Escaping HTML strings with jQuery
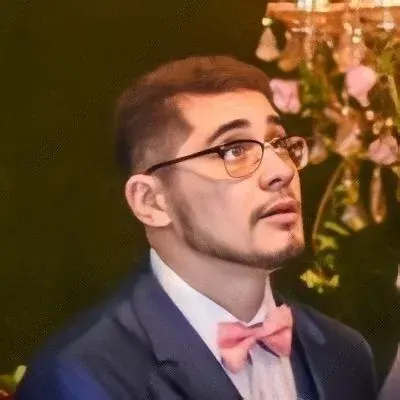
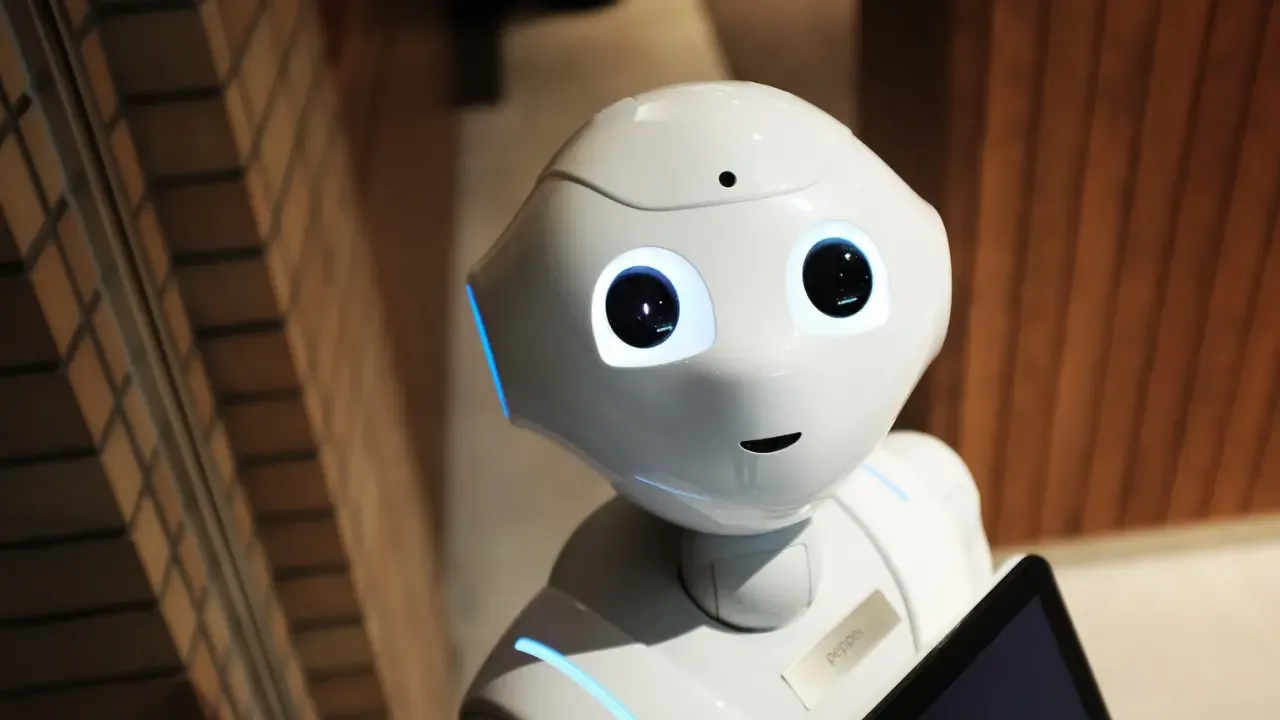
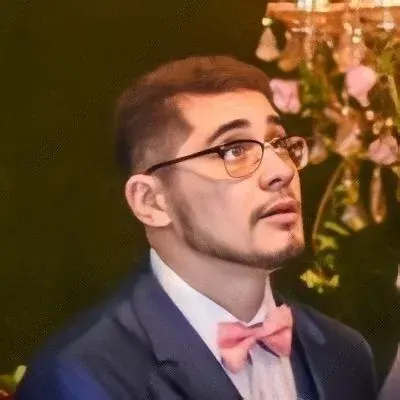
Escaping HTML Strings with jQuery: A Complete Guide 👾
Are you tired of your HTML strings causing havoc on your web page? 😫 HTML injection attacks can be a nightmare, but fear not! jQuery comes to the rescue with an easy way to escape HTML strings and keep your website safe and secure. 💪
In this blog post, we will address the common issue of escaping HTML strings using jQuery, provide you with simple solutions, and empower you to take control of your web development. Let's dive in! 🏊♀️
Understanding the Problem 🕵️♀️
Before we jump into the solutions, let's understand the problem at hand. The original question raised concerns about preventing JavaScript/HTML injection attacks when displaying arbitrary strings on an HTML page. Unescaped HTML strings can wreak havoc by executing malicious code or breaking your layout. 😱
By escaping HTML strings, we convert special characters into their corresponding entities, rendering them harmless to your web page. But how do we achieve this using jQuery? 🤔
Easy Solutions with jQuery 🚀
jQuery provides a handy method called .text()
that can be used to escape HTML strings effortlessly. This method ensures that any special characters in your string are converted to their safe counterparts. Let's see it in action! 🎬
var myString = '<p>Hello, <b>world</b>!</p>';
var escapedString = $('<div>').text(myString).html();
console.log(escapedString);
In the example above, we first create a temporary <div>
element using jQuery. Then, we set the text content of the div to our arbitrary string using the .text()
method. Finally, by accessing the .html()
of the div, we retrieve the escaped HTML string. Easy as pie! 🍰
You can now safely use escapedString
in your HTML without worrying about any unwanted side effects. Your web page will stay secure, and your users will enjoy a trouble-free experience. ✨
Level Up with Extensions 🔌
If you find yourself needing to escape HTML strings frequently, you might consider creating your own jQuery extension to simplify the process. This way, you can call your custom method whenever you need to escape a string. Let's take a look! 🧐
$.escapeHTML = function(string) {
return $('<div>').text(string).html();
};
var myString = '<p>Stay awesome, <i>world</i>!</p>';
var escapedString = $.escapeHTML(myString);
console.log(escapedString);
In the code snippet above, we define a $.escapeHTML()
function that utilizes the same technique we discussed earlier. By extending jQuery's functionality, we can now use $.escapeHTML()
to escape HTML strings across our entire project with ease. 🎉
Engage with us! 💬
Now that you have learned how to escape HTML strings using jQuery, it's time to put your newfound knowledge into action. Try implementing these techniques and let us know how it goes! Have any questions or want to share your own tips? Drop a comment below, and let's start a conversation! 💪🤩
Remember, securing your web pages should be a top priority, and with jQuery by your side, you can equip your development arsenal with the right tools. Keep coding, stay creative, and happy escaping! ✌️👩💻👨💻