Detecting an "invalid date" Date instance in JavaScript
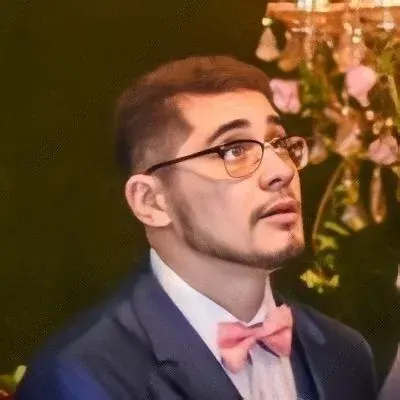
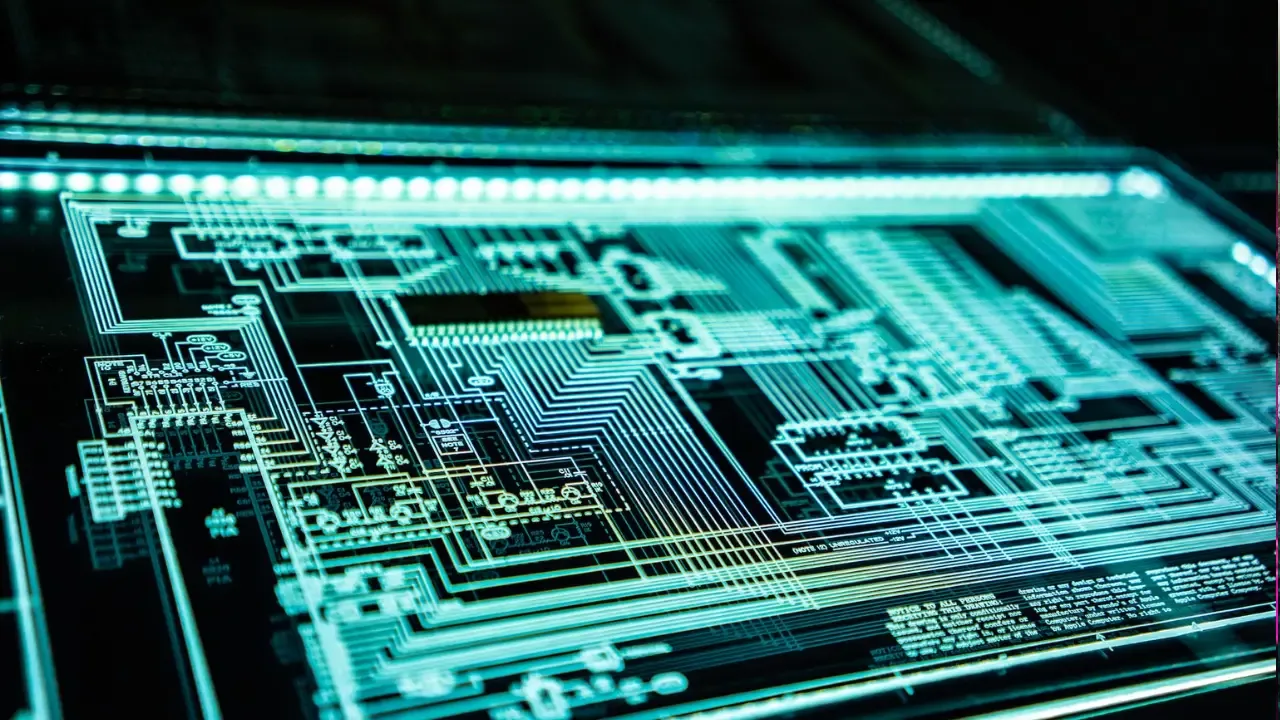
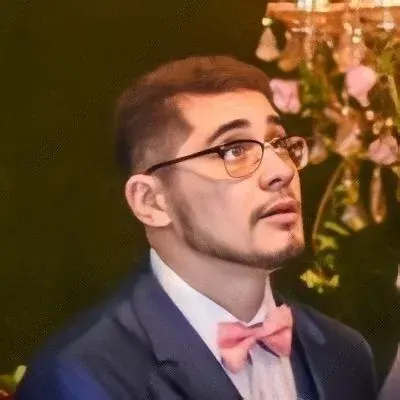
Detecting an "Invalid Date" Date instance in JavaScript 😕📅
Have you ever come across a situation where you needed to validate if a Date instance in JavaScript is valid or invalid? 🧐 It can be quite tricky to distinguish between the two, but fret not! In this article, we'll explore common issues surrounding this question and provide you with easy solutions. So let's dive right in! 💪
The Problem Scenario 📜
Here's a scenario that many developers encounter when working with Date instances in JavaScript:
var d = new Date("foo");
console.log(d.toString()); // shows 'Invalid Date'
console.log(typeof d); // shows 'object'
console.log(d instanceof Date); // shows 'true'
In this case, we have a new Date instance created with an invalid date string, where d.toString()
returns 'Invalid Date'. However, JavaScript still considers it an object of type Date
, which can make it confusing to identify whether the Date instance is valid or not. 😅
Possible Solutions ✨
Solution 1: Using Date.parse 🗓️
One possible solution, as recommended by Ash, is to use the Date.parse
method to parse the date string. This method provides an authoritative way to check if the date string is valid. You can use it like this:
function isValidDate(dateString) {
return !isNaN(Date.parse(dateString));
}
console.log(isValidDate("foo")); // returns false
Solution 2: Testing the Time Value ⌛
Another solution, suggested by Borgar, involves testing the time value of the Date instance. If the date is invalid, the time value will be NaN
. This behavior is defined in the ECMA-262 standard, making it a reliable approach.
Here's how you can implement this solution:
function isValidDate(date) {
return date instanceof Date && !isNaN(date.getTime());
}
console.log(isValidDate(new Date("foo"))); // returns false
This solution not only checks if the object is an instance of Date
, but also guarantees that the date is valid by ensuring the time value is not NaN
.
Solution 3: Avoiding Date Instances Altogether 🙅♀️
Sometimes, it may be easiest to avoid accepting Date
instances in your API altogether, as Ash suggested. By accepting date strings instead, you can use the Date.parse
method to validate them. Alternatively, you could parse the date strings yourself and perform additional checks if needed.
Engage and Learn More! 🎉
I hope these solutions help you detect and handle "Invalid Date" instances effectively in your JavaScript projects. 🎯 If you have any additional ideas or more elegant solutions, feel free to share them in the comments below. Let's help each other overcome this common challenge! 💪💬
And don't forget to share this post with your fellow developers who might find it useful. Sharing is caring, after all! 🤗✨