ES6 Map in Typescript
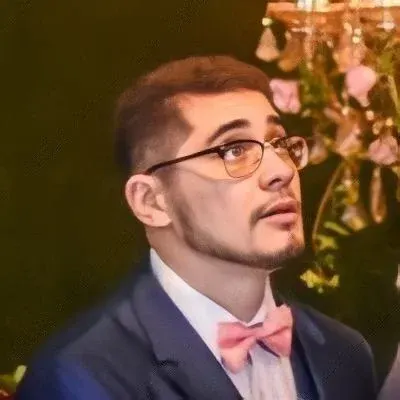
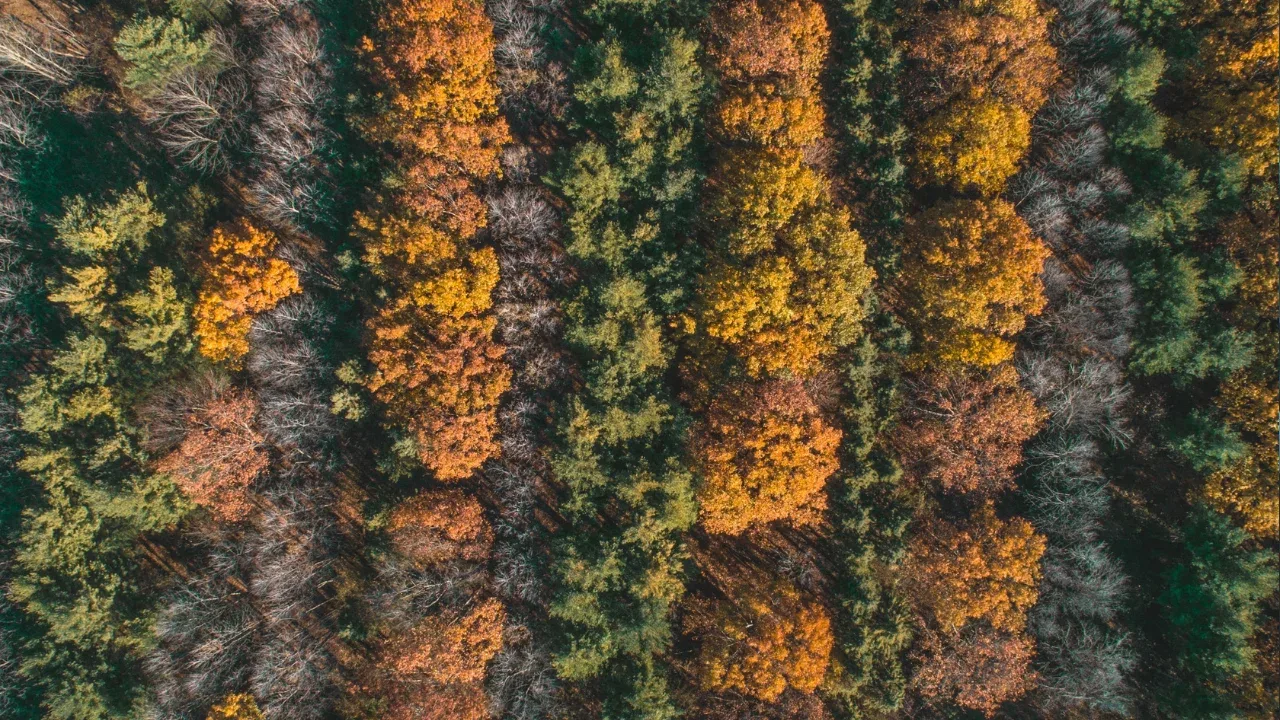
🎉✍🔥 Title: Demystifying ES6 Map in TypeScript: A Killer Combo for High-performance Data Management
Hey there TypeScript enthusiasts! 👋 Are you struggling to figure out how to declare an ES6 Map type in TypeScript? Fear not, because we've got you covered! In this blog post, we'll break down the common issues around using ES6 Maps in TypeScript, provide easy-to-implement solutions, and leave you with a compelling call-to-action. Let's dive in! 💪
So, you want to create a class in TypeScript with a property that utilizes the power of an ES6 Map. We've got the basics covered for you. Check out the code snippet below:
class Item {
configs: ????;
constructor () {
this.configs = new Map();
}
}
☝️ The question mark placeholders indicate that we're about to reveal the secret of declaring an ES6 Map type in TypeScript. Ready? Let's go! 🕵️♀️
Declaring an ES6 Map Type in TypeScript
To declare an ES6 Map type in TypeScript, you can utilize the Map
interface provided by TypeScript. It's super straightforward! Replace the question marks with the following code:
class Item {
configs: Map<YOUR_KEY_TYPE, YOUR_VALUE_TYPE>;
constructor () {
this.configs = new Map();
}
}
Replace YOUR_KEY_TYPE
with the type you want to use as the key in the Map, and YOUR_VALUE_TYPE
with the type you want to use as the corresponding value. For example, if you want to store string keys and number values, use string
and number
as the types respectively. Simple, right? 😎
Common Pitfalls and Easy Solutions
🚧 Pitfall: Missing Type Declarations
In some cases, you might encounter TypeScript errors because the required type declarations are missing for the keys or values used in the Map. This can lead to confusion and frustration. But don't worry! We've got an easy solution for you.
