Does JavaScript have a method like "range()" to generate a range within the supplied bounds?
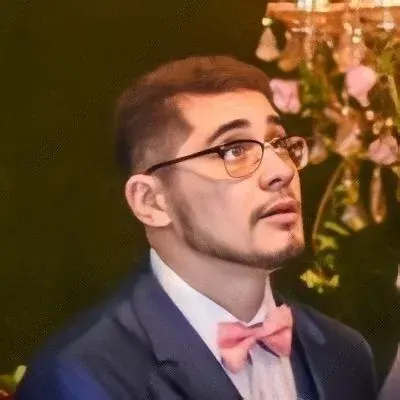
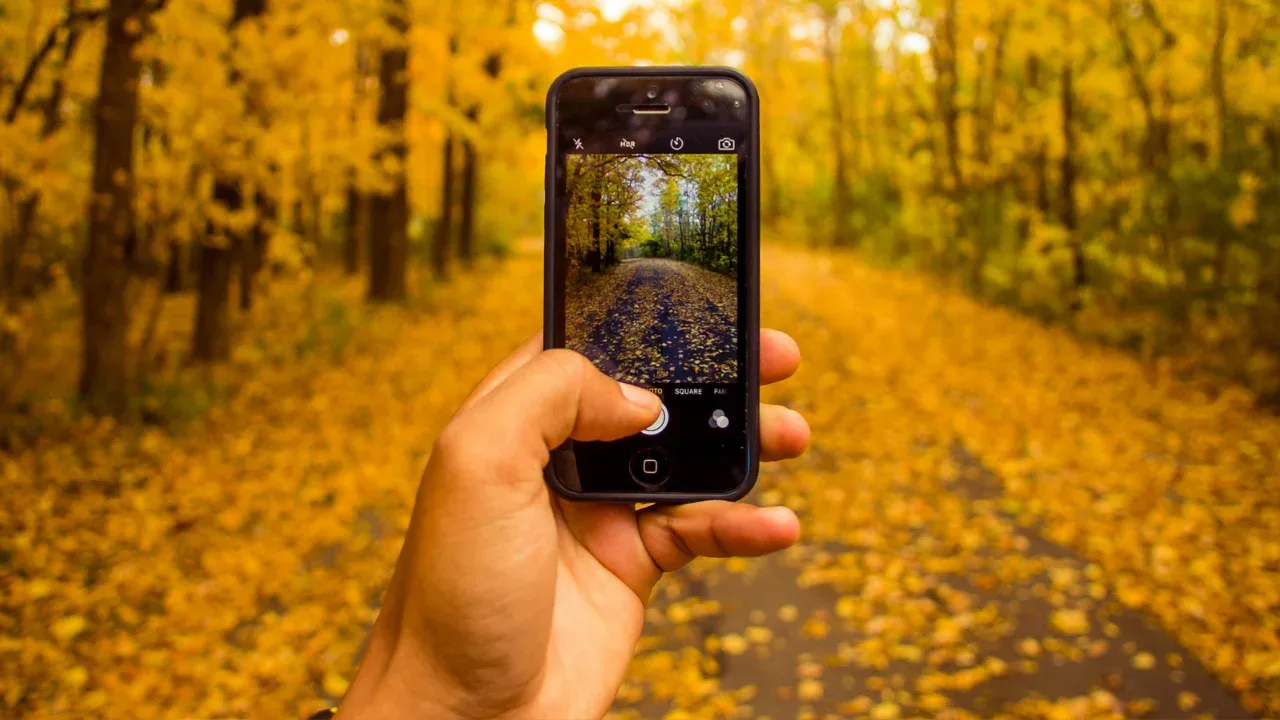
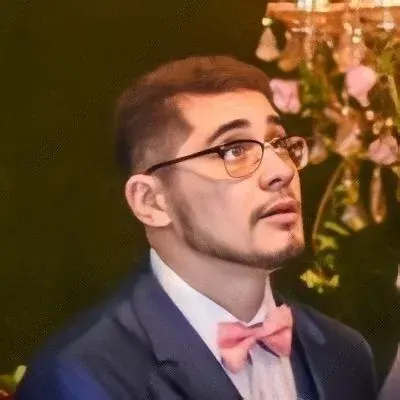
🧩 JavaScript: Generating Ranges with the range()
Method
If you've ever worked with PHP, you might be familiar with the handy range()
function that allows you to generate a range of numbers or characters. But what about JavaScript? Does it have a similar method to easily generate a range within the supplied bounds? 🤔
Well, the short answer is, JavaScript does not have a built-in range()
method like PHP. However, fear not! There are several easy solutions to achieve the same functionality. Let's dive in! 💪
🌟 Option 1: Using a for
loop
One straightforward approach is to utilize a for
loop to generate the desired range. Here's a sample code snippet:
function range(start, end) {
const result = [];
for (let i = start; i <= end; i++) {
result.push(i);
}
return result;
}
console.log(range(1, 3)); // [1, 2, 3]
console.log(range(10, 15)); // [10, 11, 12, 13, 14, 15]
In this example, the range()
function takes a starting and ending number and returns an array containing all the numbers within that range.
🌟 Option 2: Utilizing Array.from()
Another approach leverages the Array.from()
method, which creates a new, shallow-copied array from an array-like or iterable object. Here's how you can use it to generate a range:
function range(start, end) {
return Array.from({ length: end - start + 1 }, (_, index) => start + index);
}
console.log(range(1, 3)); // [1, 2, 3]
console.log(range(10, 15)); // [10, 11, 12, 13, 14, 15]
By specifying the length
property in the object passed to Array.from()
, we determine the size of the resulting array. Then, using the arrow function, we calculate and populate each element by adding the index to the starting value.
🌟 Option 3: ES6 Generator Function
For the more adventurous JavaScript developers among us, you can also create a generator function to produce the desired range on the fly. It's a bit more advanced but quite powerful! Take a look at this example:
function* range(start, end) {
for (let i = start; i <= end; i++) {
yield i;
}
}
const myRange = [...range(1, 3)];
console.log(myRange); // [1, 2, 3]
In this alternative solution, we define a generator function using the function*
syntax. By using the yield
keyword, we can generate and return each number in the range upon iteration.
🎉 Conclusion
While JavaScript doesn't have a built-in range()
method like PHP, there are several clever ways to generate ranges within the supplied bounds. Whether you prefer using a for
loop, Array.from()
, or even an ES6 generator function, you now have the tools to tackle this common problem in JavaScript! 🚀
So go ahead, give these methods a try, and start generating those ranges like a pro! If you have any other JavaScript-related questions or cool methods you'd like to share, drop a comment below and let's keep the conversation going! 👇😄