Execute a command line binary with Node.js
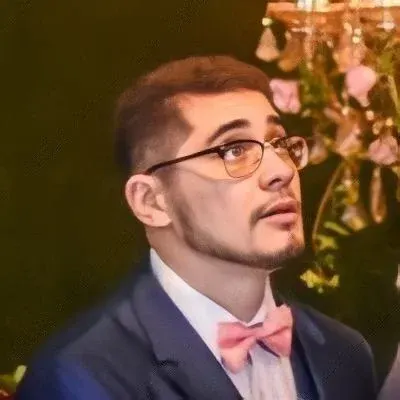
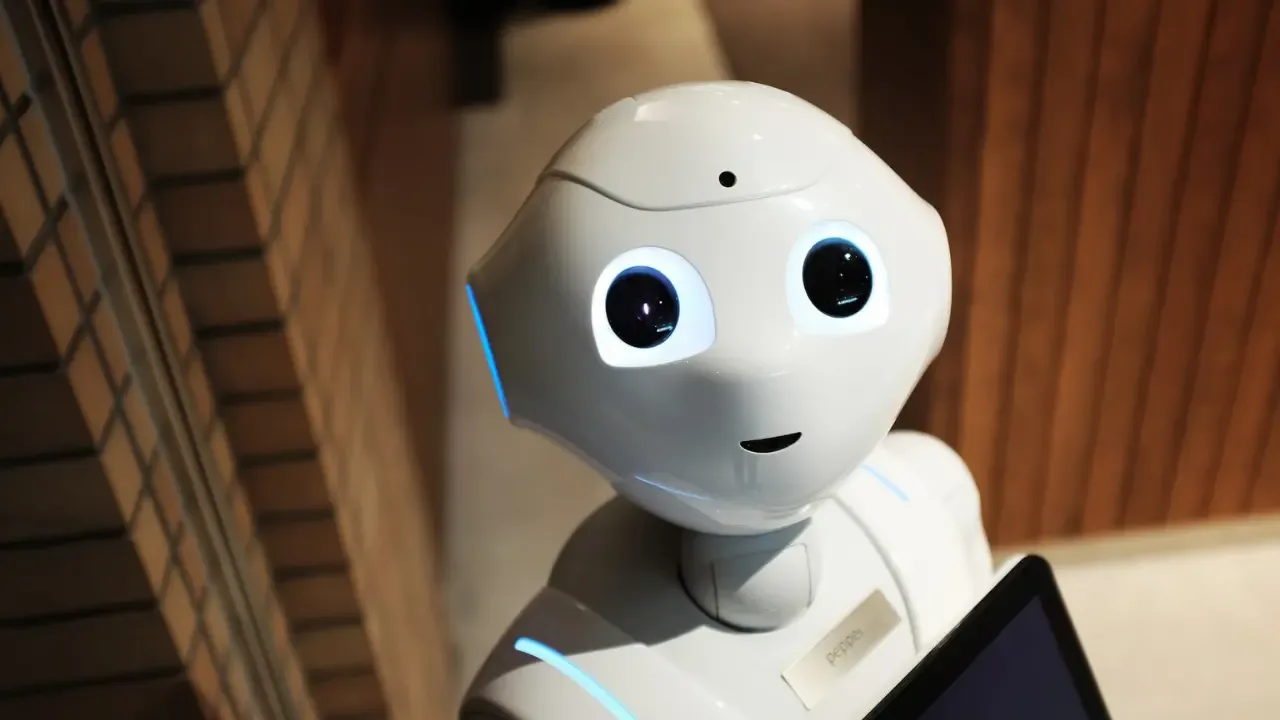
🔥 Executing Command Line Binaries with Node.js: A Beginner's Guide 🔥
Are you in the process of porting a CLI library from Ruby to Node.js? Facing the challenge of executing third-party binaries in your Node code? Fear not! We've got you covered with easy solutions and cool code snippets to accomplish this task seamlessly.
💡 Common Issue: Executing a Binary in Node.js Executing a command line binary in Node.js can be confusing, especially if you are new to the Node environment. However, with the right approach, it can be just as simple and straightforward as in Ruby.
