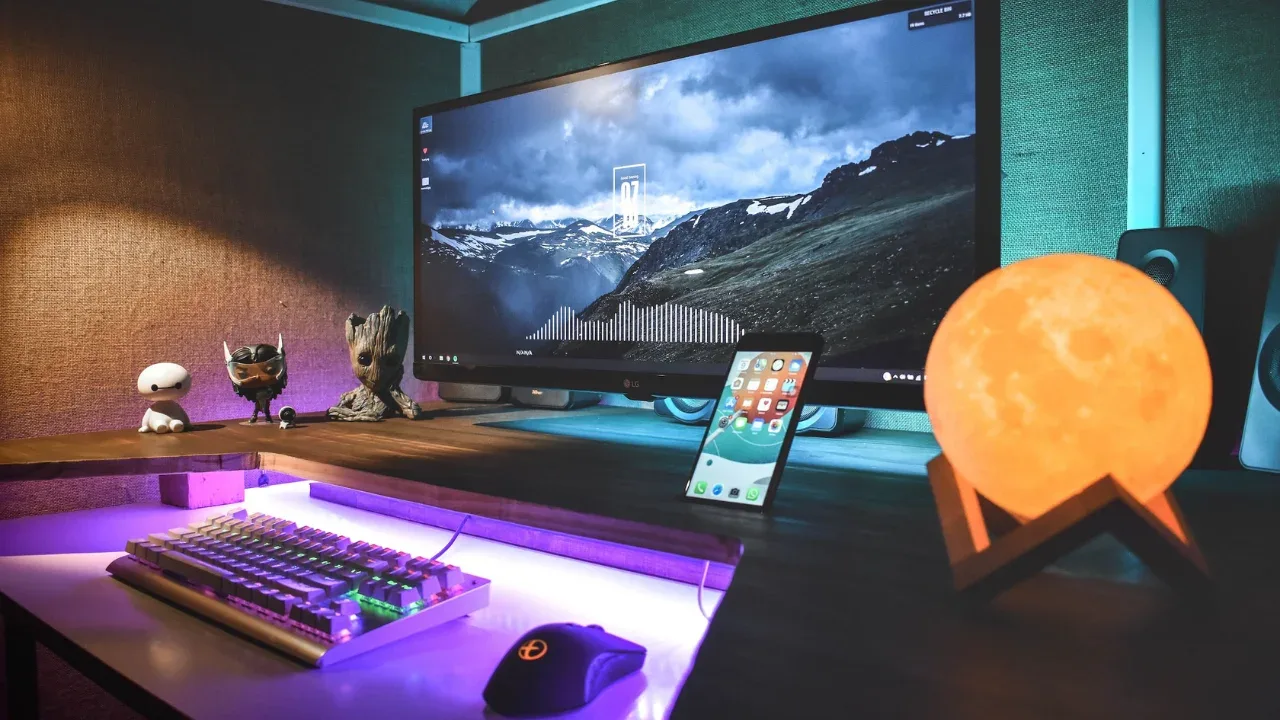
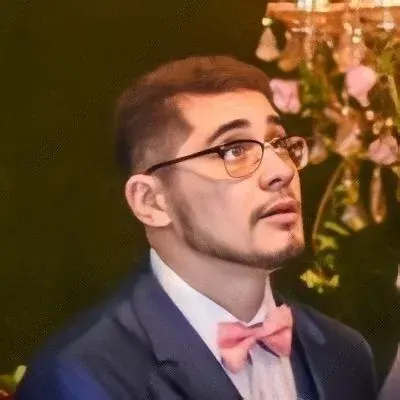
The Need for Speed: Fastest Method to Replace Characters in a Stringπ₯π¨
π Hey there tech enthusiasts! Today, we are diving into the world of JavaScript and unleashing the fastest method to replace all instances of a character in a string. If you've ever struggled with finding an efficient solution to this common problem, then this guide is for you! πͺ
The Need for Speed: Common Issues and Problems
Before we jump into the solutions, let's take a quick peek at the common issues encountered when replacing characters in a string:
Performance Matters π: Often, we deal with large strings or situations where performance is a critical factor. In such cases, we can't afford to have a sluggish solution that takes ages to complete the process.
Replacing Multiple Instances: Sometimes, we need to replace multiple occurrences of a specific character in a string. We don't want a solution that only handles the first or last occurrence, leaving the rest untouched.
Unleashing the Speed Demons: Solutions to the Rescue
Solution 1: Using the split()
and join()
Methods
One of the quickest and most elegant ways to replace characters in a string is by using JavaScript's split()
and join()
methods. Here's how it works:
const originalString = "Hello world!";
const replacedString = originalString.split("o").join("*");
console.log(replacedString);
In this example, we split the original string based on the character we want to replace ("o"). Then, we join the split parts using the desired replacement character ("*"). The result is a blazing fast replacement of all occurrences!
Solution 2: Utilizing Regular Expressions π§
Regular expressions, or regex, provides a powerful toolset for manipulating strings. We can utilize the replace()
method in JavaScript along with regex to achieve our goal. Check out this example:
const originalString = "Hello world!";
const replacedString = originalString.replace(/o/g, "*");
console.log(replacedString);
In this snippet, we make use of the /g
flag in the regex to replace all occurrences of the character "o" with "*". Easy, peasy!
Time to Take Action: Engage and Share Your Wisdom! π‘π£
Now that you've discovered the need for speed when it comes to replacing characters in a string, it's time to take action. Here's a call-to-action challenge for you:
Challenge: Implement both solutions in your project and conduct some performance testing. Share your findings with our community! π
Engage: Leave a comment below and let us know your thoughts! Have you encountered any other unique situations where character replacement posed a challenge? We'd love to hear your experiences and solutions.
Remember, knowledge shared is knowledge gained! Let's come together and level up our JavaScript skills in the quest for optimized code. π
Stay tuned for more tech tips, tricks, and hacks. Until next time! πβ¨