Expected validator to return Promise or Observable
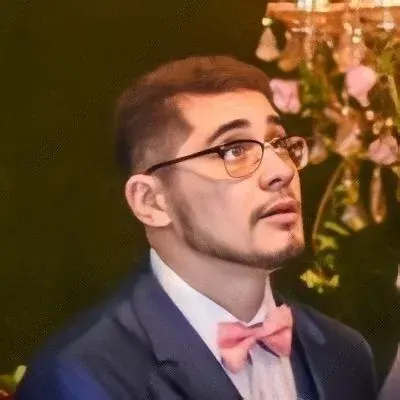
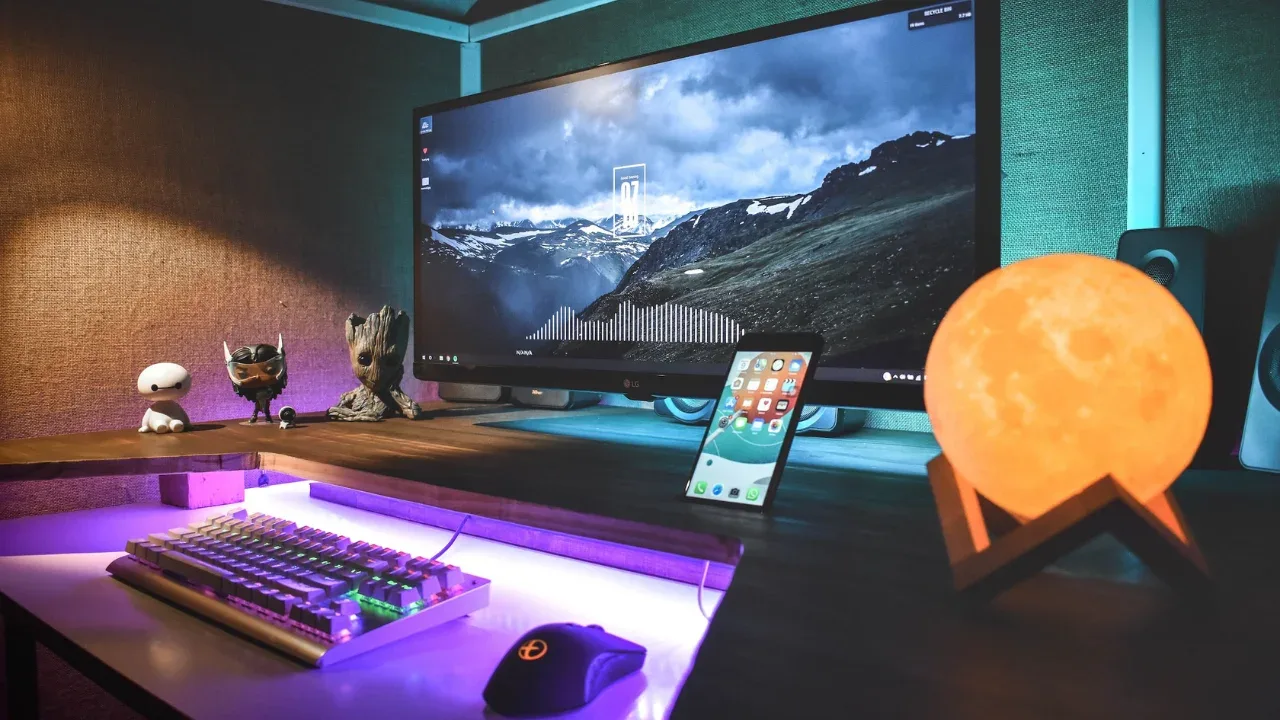
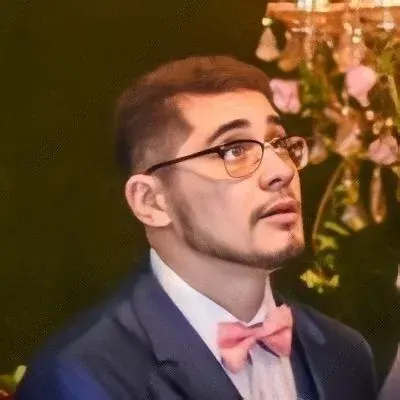
🚧 Expected validator to return Promise or Observable: A Common Issue in Angular 5 Custom Validation 🚧
So, you're trying to implement a custom validation in Angular 5 and stumbled upon the dreaded:
Expected validator to return Promise or Observable.
Fear not, my tech-savvy friend! I've got your back. In this blog post, I'll address this common issue, provide easy solutions, and even throw in a cool call-to-action at the end. Let's dive in!
Understanding the Problem
The error message is pretty straightforward. Angular expects your custom validator function to return either a Promise or an Observable, but it seems like your validation function isn't currently doing that. So let's fix it!
The Code Breakdown
First, let's take a look at the code you shared:
// Component Code
constructor(fb: FormBuilder, private cadastroService:CadastroService) {
this.signUp = fb.group({
"name": ["", Validators.compose([Validators.required, Validators.minLength(2)])],
"email": ["", Validators.compose([Validators.required, Validators.email])],
"phone": ["", Validators.compose([Validators.required, Validators.minLength(5)])],
"cpf": ["", Validators.required, ValidateCpf]
})
}
// Validation Function
import { AbstractControl } from '@angular/forms';
export function ValidateCpf(control: AbstractControl){
if (control.value == 13445) {
return {errorCpf: true}
}
return null;
}
Now that we have a better understanding of the code, let's see how we can solve this issue!
Easy Solution: Returning a Synchronous Value
To fix the "Expected validator to return Promise or Observable" error, we need to modify your custom validation function to return a synchronous value (null for valid or an error object for invalid).
To do this, we can simply wrap the error object in a synchronous Promise and return it. Here's how you can modify your ValidateCpf
function:
export function ValidateCpf(control: AbstractControl) {
if (control.value === 13445) {
return Promise.resolve({ errorCpf: true });
}
return null;
}
By using Promise.resolve()
, we create a synchronous Promise and return it. This satisfies Angular's requirement for a Promise or Observable.
Going Beyond Synchronous Validation
While the above solution works perfectly for synchronous validation, there may be scenarios where you need to perform asynchronous validation, such as making an HTTP request or querying a database.
In those cases, you would need to return a Promise or Observable directly from your custom validator function. Here's an example:
export function ValidateAsync(control: AbstractControl) {
return new Promise((resolve, reject) => {
// Simulating an asynchronous operation here
setTimeout(() => {
if (control.value === 12345) {
resolve({ errorAsync: true });
} else {
resolve(null);
}
}, 2000); // Delay of 2 seconds
});
}
In this example, we wrap our asynchronous code inside a Promise and handle the resolve and reject logic accordingly. You can adapt this code to handle your specific scenario.
Wrapping Up
And there you have it! We've tackled the "Expected validator to return Promise or Observable" issue by understanding the problem, providing an easy solution for synchronous validation, and even going beyond with an example of asynchronous validation.
I hope this blog post has helped you overcome this hurdle in your Angular 5 custom validation journey. If you have any questions or need further assistance, feel free to leave a comment below. Happy coding! 🎉
😎 Let's Keep the Conversation Going! 😎
Did this guide solve your problem? Have any additional tips or tricks to share? I'd love to hear from you! Leave a comment below and let's keep the conversation flowing. Together, we can make the tech world a better, bug-free place! 💻💪
[Insert cool and engaging call-to-action here]
Remember to share this blog post with your tech-savvy friends who are struggling with Angular 5 custom validation. Sharing is caring! 💌
Stay tuned for more exciting tech tips and solutions on my blog. Until next time, happy coding! 💻🚀