Difference between JSON.stringify and JSON.parse
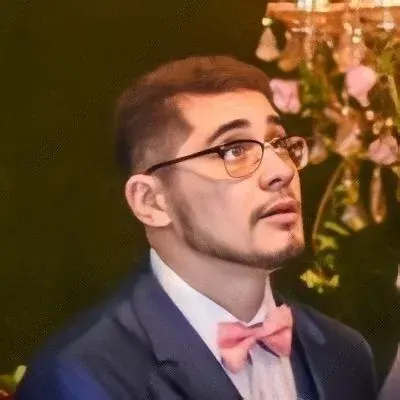
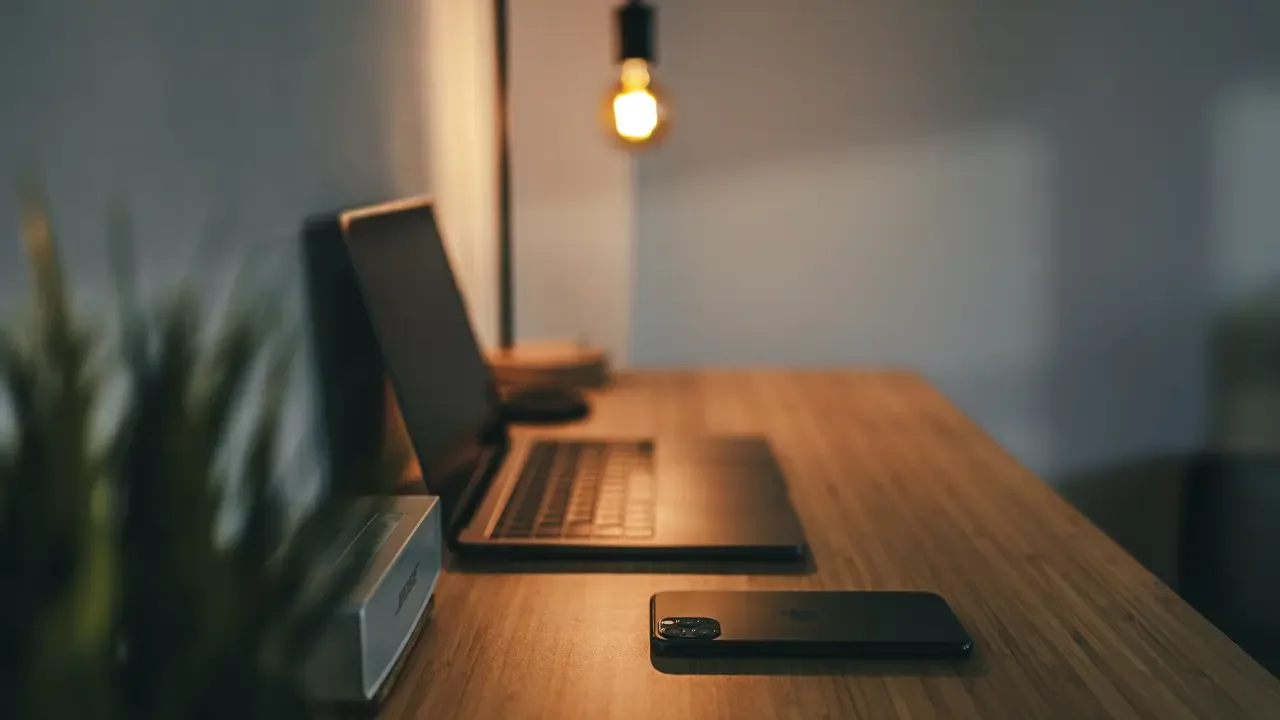
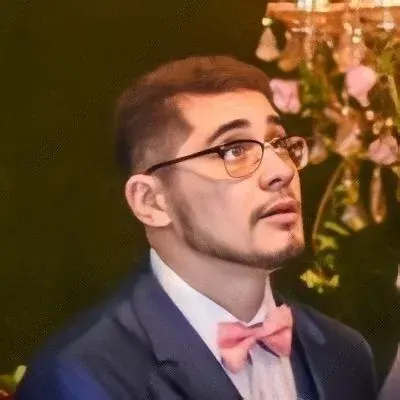
Understanding JSON.stringify and JSON.parse in JavaScript
Have you ever come across the situation where you send data via AJAX and when you try to parse it or stringify it, you get unexpected results? 😫 Don't worry, you are not alone! Many developers stumble upon this confusion. In this blog post, we are going to demystify the differences between JSON.stringify and JSON.parse methods in JavaScript, understand common issues, and provide easy solutions. Let's get started! 🚀
JSON.stringify: Converting JavaScript Objects to Strings 📝
JSON.stringify is a method in JavaScript that converts a JavaScript object into a JSON string. It takes an object as an argument and returns a string representation of that object. This method is commonly used when sending data to a server or storing data locally.
Let's take an example. Suppose we have an object called person
:
const person = {
name: "John Doe",
age: 25,
city: "New York"
};
If we use JSON.stringify on this object:
const jsonString = JSON.stringify(person);
console.log(jsonString); // Output: {"name":"John Doe","age":25,"city":"New York"}
As you can see, the object is converted into a string representation with key-value pairs separated by commas. This string can then be sent or stored easily.
JSON.parse: Converting JSON Strings to JavaScript Objects 🖥️
JSON.parse is the counterpart of JSON.stringify. This method takes a JSON-formatted string as an argument and converts it back into a JavaScript object. It's commonly used when receiving data from a server or retrieving stored data.
Let's continue with our previous example. If we have a JSON string:
const jsonString = '{"name":"John Doe","age":25,"city":"New York"}';
If we use JSON.parse on this string:
const person = JSON.parse(jsonString);
console.log(person); // Output: {name: "John Doe", age: 25, city: "New York"}
As you can see, the JSON string is converted back into a JavaScript object.
Common Issues and Solutions 🤔
Now, let's address the confusion mentioned in the context. When using console.log on a parsed JSON string, you might see [object, Object]
instead of the expected object representation. This happens because the console is trying to print the object as a string. To see the actual object, you can use console.dir
or console.table
methods:
console.dir(person); // Output: {name: "John Doe", age: 25, city: "New York"}
If you need to use the JavaScript object for further processing or manipulation, there is no need to worry. The parsed object is still intact, and you can work with it as usual.
Conclusion and Actionable Takeaways ✍️
Understanding the differences between JSON.stringify and JSON.parse is crucial when dealing with JSON data in JavaScript. Remember the following key points:
JSON.stringify converts a JavaScript object into a JSON string.
JSON.parse converts a JSON string back into a JavaScript object.
When using JSON.parse, the console might display
[object, Object]
, but the parsed object is still valid.
The next time you encounter issues while parsing or stringifying JSON data, refer back to this guide.
If you found this blog post helpful, share it with your fellow developers! 😃 And if you have any questions or additional insights, leave a comment below. Let's continue the conversation!
Happy coding! 💻✨