Detecting an undefined object property
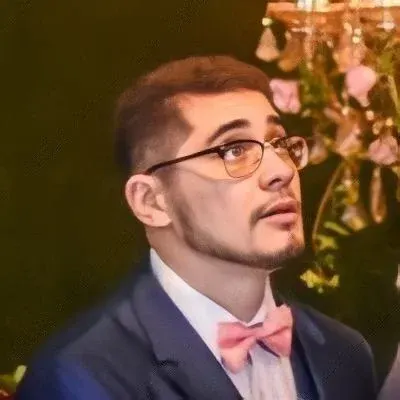
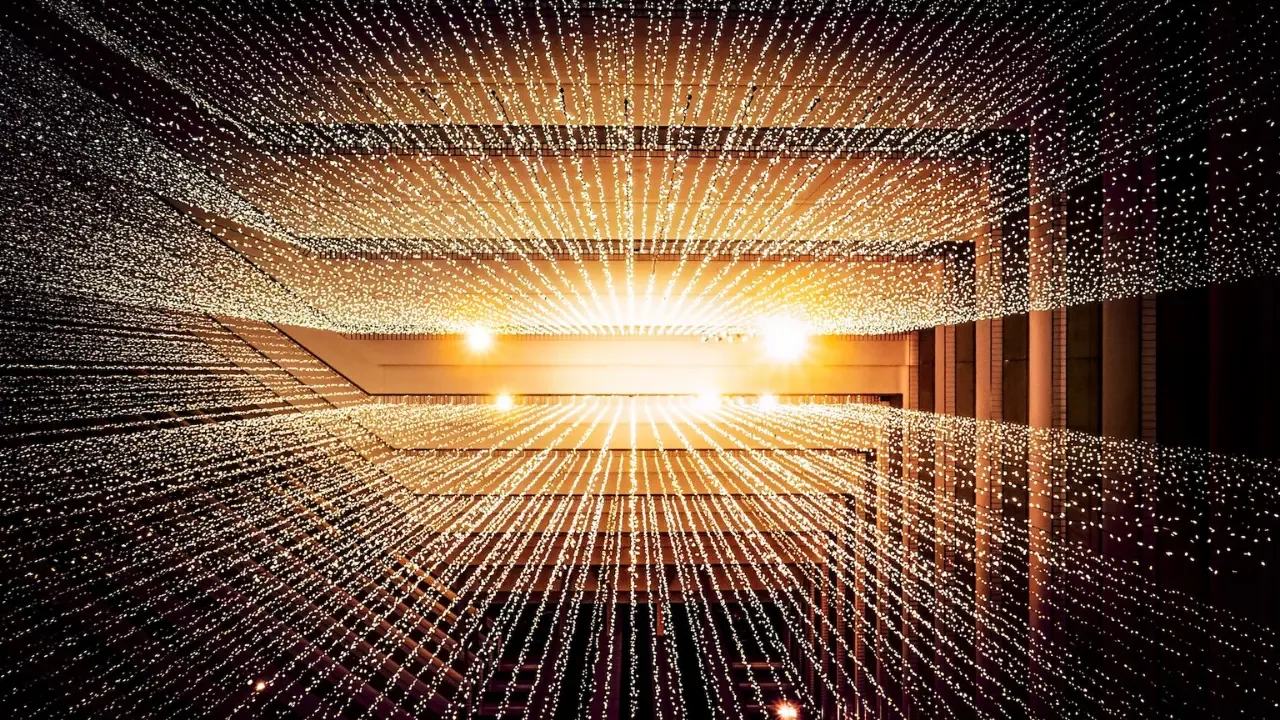
🔍 Detecting an Undefined Object Property in JavaScript
So, you've stumbled upon the age-old JavaScript question: "How do I check if an object property is undefined?" 🤔 Don't worry, my fellow tech enthusiasts! I'm here to break it down for you in a way that's easy to understand. Let's dive right in!
The Common Conundrum
Imagine you have an object with multiple properties and you want to check if a specific property exists or if it's undefined. This can occur when you're working with APIs, retrieving data from databases, or even when developing your own applications. Here's an example:
const myObject = {
name: "John",
age: 25,
};
console.log(myObject.address); // undefined
In the example above, we're trying to access the address
property of myObject
. But alas, it's undefined! How do we detect this and handle it gracefully? Let's explore some easy solutions.
