Escape string for use in Javascript regex
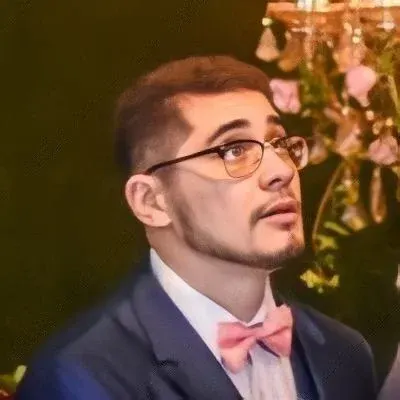
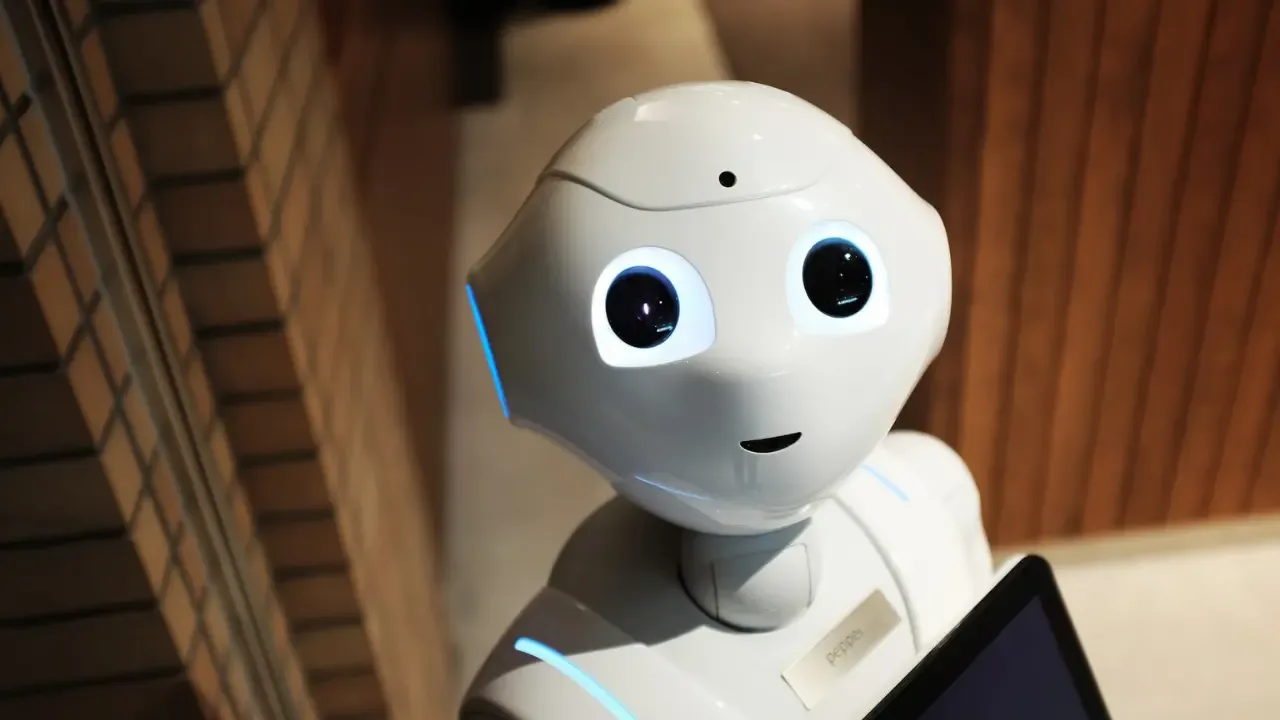
How to Escape Strings for Use in JavaScript Regex 🔒🔍
JavaScript regex can be a powerful tool for searching and manipulating strings, but it can also be tricky when it comes to dealing with special characters. In this guide, we'll tackle the common issue of escaping strings for use in JavaScript regex, providing easy solutions that will save you time and frustration. Let's dive in! 💪
Understanding the Problem 😰
When building a JavaScript regex based on user input, you may encounter issues when that input contains special characters. These special characters, such as ?
or *
, have special meanings in regex and can cause unexpected behavior or even render the regex invalid.
