Difference between == and === in JavaScript
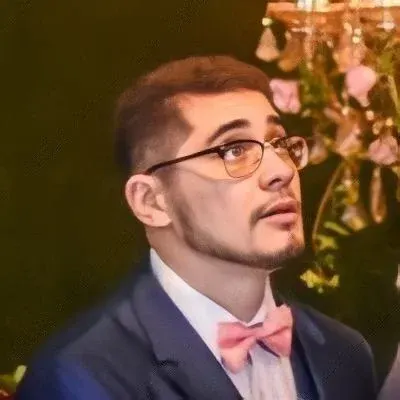
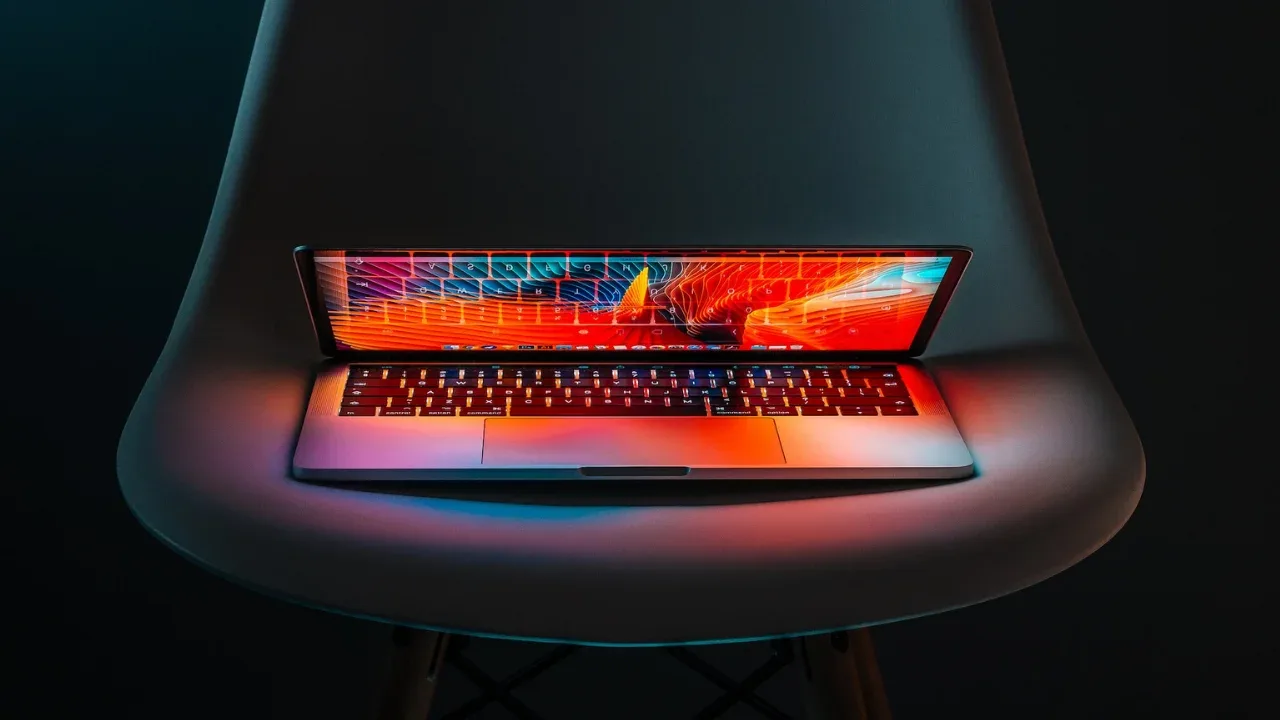
Understanding the Difference between == and === in JavaScript 🤔
JavaScript, the language powering the web, provides various operators that allow us to compare values. Among these, ==
and ===
are commonly used. But what exactly is the difference between these two operators? 🤷♂️ Let's delve into it!
The Basics: Equality Operators 👥
First, let's get familiar with the basic equality operators in JavaScript:
==
(double equals): Evaluates if two values are equal without considering their variable types.===
(triple equals): Evaluates if two values are strictly equal, taking into account their variable types as well.
The Sneaky == Operator 🕵️♀️
The ==
operator can sometimes lead to unexpected outcomes due to a concept called type coercion. Let me explain with an example:
console.log(5 == "5"); // true 🤔
Wait, what? Shouldn't "5"
(a string) and 5
(a number) be considered different? Not quite! JavaScript implicitly converts one of the values to match the other during the comparison process.