🛠️ Solution 1: Using the "in" Operator
One way to check if an object property is undefined is by using the in
operator. It allows us to check if a particular property exists within an object. Let's take a look:
const myObject = {
name: "John",
age: 25,
};
if ("address" in myObject) {
console.log(myObject.address);
} else {
console.log("Property does not exist or is undefined.");
}
By using the in
operator, we first check if the address
property exists within the myObject
. If it does, we log its value. If it doesn't, we handle the undefined case.
🛠️ Solution 2: Using the typeof Operator
Another approach to detect an undefined object property is by using the typeof
operator. It allows us to determine the type of a particular value. Let's see it in action:
const myObject = {
name: "John",
age: 25,
};
if (typeof myObject.address !== "undefined") {
console.log(myObject.address);
} else {
console.log("Property does not exist or is undefined.");
}
Here, we're using the typeof
operator to check if myObject.address
is not equal to the string value of "undefined"
. If it's not, we log the value. Otherwise, we handle the undefined case.
📢 Your Turn to Shine!
Now that you've learned two solid solutions to detect an undefined object property, it's time for you to put your newfound knowledge into practice! 🙌
Consider a real-world scenario where detecting undefined object properties becomes crucial. Write some code, experiment, and share your experience in the comments below. I'd love to see how you tackle this issue!
So, remember: 🕵️ Always put on your detective hat when dealing with undefined object properties. Utilize the in
operator or the typeof
operator to gracefully handle these situations.
Stay curious, my friends! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
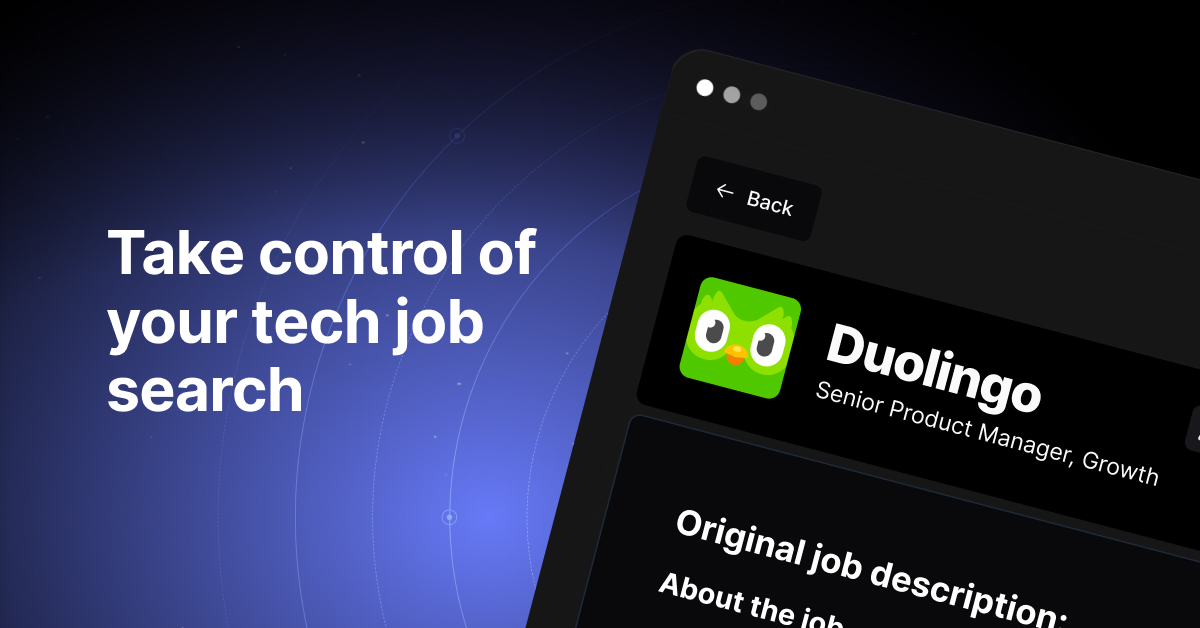