Detect if the internet connection is offline?
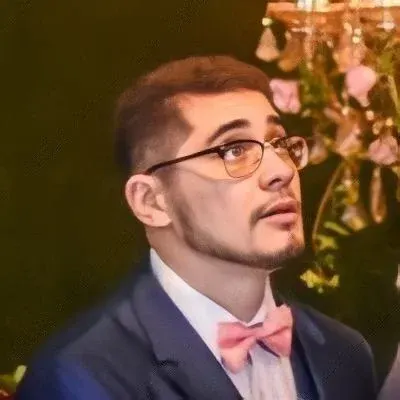
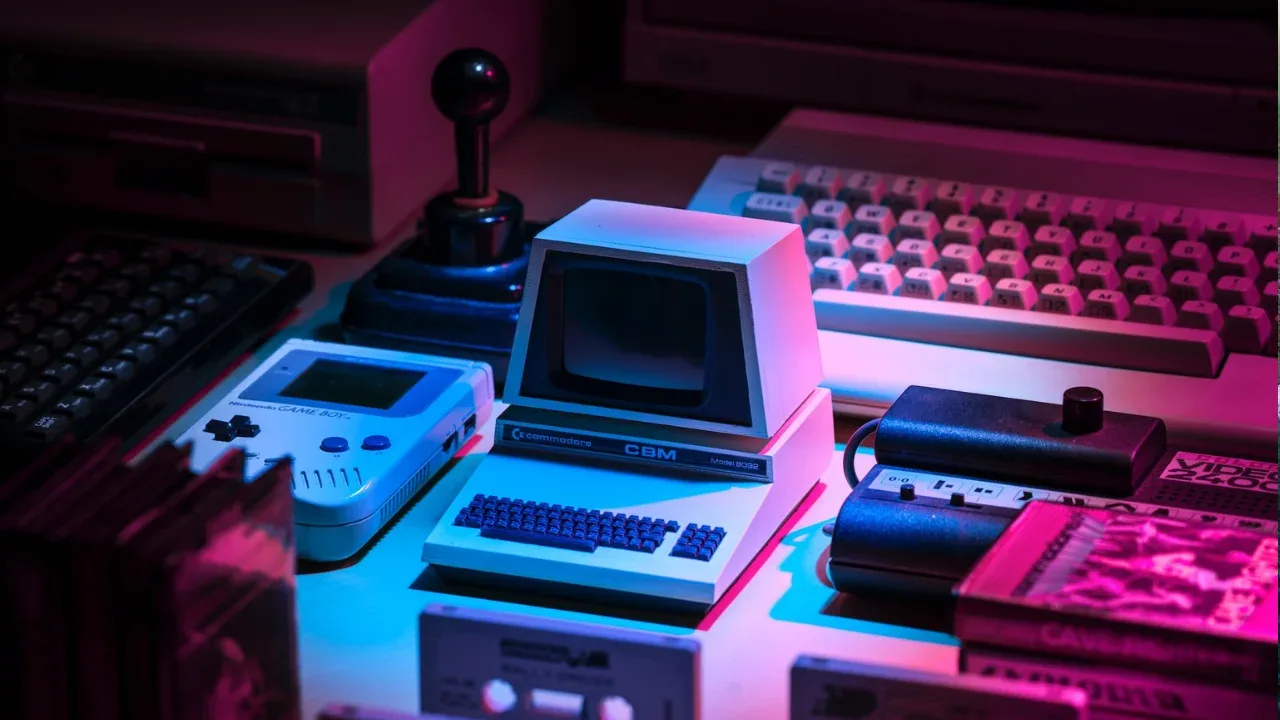
π How to Detect if the Internet Connection is Offline in JavaScript?
We've all been there - you're browsing the web and suddenly you lose your internet connection. π It can be frustrating, especially when you're in the middle of something important. But fear not! π¦ΈββοΈ In this blog post, we'll show you some nifty JavaScript techniques to easily detect if your internet connection is offline.
The Problem: Losing Internet Connection π΅
Before we dive into the solutions, let's understand the common issue we face - losing our internet connection. It happens more often than we'd like, due to various reasons like network issues, server problems, or even our own Wi-Fi acting up. But the big question is, how can we detect this offline state programmatically using JavaScript?
