event.returnValue is deprecated. Please use the standard event.preventDefault() instead
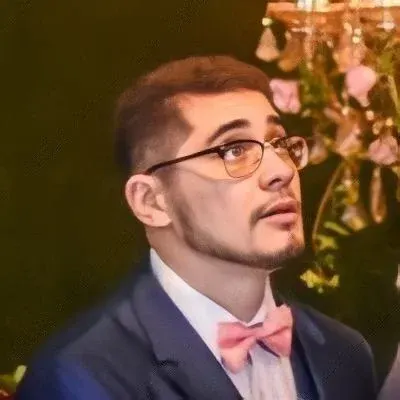
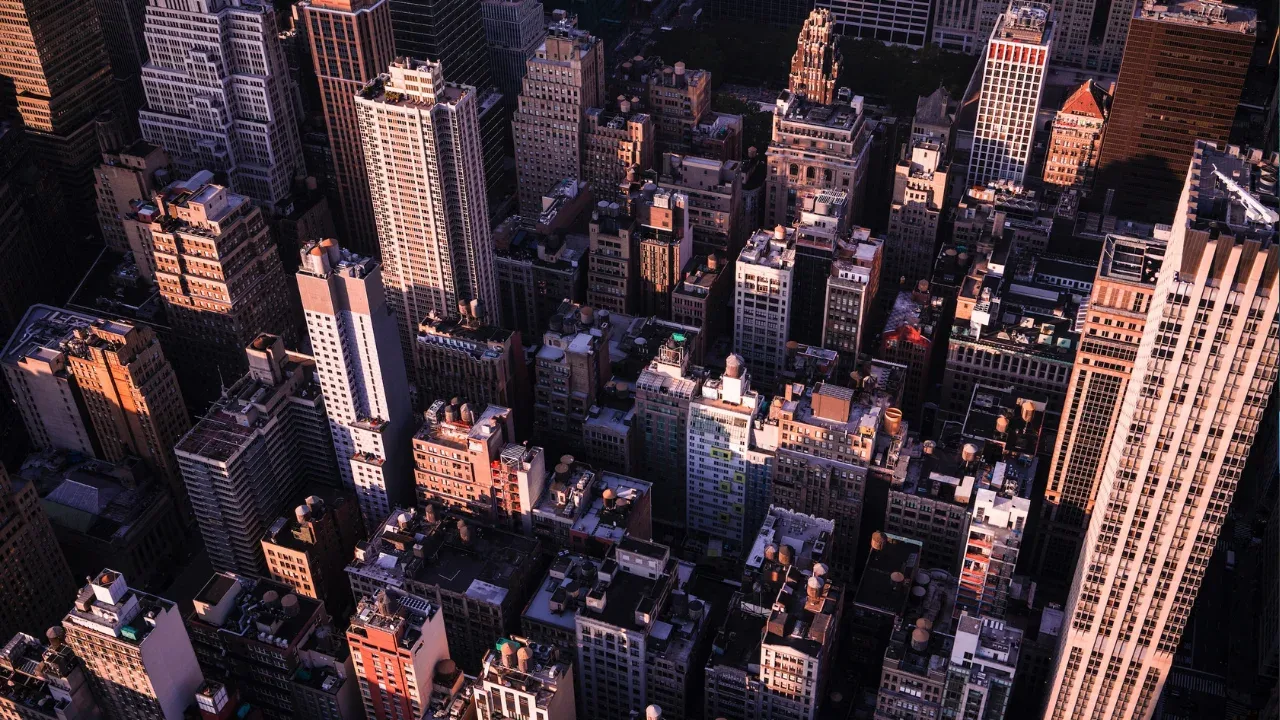
The Dreaded event.returnValue Error: Why event.preventDefault() is the Solution π ββοΈ
Are you experiencing the following error in your Google Chrome console? π±
event.returnValue is deprecated. Please use the standard event.preventDefault() instead.
If so, don't worry! You're not alone. Many developers encounter this error when using older versions of jQuery and relying on the deprecated event.returnValue
property. But fear not, I'm here to guide you through this issue and provide easy solutions! Let's dive in. πββοΈ
Understanding the Problem π
The error message you received is a result of using an outdated method to prevent the default action of an event. In modern versions of jQuery, such as 1.10.2 and above, event.returnValue
has been deprecated, meaning it's no longer recommended for use. Instead, the standard method to prevent the default action is event.preventDefault()
.
