Download a file by jQuery.Ajax
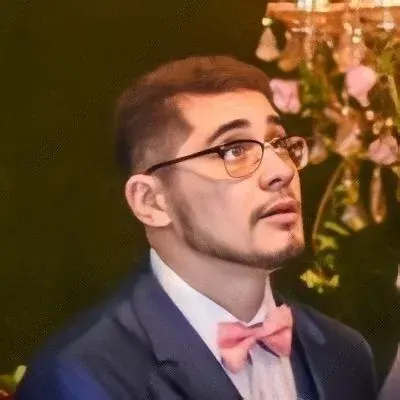
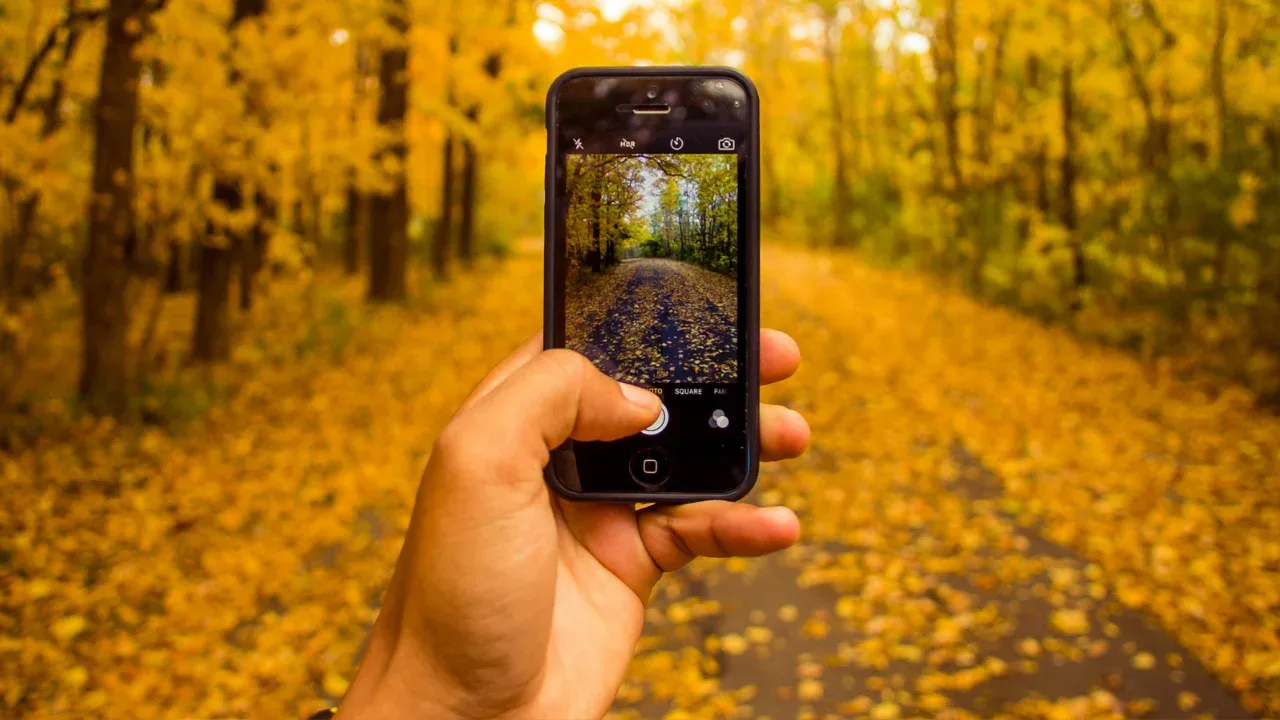
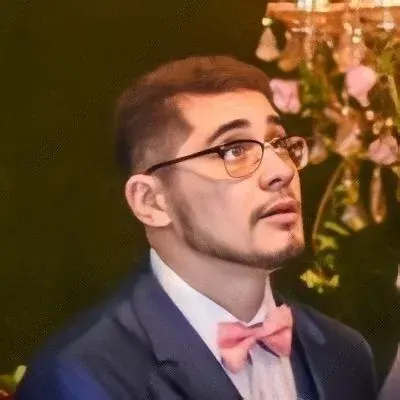
Download a File Using jQuery.Ajax: Easy Solutions for Common Issues
π Welcome to our tech blog, where we're all about finding easy solutions to common coding problems! Today, we'll tackle the question of how to download a file using jQuery.Ajax. So, if you've been struggling with opening that file downloading window, stick around and we'll guide you through it! πͺ
The Struts2 Action Setup
To give you some context, let's first look at the server-side configuration for file downloading using Struts2. In your Struts2 action, you have the following setup:
<action name="download" class="com.xxx.DownAction">
<result name="success" type="stream">
<param name="contentType">text/plain</param>
<param name="inputName">imageStream</param>
<param name="contentDisposition">attachment;filename={fileName}</param>
<param name="bufferSize">1024</param>
</result>
</action>
This configuration ensures that the file is streamed and sent back as a response when the download
action is called.
The jQuery.Ajax Dilemma
Now, here's where the problem arises. When you try to call the Struts2 action using jQuery.Ajax, you encounter an issue. Let's take a look at the code snippet again:
$.post(
"/download.action",{
para1:value1,
para2:value2
....
},function(data){
console.info(data);
}
);
In this case, when you inspect the response in Firebug, you'll notice that the data retrieved is seen as a "Binary stream." So, how do you open that file downloading windowβποΈ you know, the one that allows users to save the file locally?
The Solution: Response Handling with jQuery.Ajax
Thankfully, we have an easy solution to this problem. To trigger the file downloading window, you need to handle the response in a specific way.
Instead of using the regular $.post
function, you can use the $.ajax
function to have more control over the response. Here's an updated code snippet that solves the issue:
$.ajax({
url: '/download.action',
method: 'POST',
data: {
para1: value1,
para2: value2
....
},
xhrFields: {
responseType: 'blob' // Set the response type to blob
},
success: function(data) {
const downloadUrl = URL.createObjectURL(data); // Create a temporary URL for the downloaded file
const link = document.createElement('a');
link.href = downloadUrl;
link.download = 'file_name.ext'; // Set the desired file name and extension
link.click(); // Trigger the click event to open the file downloading window
URL.revokeObjectURL(downloadUrl); // Clean up the temporary URL
}
});
This modified code snippet uses $.ajax
instead of $.post
and sets the responseType
property to 'blob'
in the xhrFields
option. This ensures that the response is treated as a blob, which contains the file data.
Furthermore, we create a temporary URL for the downloaded file using URL.createObjectURL
and attach it to a dynamically created <a>
element. By setting the download
attribute of the <a>
element to the desired file name and extension, we ensure that the file is saved with the correct name when the user clicks on it.
Finally, we trigger the click event on the <a>
element to open the file downloading window, allowing users to save the file locally. Don't forget to clean up the temporary URL using URL.revokeObjectURL
to avoid memory leaks.
π£ Engage with Us!
And there you have it! With just a few tweaks to your jQuery.Ajax code, you can seamlessly download a file and provide a smooth user experience.
If you found this blog post helpful, be sure to share it with your fellow developers. Have any more questions or need assistance with a different coding dilemma? Drop a comment below or reach out to us on social media. We love hearing from you! π¬
Happy coding! ππ»