Difference between "process.stdout.write" and "console.log" in node.js?
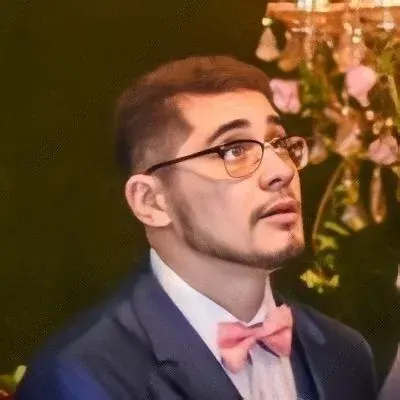
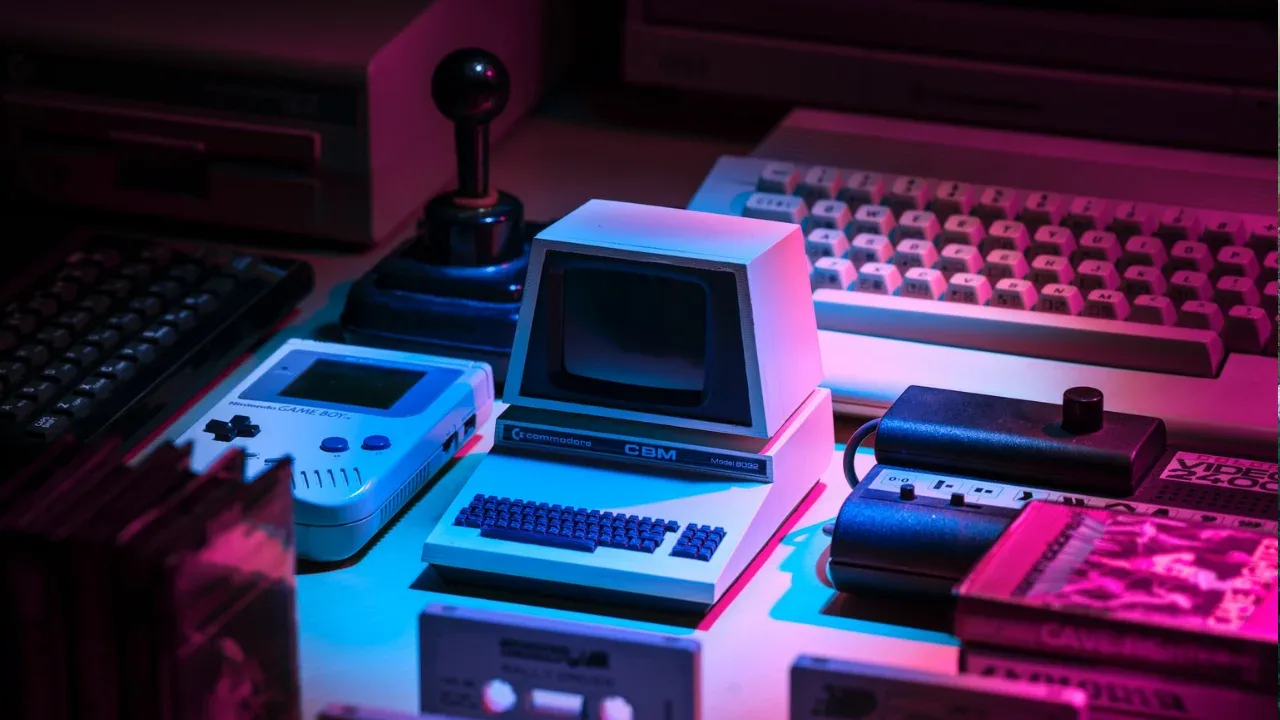
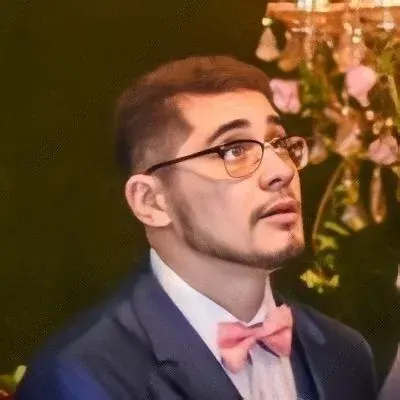
Understanding the Difference between "process.stdout.write" and "console.log" in Node.js
Have you ever used both process.stdout.write
and console.log
in your Node.js code and wondered why they produce different results? If you've encountered this issue, don't worry - you're not alone!