🛠️ Solution: Ensure that you have accurately declared the types of your Map keys and values. Double-check if you have imported any necessary libraries to provide the type declarations, or define custom types if needed. Remember, TypeScript thrives on the power of explicit typing! 😉
🚧 Pitfall: Incompatible Type Assignments
Another stumbling block you might face is assigning incompatible types to your Map. TypeScript's strict type-checking can be your best friend or your worst enemy in such situations.
🛠️ Solution: Double-check the types you are using when assigning values to your Map. Ensure that the assigned values match the defined value type in your Map declaration. TypeScript won't let you sneak in any surprises, but with a little attention to detail, you'll be on the right track in no time! 🚀
Your Coding Journey Continues!
Now that you've mastered the art of declaring an ES6 Map type in TypeScript and conquered the common issues, it's time to put your skills to the test and explore the endless possibilities that await you! 🎉
We encourage you to experiment, dive deeper into TypeScript's features, and harness the power of ES6 Maps to efficiently manage your data. Let your creativity soar, and never stop learning! 🌟
Share your thoughts, experiences, and any additional tips in the comments section below. We'd love to hear from you! Let's embark on this coding adventure together! 🌈
💡 Pro Tip: Don't forget to check out the official TypeScript documentation for more detailed examples and in-depth insights!
That's a wrap, fellow developers! You're now equipped with the knowledge you need to confidently declare and leverage ES6 Maps in TypeScript. Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
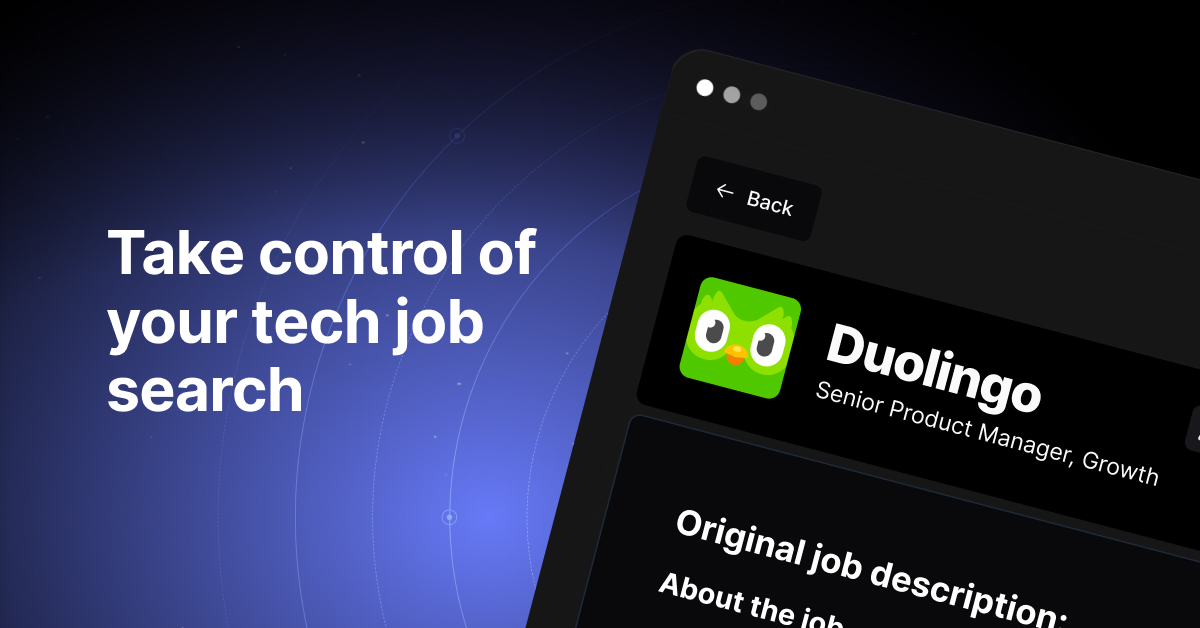