Solution 1: The navigator.onLine
Property π
JavaScript provides us with the navigator.onLine
property, which is a handy tool to check if we have an active internet connection. πΆ This property returns a boolean value, true
if online, and false
if offline. Here's an example of how you can use it:
if (navigator.onLine) {
console.log("You're online! π");
} else {
console.log("Oops, you're offline! π’");
}
By using navigator.onLine
, you can easily perform any offline-specific logic or provide a helpful message to the user.
Solution 2: The offline
Event Listener π§
Another way to detect if the internet connection is offline is by utilizing the offline
event listener provided by the window
object. This event is triggered whenever the browser goes from an online to an offline state. Here's an example:
function handleOfflineStatus() {
console.log("Oops, you're offline! π’");
}
window.addEventListener("offline", handleOfflineStatus);
By adding the offline
event listener and defining a callback function (handleOfflineStatus
in the example above), you can perform specific actions whenever the browser detects an offline state.
Solution 3: A Combination of Online and Offline Events β¨
For a more comprehensive approach, you can also use both the online
and offline
events together. This allows us to track changes in the internet connection status more accurately. Here's an example:
function handleOnlineStatus() {
console.log("You're back online! π");
}
function handleOfflineStatus() {
console.log("Oops, you're offline! π’");
}
window.addEventListener("online", handleOnlineStatus);
window.addEventListener("offline", handleOfflineStatus);
Now, whenever the browser goes from an online to an offline state or vice versa, the respective event listeners will be triggered, giving you more control over the user experience.
Stay Connected! π²
Now that you know how to detect if the internet connection is offline using JavaScript, you can create more robust web applications with better offline handling. Share this post with your friends and colleagues so they can stay connected too! πͺ
Have you ever encountered any interesting challenges when dealing with internet connectivity issues? Share your stories and solutions in the comments below! Let's help each other navigate through the unpredictable online world. β¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
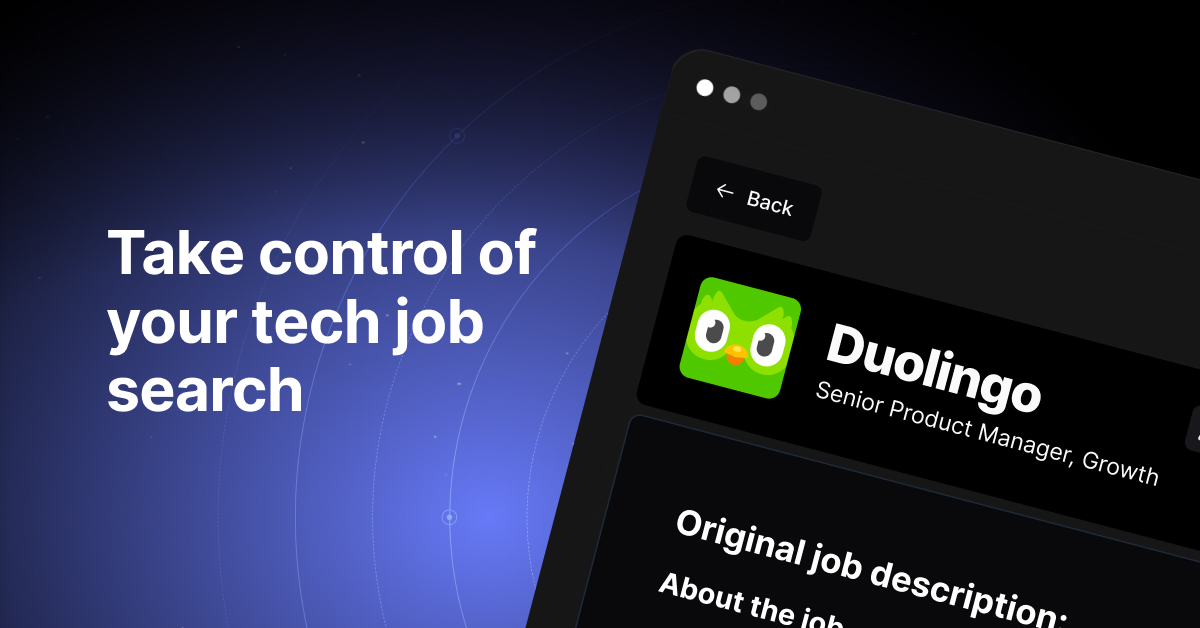