In this blog post, we'll dive deep into the distinction between process.stdout.write
and console.log
and provide easy solutions for common problems. By the end, you'll have a clear understanding of when to use each method, and you'll be able to write code that produces the desired output without any unexpected surprises! 🚀
Understanding the Basics
First, let's get familiar with process.stdout.write
and console.log
individually.
process.stdout.write
The process.stdout.write
method is part of the Node.js process
global object. It allows us to write data directly to the standard output (stdout). This method takes a string as an argument and writes it to the console without appending a newline character.
Here's an example:
process.stdout.write('Hello, World!');
The above code would output Hello, World!
without a newline character.
console.log
On the other hand, console.log
is a built-in function provided by Node.js. It prints data to the console, appending a newline character at the end.
Here's an example:
console.log('Hello, World!');
The above code would output Hello, World!
with a newline character.
The Difference Made Clear
Now that we understand the individual workings of process.stdout.write
and console.log
, let's explore the key difference between the two.
The crucial distinction lies in the way they handle different data types.
Strings
process.stdout.write
expects a string as its argument and prints it directly. It doesn't make any assumptions about the type of data provided.
On the other hand, console.log
accepts various data types, including strings, and automatically converts them to a human-readable format. This means that when you pass a string to console.log
, it will handle it as expected, printing it as a readable string.
Objects
Here's where things get interesting. If you pass an object as an argument to console.log
, it will automatically call the object's toString()
method to convert it to a string representation. This results in a nicely formatted output that is easy to read and understand.
However, process.stdout.write
behaves differently. It treats the object as a binary object and prints it in a raw, machine-readable format. This is why you observed unreadable characters when using console.log
for a variable that contained an object, while process.stdout.write
produced a clear object representation.
To illustrate this further, take a look at the following example:
const exampleObject = {
name: 'John Doe',
age: 30,
};
console.log(exampleObject);
The output will be a formatted, human-readable version of the object:
{ name: 'John Doe', age: 30 }
However, if you use process.stdout.write
instead:
process.stdout.write(exampleObject);
The output will be the raw, machine-readable representation of the object:
[object Object]
Solutions and Best Practices
If you're facing the issue of unreadable characters while using console.log
for objects, here are a few solutions:
Use
console.dir
: Instead of usingconsole.log
, you can useconsole.dir
to print an object's properties in a readable format. It is specifically designed for debugging objects.console.dir(exampleObject);
The output will be:
{ name: 'John Doe', age: 30 }
Convert the object to a string: If you need to print the object as a string using
process.stdout.write
, you can useJSON.stringify
to convert it into a string representation before writing it to the console.process.stdout.write(JSON.stringify(exampleObject));
The output will be:
{"name":"John Doe","age":30}
By following these solutions and best practices, you can ensure that your Node.js code produces the expected output, whether you choose to use process.stdout.write
or console.log
.
Join the Discussion!
We hope this blog post helped you understand the difference between process.stdout.write
and console.log
in Node.js. If you have any questions or comments, feel free to join the discussion below! We'd love to hear your experiences and insights on this topic.
Remember, understanding the nuances of different Node.js methods will elevate your coding skills and make you a more effective developer.
Happy coding! 😄👩💻👨💻
PS. Share this post with your fellow developers to help them tackle the "unreadable characters" issue in Node.js!