ESLint Parsing error: Unexpected token
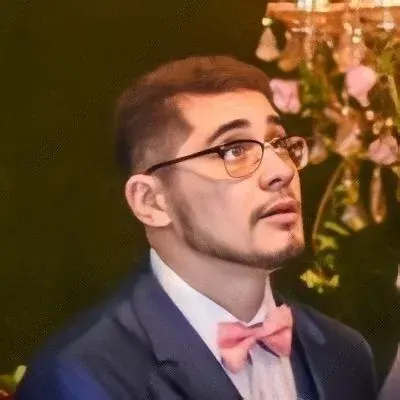
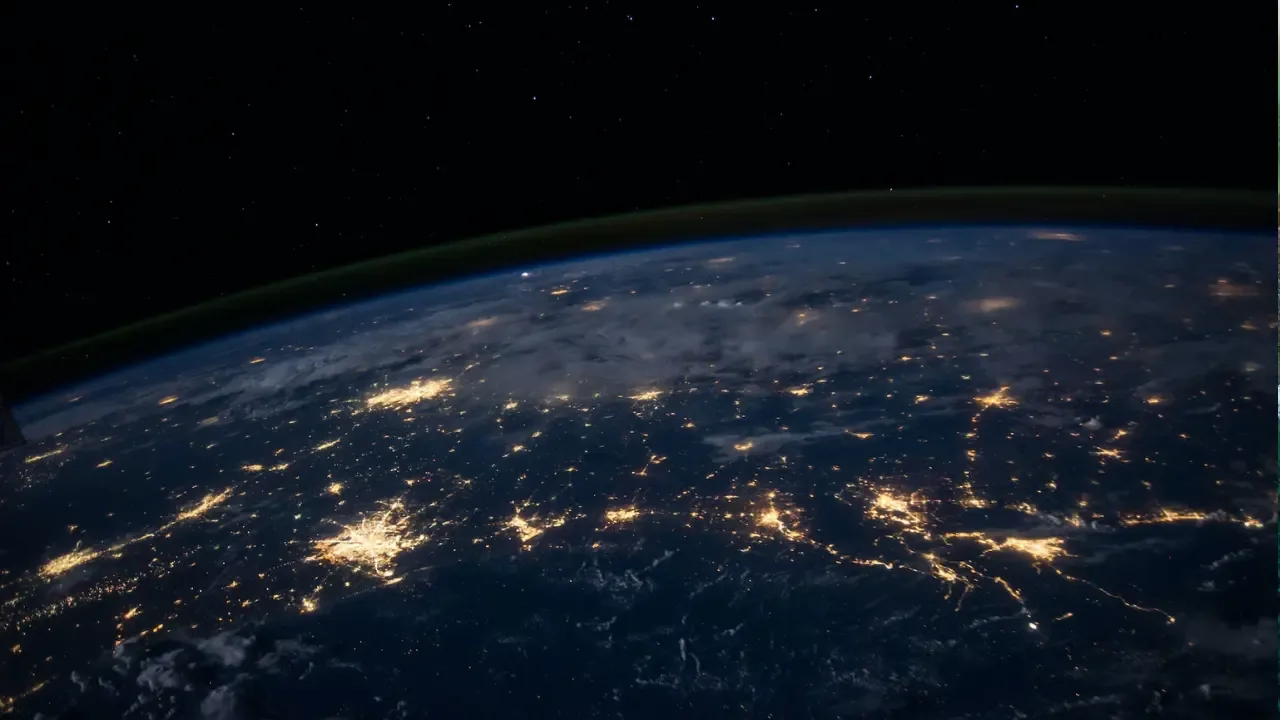
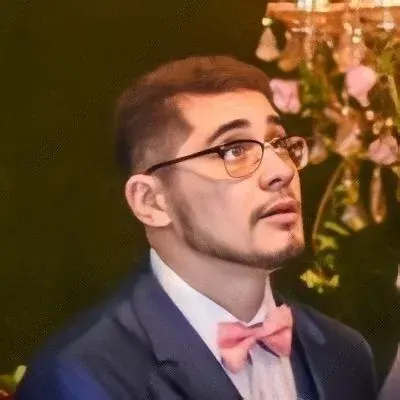
📝 ESLint Parsing error: Unexpected token
Hey there! If you're getting an unexpected token error while using ESLint, don't worry, I've got you covered! 👍
The error message you're seeing, "Parsing error: Unexpected token," usually occurs when the code contains a syntax error that ESLint is unable to parse. In your case, the issue is with the line:
const { Home, ...Components } = Pages;
The spread operator (...
) is causing the unexpected token error.
Now, let's take a closer look at your ESLint configuration. It seems like you're extending the "airbnb" ruleset, which is awesome! However, the "airbnb" ruleset might not support all the language features you're using.
To fix this issue, you have a few options:
1️⃣ Upgrade ESLint and babel-eslint: First, make sure you have the latest versions of ESLint and babel-eslint installed. Outdated versions can sometimes produce parsing errors.
2️⃣ Change the ESLint Parser: If you're already using the latest versions of ESLint and babel-eslint, you can try changing the parser used by ESLint. One popular option is @babel/eslint-parser
. To use it, install it as a dev dependency:
npm install --save-dev @babel/eslint-parser
Then, update your ESLint configuration to use @babel/eslint-parser
:
{
"parser": "@babel/eslint-parser",
"parserOptions": {
"requireConfigFile": false
}
}
3️⃣ Disable ESLint for specific line: If you don't want to change the parser or can't install additional dependencies, you can disable ESLint on the specific line causing the error. In your case, you can add the following comment above the line:
// eslint-disable-next-line
const { Home, ...Components } = Pages;
However, be cautious when disabling ESLint rules, as they serve as guidelines for maintaining code quality.
💡 Remember, these solutions should solve the "Parsing error: Unexpected token" issue. However, it's always a good idea to examine the overall code and ensure it adheres to best practices and the syntax rules of the language being used.
I hope these tips help you resolve the issue! If you have any further questions or need more assistance, feel free to ask. Happy coding! 😊
📣 Now, I want to hear from you! Have you ever encountered this error before? What was your solution? Share your experience in the comments below and let's help each other out!