The Perfect Solution 💡
To correctly escape all special characters for use in JavaScript regex, you can make use of the RegExp.escape()
function. Unfortunately, JavaScript doesn't provide this function out of the box, but fret not! We can create our own version of it.
Creating a RegExp.escape() Function ✨
To escape special characters in JavaScript regex, you can define the RegExp.escape()
function using the following code snippet:
RegExp.escape = function (string) {
return string.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
};
This function takes a string as an argument and returns the escaped version of that string.
Implementing the Solution ✔️
Now that we have our RegExp.escape()
function in place, we can use it to escape user input for a JavaScript regex. Here's an updated version of the FindString()
function that incorporates the escaping:
function FindString(input) {
var escapedInput = RegExp.escape(input);
var reg = new RegExp(escapedInput);
// [snip] perform search
}
By calling RegExp.escape(input)
, we escape all special characters in the user input before using it to build our regex. This ensures that the regex will work correctly and produce the expected results, regardless of any special characters in the input.
Let's Put it into Action! 🚀
To illustrate how the RegExp.escape()
function works, let's take a look at an example:
var userInput = 'How much is 2+2?';
var escapedInput = RegExp.escape(userInput);
console.log(escapedInput); // Output: "How much is 2\\+2\\?"
As you can see, the ?
and +
characters in the user input have been correctly escaped with a backslash \
.
Engage with Our Community! 💬
Did you find this blog post helpful? Have you used the RegExp.escape()
function before? We'd love to hear your experiences and any additional tips you might have. Leave us a comment below and let's start a conversation! 😄
Conclusion 🎉
Escaping strings for use in JavaScript regex doesn't have to be a daunting task. By using the RegExp.escape()
function, we can easily handle special characters in user input and ensure our regex works as intended. Incorporate this practice into your coding workflow, and you'll be regex-ing like a pro in no time! Happy coding! 🌟✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
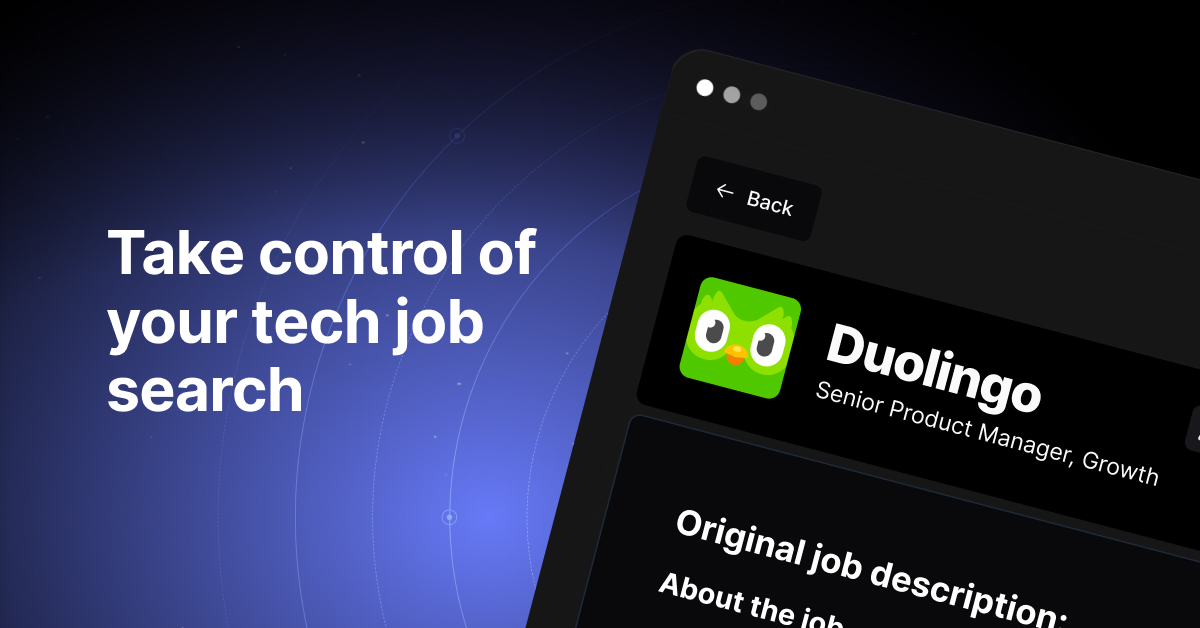