Event binding on dynamically created elements?
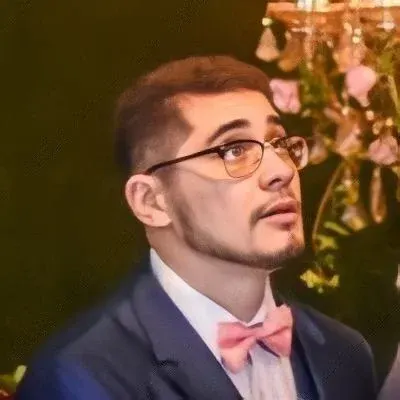
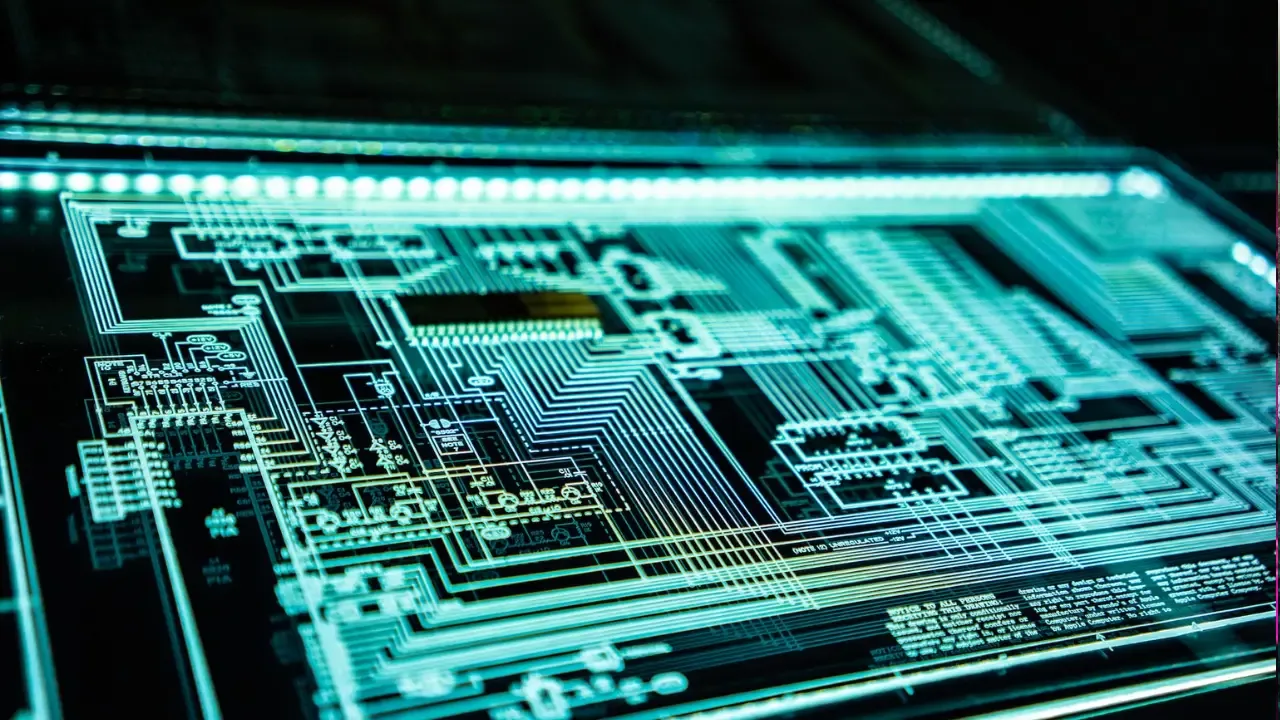
Dynamically creating and binding events to elements with jQuery
You're in the zone, twiddling with your code like a boss, until you come across an annoying problem. You've painstakingly looped through all the select boxes on your page and successfully bound a hover event to them, rocking that width change on mouse on/off. But, oh no! Any select boxes you add after the initial loop won't have the event bound. 😱
Don't worry, my friend! I've got your back. In this blog post, I'm going to show you how to dynamically create elements with jQuery, bind events to them, and solve the problem you're facing.
The problem:
After looping through the select boxes on your page and binding the hover event to them, any select boxes added via Ajax or DOM don't automatically have the event bound. This is because the event binding only happens on page ready, and any new elements introduced later don't receive the same treatment.