🔌 Solution 1: Using the 'child_process' Module Node.js provides the 'child_process' module, which allows you to run shell commands and execute binaries. Here's an example of how to convert a file to PDF using the 'PrinceXML' binary:
const { exec } = require('child_process');
exec('prince -v builds/pdf/book.html -o builds/pdf/book.pdf', (error, stdout, stderr) => {
if (error) {
console.error(`Error: ${error.message}`);
return;
}
if (stderr) {
console.error(`STDERR: ${stderr}`);
return;
}
console.log(`PDF conversion successful!`);
});
In the above code snippet, we import the 'exec' function from the 'child_process' module. We pass our command as a string to the 'exec' function and handle any potential errors or output.
🔌 Solution 2: Using 'execSync' for Synchronous Execution If you prefer synchronous execution, Node.js also provides the 'execSync' function in the 'child_process' module. Here's an example:
const { execSync } = require('child_process');
try {
execSync('prince -v builds/pdf/book.html -o builds/pdf/book.pdf');
console.log(`PDF conversion successful!`);
} catch (error) {
console.error(`Error: ${error.message}`);
}
In this code snippet, we use 'execSync' to execute the binary synchronously. If an error occurs, we catch it and log an error message.
💪 Take Action: Try it Out! Now that you have the tools and code snippets in your arsenal, it's time to give it a spin! Open your Node.js project, replace the command with your desired binary, and run the code. Test it out and see the magic happen!
🌟 Engage with Us! We hope this guide has helped you overcome the hurdle of executing command line binaries with Node.js. If you have any further questions or need assistance, feel free to drop a comment below or reach out to our community. Keep coding and exploring the vast world of Node.js!
💌 Share this Guide! If you found this guide helpful, don't keep it to yourself! Share it with your tech-savvy friends, colleagues, or anyone facing similar challenges. Let's help each other rock Node.js development! 💪🚀
Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
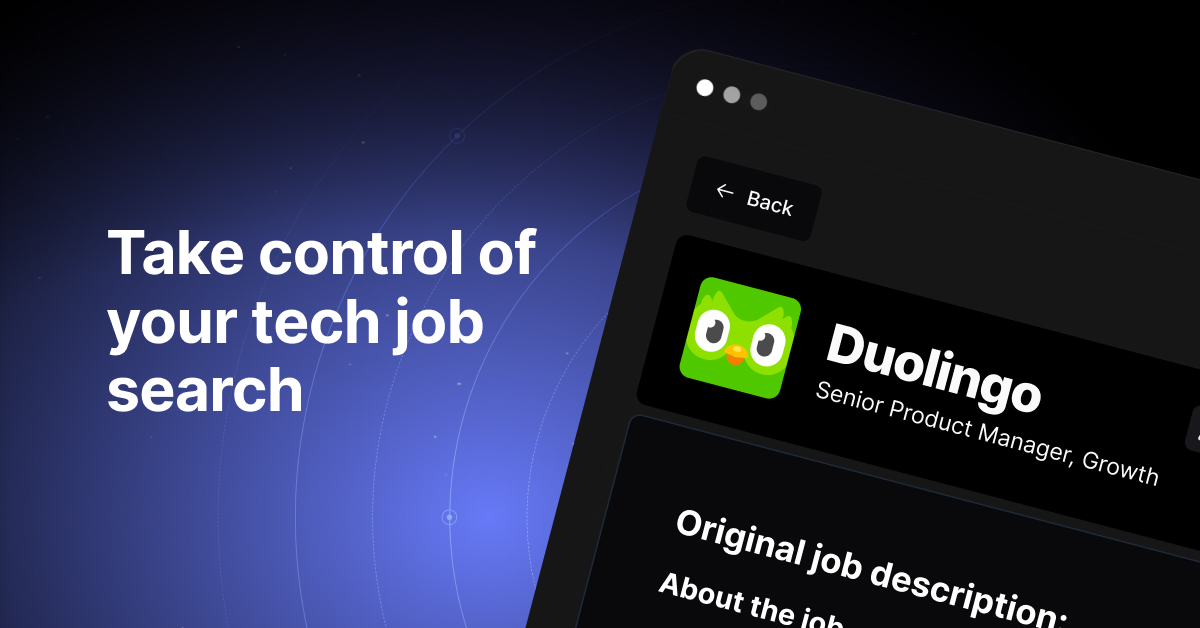