Extract hostname name from string
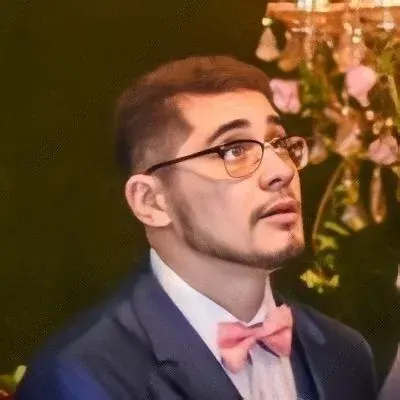
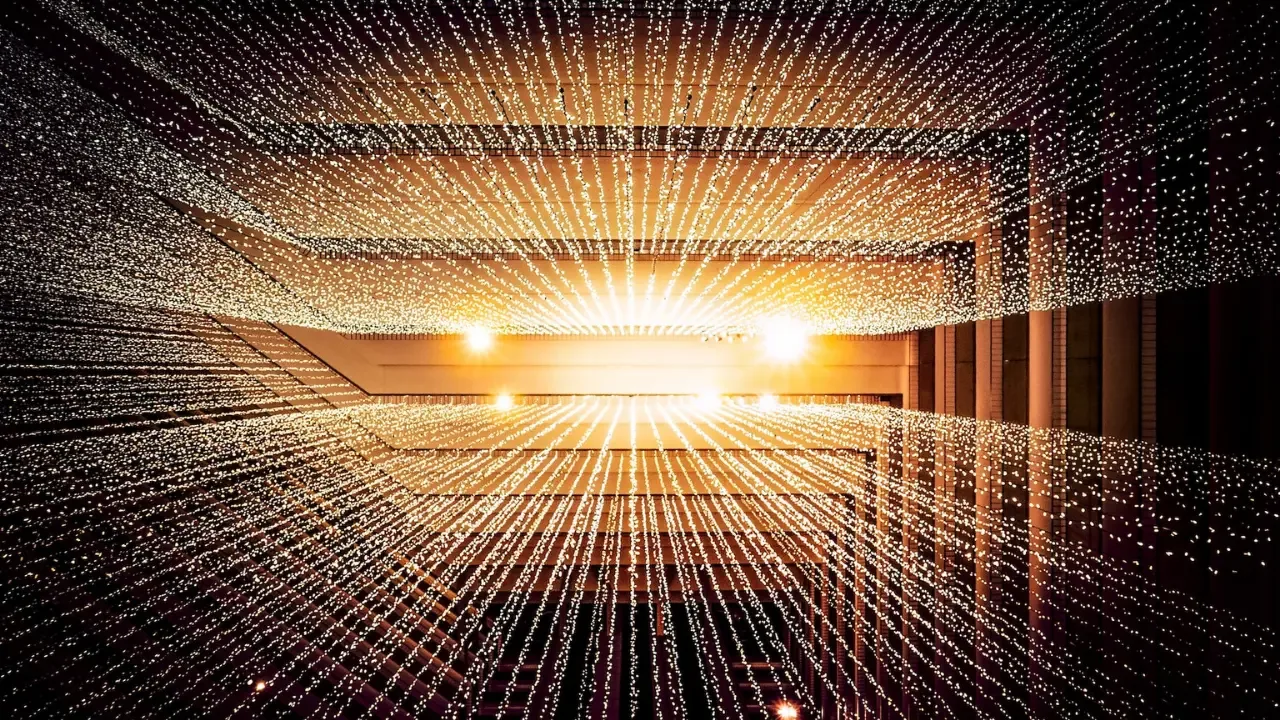
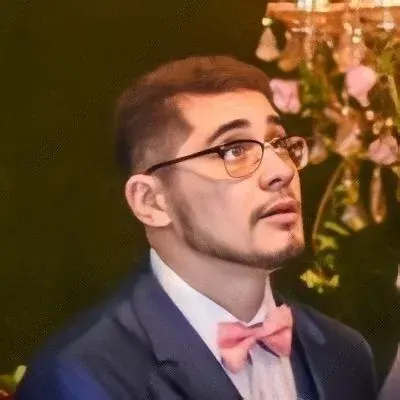
Extracting Hostname from a String: A Guide to Simplify the Process! 🏰🔎💪
Have you ever had a scenario where you needed to extract hostnames from a string, but found it to be a daunting task? Well, fear not, because in this blog post, we will address common issues, provide easy solutions, and help you extract those elusive hostnames effortlessly! 🎯
The Challenge 🤔
Let's dive right into the challenge at hand. Imagine having a text string that contains various URLs and their respective hostnames. You want to extract only the root of the URL, specifically the "www.example.com" or "example.com" domain, without removing any other parts of the string. Here are a few examples of what the string might look like:
http://www.youtube.com/watch?v=ClkQA2Lb_iE
http://youtu.be/ClkQA2Lb_iE
http://www.example.com/12xy45
http://example.com/random
Your goal is to extract the last two instances of hostnames (www.example.com
or example.com
) from the given string. However, there's a catch – you want to accomplish this task using JavaScript/jQuery, and if possible, without resorting to regular expressions (regex). Let's make it more efficient and snappy! 💨
The Solution: JavaScript + String Manipulation 👨💻⚙️
To begin with, let's look at a solution that avoids using regex and instead leverages JavaScript string manipulation techniques.
function extractHostnamesFromString(inputString) {
// Split the input string into an array of separate lines
const lines = inputString.split("\n");
// Iterate over the lines in reverse order
let foundHostnames = [];
for (let i = lines.length - 1; i >= 0; i--) {
const line = lines[i];
// Extract the hostname using substring and indexOf
const startIndex = line.indexOf("://") + 3;
const endIndex = line.indexOf("/", startIndex);
const hostname = line.substring(startIndex, endIndex);
// Check if the extracted hostname matches the desired format
if (hostname.includes("example.com")) {
foundHostnames.push(hostname);
// Break the loop when we have found the last two hostnames
if (foundHostnames.length === 2) {
break;
}
}
}