Analyzing the Code π¨βπ»
Let's take a closer look at the script provided:
<script>
$(document).ready(function () {
$("#changeResumeStatus").click(function () {
$.get("{% url 'main:changeResumeStatus' %}", function (data) {
if (data['message'] == 'hidden') {
$("#resumeStatus").text("ΡΠΊΡΡΡΠΎ");
} else {
$("#resumeStatus").text("ΠΎΠΏΡΠ±Π»ΠΈΠΊΠΎΠ²Π°Π½ΠΎ");
}
}, "json");
});
});
</script>
In this script, an event handler is attached to the element with the ID #changeResumeStatus
, specifically for the click
event. When this element is clicked, an AJAX request is made using $.get()
. Upon receiving the response, the content of the #resumeStatus
element is updated based on the value of data['message']
.
Solving the Issue β
To fix the error, we need to replace the usage of event.returnValue
with event.preventDefault()
in the event handler. Here's the updated script:
<script>
$(document).ready(function () {
$("#changeResumeStatus").click(function (event) {
event.preventDefault();
$.get("{% url 'main:changeResumeStatus' %}", function (data) {
if (data['message'] == 'hidden') {
$("#resumeStatus").text("ΡΠΊΡΡΡΠΎ");
} else {
$("#resumeStatus").text("ΠΎΠΏΡΠ±Π»ΠΈΠΊΠΎΠ²Π°Π½ΠΎ");
}
}, "json");
});
});
</script>
By adding the event
parameter to the click event handler and calling preventDefault()
, we ensure that the default action of the event is canceled. This will prevent the error message from appearing in the console.
Keep Your Code Future-Proof π
While replacing event.returnValue
with event.preventDefault()
solves the immediate issue, it's also worth considering upgrading to a more recent version of jQuery. Newer versions often provide improved performance, bug fixes, and additional features. By keeping your codebase up-to-date, you can avoid potential deprecation issues and take advantage of the latest advancements in jQuery.
Engage with the Community π€
I hope this guide helped you understand the issue and find a solution! If you have any further questions or insights, don't hesitate to leave a comment below. Let's grow and learn together! Happy coding! π»π
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
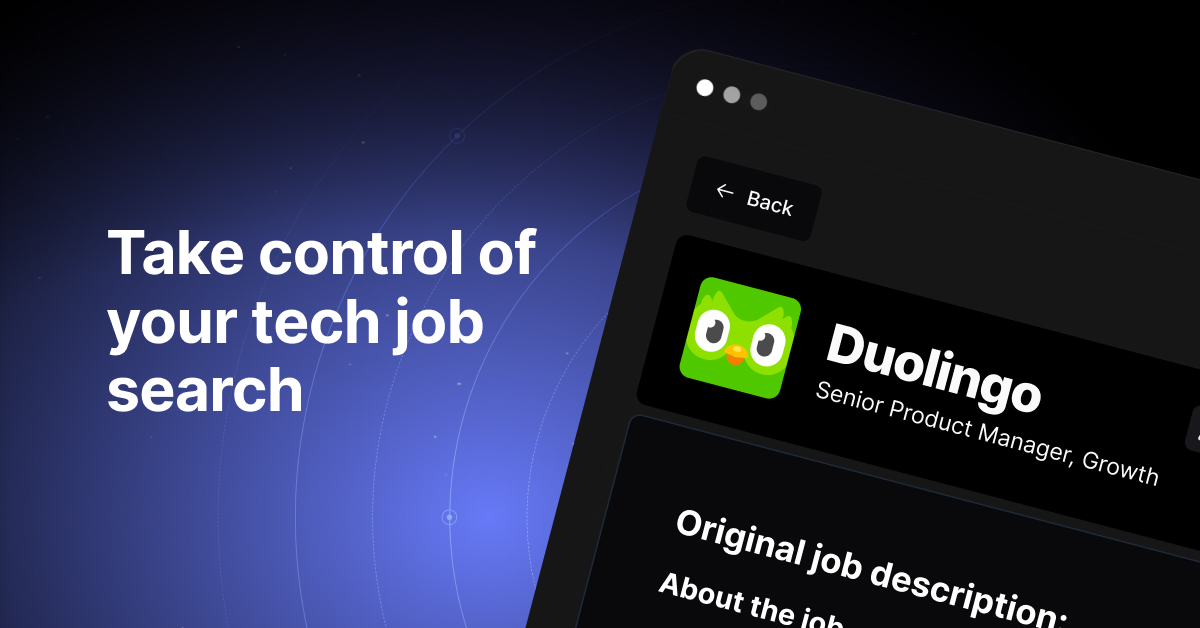