Determine if ajax call failed due to insecure response or connection refused
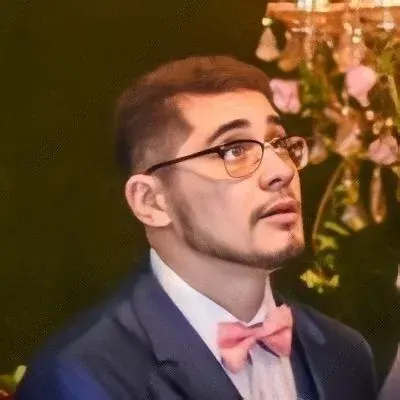
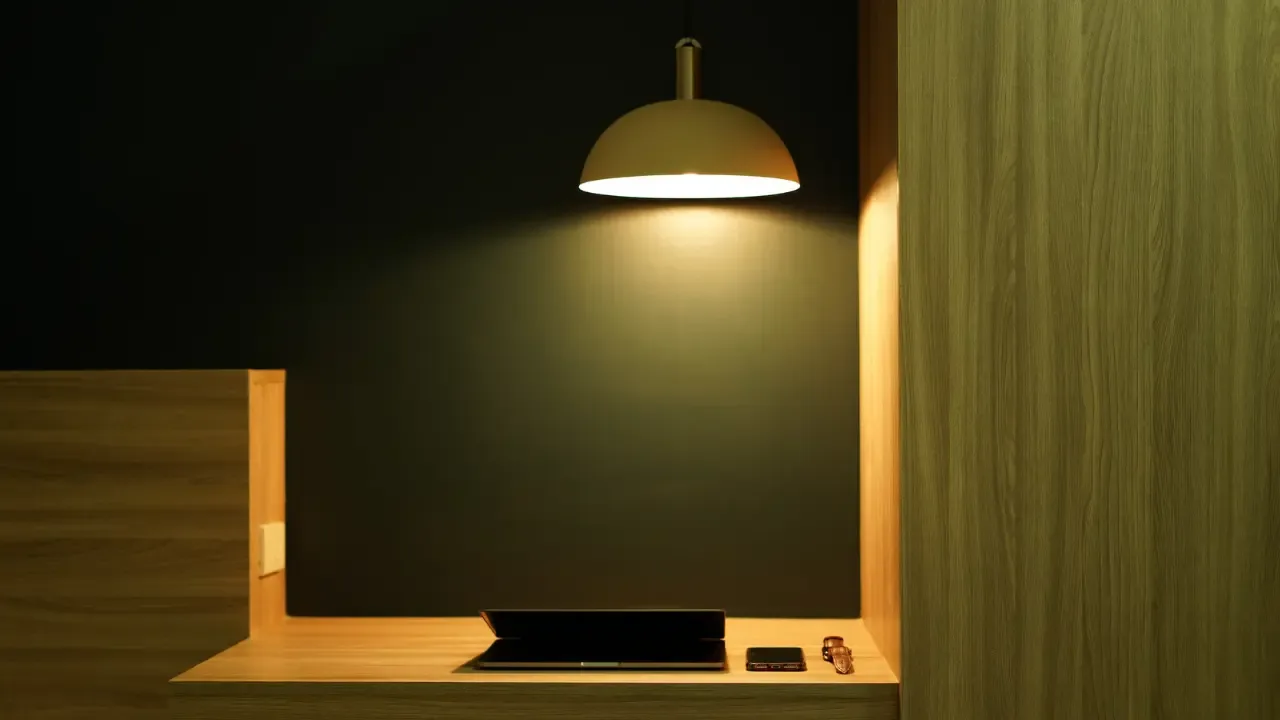
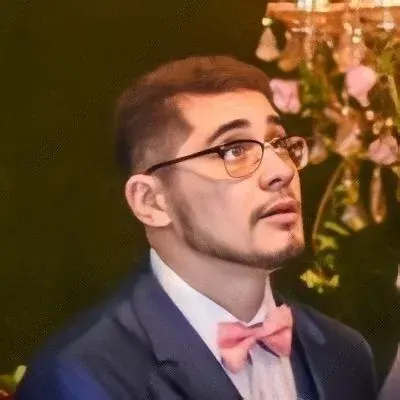
๐ซ๐ Ajax Call Failed: Insecure Response or Connection Refused?
Are you struggling to determine if your jQuery ajax call failed due to an insecure response or a connection refusal? ๐๐ก Let's dive into this common issue and find easy solutions to handle it! ๐ก
The Challenge ๐ค
Imagine you're performing a jQuery ajax call from an https server to a localhost https server running Jetty with a custom self-signed certificate. ๐ But there's a problem - you can't tell if the response failed due to the lack of certificate acceptance (insecure response) or if the localhost server is shut down (connection refused). ๐ฑ
When you check the console in Chrome, you notice two different error messages: net::ERR_INSECURE_RESPONSE
and net::ERR_CONNECTION_REFUSED
. However, using responseText
and statusCode
in your ajax call's error function, you always get consistent values of ""
and "0"
respectively. ๐ซ
The Solution โ
To determine if your ajax call failed due to an insecure response or connection refusal, you can use the errorThrown
parameter in jQuery's error callback. ๐
Here's an example of how you can modify your code:
$.ajax({
type: 'GET',
url: "https://localhost/custom/server/",
dataType: "json",
async: true,
success: function (response) {
// Do something
},
error: function (xhr, textStatus, errorThrown) {
if (errorThrown === "net::ERR_INSECURE_RESPONSE") {
// Handle insecure response error
console.log("Insecure response error occurred");
} else if (errorThrown === "net::ERR_CONNECTION_REFUSED") {
// Handle connection refusal error
console.log("Connection refusal error occurred");
} else {
// Handle other errors
console.log("An error occurred:", errorThrown);
}
}
});
By checking the value of errorThrown
, you can differentiate between the two scenarios and handle them accordingly. ๐
Manually Testing the Request ๐งช
If you prefer to perform the request manually using XMLHttpRequest
, you can still achieve the same result. ๐
Here's an example:
var request = new XMLHttpRequest();
request.open('GET', "https://localhost/custom/server/", true);
request.onload = function () {
// Handle successful response
console.log(request.responseText);
};
request.onerror = function () {
// Handle error response
var errorMessage = request.statusText || "An error occurred";
if (errorMessage === "net::ERR_INSECURE_RESPONSE") {
// Handle insecure response error
console.log("Insecure response error occurred");
} else if (errorMessage === "net::ERR_CONNECTION_REFUSED") {
// Handle connection refusal error
console.log("Connection refusal error occurred");
} else {
// Handle other errors
console.log("An error occurred:", errorMessage);
}
};
request.send();
By checking the statusText
property of the XMLHttpRequest
object, you can determine the type of error and handle it accordingly. ๐
Take Action! ๐
Now that you have the tools to determine if your ajax call failed due to an insecure response or connection refusal, go ahead and implement these solutions in your code. Don't let these errors bring you down! ๐ช And if you have any further questions or suggestions, feel free to leave a comment below. Let's conquer the tech world together! ๐๐
๐ค Join the Conversation!
Have you ever encountered issues with determining ajax call failures? How did you handle them? Share your thoughts and experiences in the comments section below! Let's learn from each other and make the tech community stronger! ๐ฌ๐ก