// Reverse the order of found hostnames to match the original order in the string
const finalHostnames = foundHostnames.reverse();
// Return the extracted hostnames as an array
return finalHostnames;
}
// Example usage
const input = `
http://www.youtube.com/watch?v=ClkQA2Lb_iE
http://youtu.be/ClkQA2Lb_iE
http://www.example.com/12xy45
http://example.com/random
`;
const extractedHostnames = extractHostnamesFromString(input);
console.log(extractedHostnames); // Output: ["www.example.com", "example.com"]
Let's break down this solution step by step:
We start by splitting the inputString into an array of separate lines using the
split("\n")
function. This allows us to iterate over each line individually.Next, we iterate over the lines in reverse order, starting from the last line and moving upwards. This ensures that we collect the last two hostnames.
Within each iteration, we use substring and indexOf to extract the relevant part of the line as the hostname. We find the starting index after the "://" part, and the ending index before the first forward slash ("/").
Once we have the extracted hostname, we check if it matches the desired format (in this case, containing "example.com"). If it does, we add it to the
foundHostnames
array and break the loop when we have collected the last two hostnames.Finally, we reverse the order of the
foundHostnames
array to match the original order in the string. This step is necessary because we traversed the lines in reverse order.The extracted hostnames are then returned as an array.
Embrace Simplicity and Boost Efficiency! 🚀
By following the above solution, you can easily extract hostnames from a string without relying on regex. JavaScript's string manipulation capabilities pave the way for a more efficient implementation, leaving regex for other tasks.
Are you ready to give it a try? Simply copy and paste the solution into your JavaScript/jQuery project, and adapt it to suit your specific needs. Extract those hostnames like a pro! 💪
If you have any other tech-related questions or need further assistance, feel free to reach out in the comments section below. Let's conquer these challenges together! 🙌
Have a great time extracting hostnames! Happy coding! ✨💻🔍