The solution:
Delegated event binding ✨: Instead of directly binding the event to each select box individually, we'll use event delegation. Event delegation allows us to bind the event to a parent element that exists on page ready and capture the event when it occurs on any of its child elements, regardless of when they were added.
jQuery's
on()
method: We'll make use of jQuery'son()
method, which allows us to attach event handlers to elements, including ones that are dynamically created. This method provides us the flexibility to handle events for existing and future elements in a single go. 🙌
Let's dive into some code to see how it works:
$(document).ready(function() {
// Bind hover event to parent element
$(document).on('mouseenter', 'select', function() {
// Handle mouse enter event
$(this).css('width', '200px');
}).on('mouseleave', 'select', function() {
// Handle mouse leave event
$(this).css('width', '150px');
});
});
In the code above, we're using the on()
method to bind two events, mouseenter
and mouseleave
, to the parent element document
. The second argument 'select'
is a selector that identifies the child elements we want to capture events for. This way, any select boxes, whether existing or added later, will trigger the hover event. 🎉
By using event delegation and jQuery's on()
method, you can ensure that dynamically created elements are also included in your event bindings.
Delegation got your back:
Remember, when it comes to delegation, choosing the most appropriate parent element is crucial. Select an element that will always be present in the DOM, ideally as close as possible to the dynamically created elements you want to target.
Your turn to shine ✨
Now that you know how to dynamically bind events to elements with jQuery, it's time to put your newly acquired knowledge into action and solve that problem you've been facing like a champ! Try implementing the code I provided and watch those dynamic elements magically respond to your hover events. 😎
Share your successes, troubles, or any cool tips you've discovered while solving this problem in the comments below. Let's help each other rock that dynamic event binding! 💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
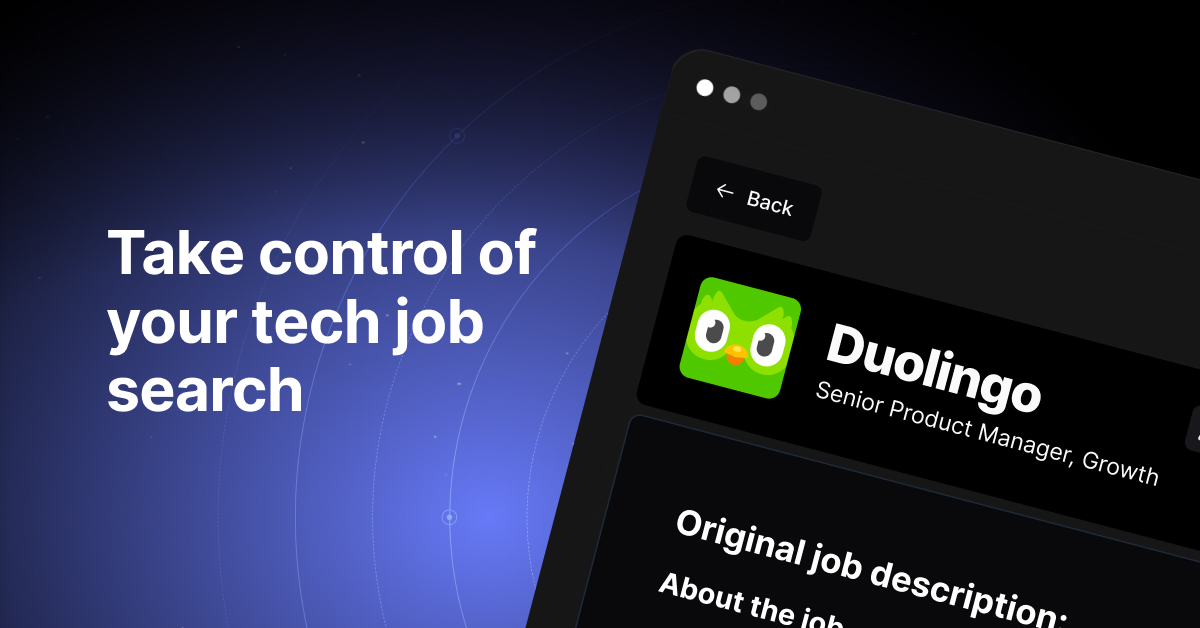