In this case, JavaScript converts the string "5"
to a number before evaluating equality. So, the statement 5 == "5"
becomes 5 == 5
, which obviously evaluates to true
. 😯
The Reliable === Operator 🛡️
Unlike ==
, the ===
operator performs a strict comparison. It not only checks for the value, but also for the type of the operands. Let's see an example:
console.log(5 === "5"); // false 😎
Here, JavaScript doesn't perform any type coercion since it requires both values to have the same type. The number 5
and the string "5"
are considered different types, so the strict comparison 5 === "5"
evaluates to false
as expected. 🙌
Solving Common Issues: 🚀
1. Be Consistent with Your Comparisons 🔁
It's generally recommended to use the ===
operator for comparisons since it provides more accuracy and avoids unexpected results caused by type coercion. By using strict comparisons consistently, you can prevent potential bugs in your code.
2. Use Type Conversion Explicitly when Required 🔄
If you need to compare values of different types, it's better to convert them explicitly before using the equality operators. This makes your intentions clear and avoids confusion. For instance:
console.log(5 === Number("5")); // true 😇
In this example, we convert the string "5"
into a number explicitly using the Number()
function, allowing us to perform a reliable strict comparison.
The Implementation: Battle-Tested Operators 💪
In addition to ==
and ===
, JavaScript provides two more equality operators:
!=
(not equals): Evaluates if two values are not equal without considering their variable types.!==
(strict not equals): Evaluates if two values are not strictly equal, considering both their values and types.
These operators work in a similar manner as their equality counterparts, but with negated results.
Your Turn! 📢
Now that you have a good understanding of the difference between ==
and ===
in JavaScript, it's time to put your knowledge to the test! 🚀
Think of a scenario where you can utilize strict comparisons (===
) for a specific task and share it in the comments below. Let's discuss and learn from each other! 💬
Remember, choosing the right operator can make a big difference in ensuring the accuracy and reliability of your JavaScript code. 😊
Happy Coding! 💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
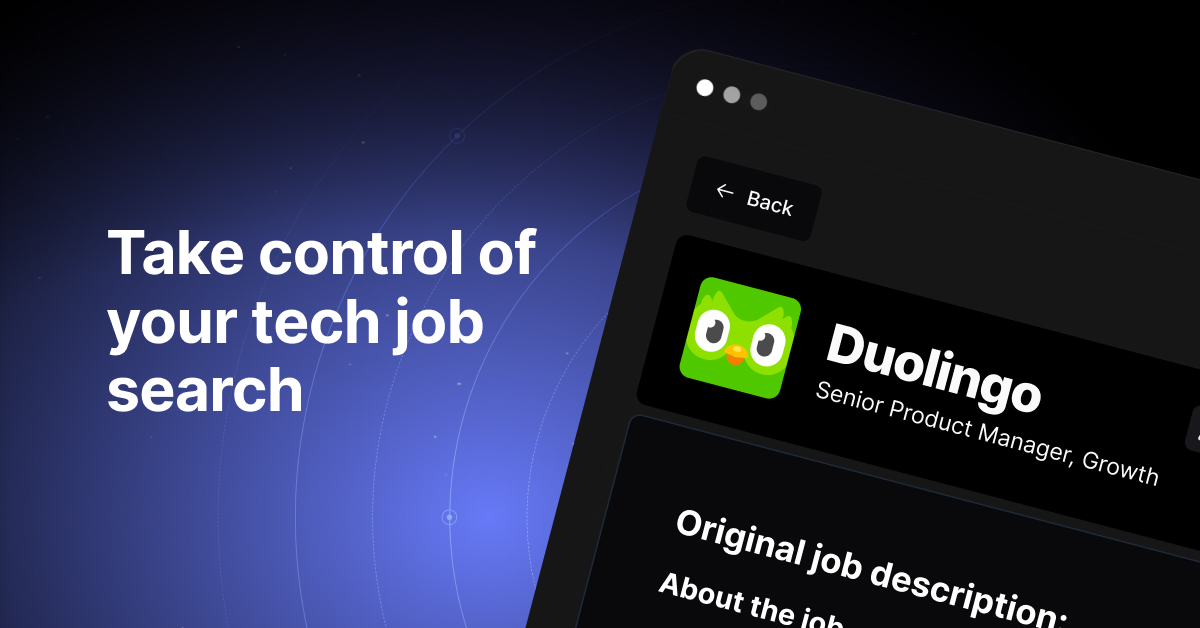