Dynamic tag name in React JSX
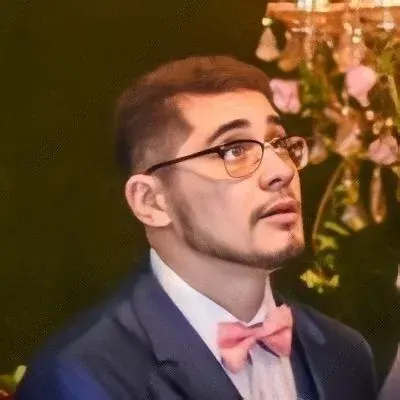
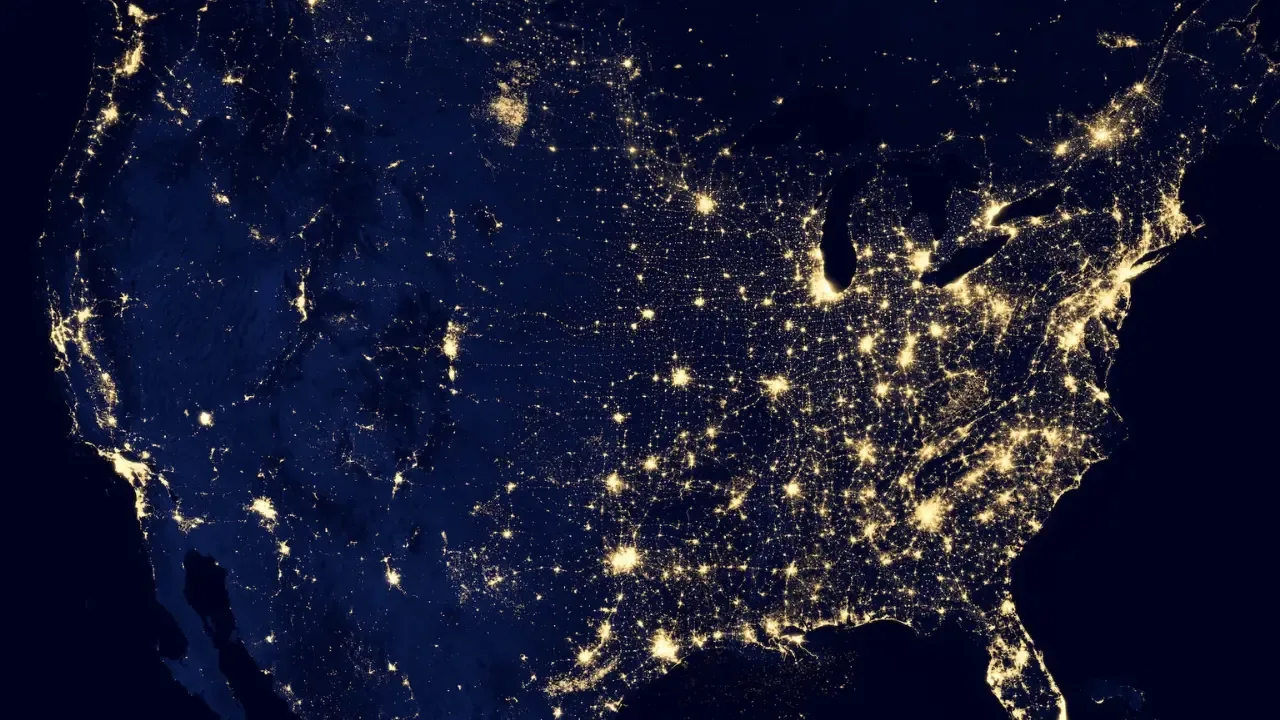
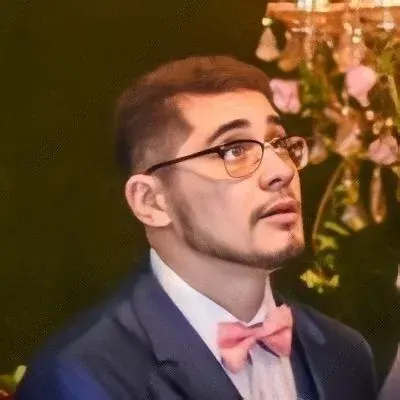
🌟 Dynamic Tag Name in React JSX: A Guide
Are you tired of hardcoding your React component's HTML heading tags? 😫 Do you want to dynamically set the heading level based on a prop? 🏗️ Look no further, my friend! In this blog post, I'll show you how to solve this common issue 🤓 and empower you to level up your React game! 🚀
The Problem 🤔
The challenge lies in dynamically setting the tag name for HTML headings in a React component. Traditionally, you might try something like this 👇:
<h{this.props.level}>Hello</h{this.props.level}>
And you may expect to see the output 👇:
<h1>Hello</h1>
But alas, this approach does not work as expected! 😢
The Solution 💡
Fear not, for there's a simple solution to achieve the desired dynamic heading tag in React JSX! 🎉
To overcome this obstacle, you can utilize the React.createElement()
function, which allows you to create custom React elements with dynamic tag names. Here's how it looks in action 👇:
React.createElement(`h${this.props.level}`, null, 'Hello')
This line of code dynamically generates the desired HTML heading tag using the level
prop. Voila! 😎
Implementation Example 🚀
Now, let's put theory into practice! Suppose you have a Header
component that accepts a level
prop to determine the heading's level. Here's an example implementation to give you a clear picture 👇:
import React from 'react';
const Header = (props) => {
const Tag = `h${props.level}`;
return React.createElement(Tag, null, 'Hello');
};
export default Header;
In this implementation, we create a variable Tag
that dynamically holds the appropriate HTML heading tag based on the received level
prop. We then use React.createElement()
to generate the desired heading tag. Easy-peasy, right? 😉✨
Your Turn! 🚀📢
Now that you've learned this awesome trick, it's time to put it to use! Try implementing a React component that dynamically changes the heading tag based on user input or any other condition. Get creative! 🎉
Share your implementation in the comments below and let's engage in a vibrant discussion! 🔥💬 Don't forget to spread the word and share this blog post to help other React enthusiasts tackle this issue effortlessly. Together, we can level up our React skills! 🌟
Happy coding! 😄💻