Detect if an element is visible with jQuery
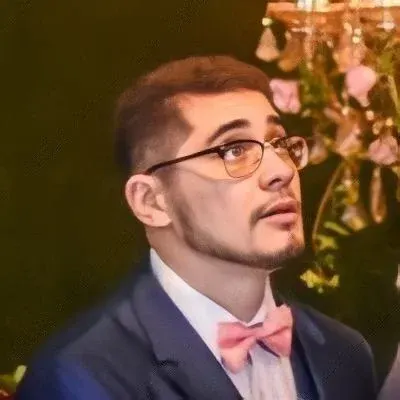

📢 Easy Guide: How to Detect if an Element is Visible with jQuery 💡
If you're looking for a way to detect the visibility of an element using jQuery, you're in the right place! In this guide, we'll address common issues and provide easy solutions for this specific problem. Let's dive in! 💪
⚠️ The Problem: Toggling Visibility with One Button ⚠️
You want to toggle the visibility of an element on your page using just one button. Currently, you have separate functions to show and hide the element. However, you'd like to simplify your code by using a single function that can dynamically toggle the visibility.
☑️ The Solution: Checking Element Visibility ☑️
To achieve this, you can use jQuery's :visible
selector to check if the element is currently visible or not. Here's how you can modify your code:
<a onclick="toggleTestElement()">Show/Hide</a>
function toggleTestElement() {
if ($('#testElement').is(':visible')) {
$('#testElement').fadeOut('fast');
} else {
$('#testElement').fadeIn('fast');
}
}
🔍 Explanation: Understanding the Code 🔍
1️⃣ We've replaced the separate show and hide functions with a single function called toggleTestElement()
. This function will be triggered when the "Show/Hide" button is clicked.
2️⃣ Inside the function, we use the is(':visible')
function of jQuery to check the visibility of the element with the ID testElement
. This function returns true
if the element is visible and false
otherwise.
3️⃣ If the element is visible (true
), we use fadeOut()
to hide it with a fade animation. If the element is not visible (false
), we use fadeIn()
to show it with a fade animation.
4️⃣ Adjust the animation speed ('fast'
in this example) according to your preference or requirements.
🤔 Wrap-Up: Detecting Element Visibility Made Easy 🎉
Using the :visible
selector alongside the is()
function in jQuery allows you to easily detect if an element is visible or not. By modifying your code to handle the toggle functionality, you can simplify your implementation and achieve the desired results.
👉 Now go ahead and give it a try! Update your HTML and JavaScript code accordingly, and enjoy the power of toggling element visibility with just one button. Don't forget to share your experience and any questions you may have in the comments below. Let's make the web more dynamic! 💪💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
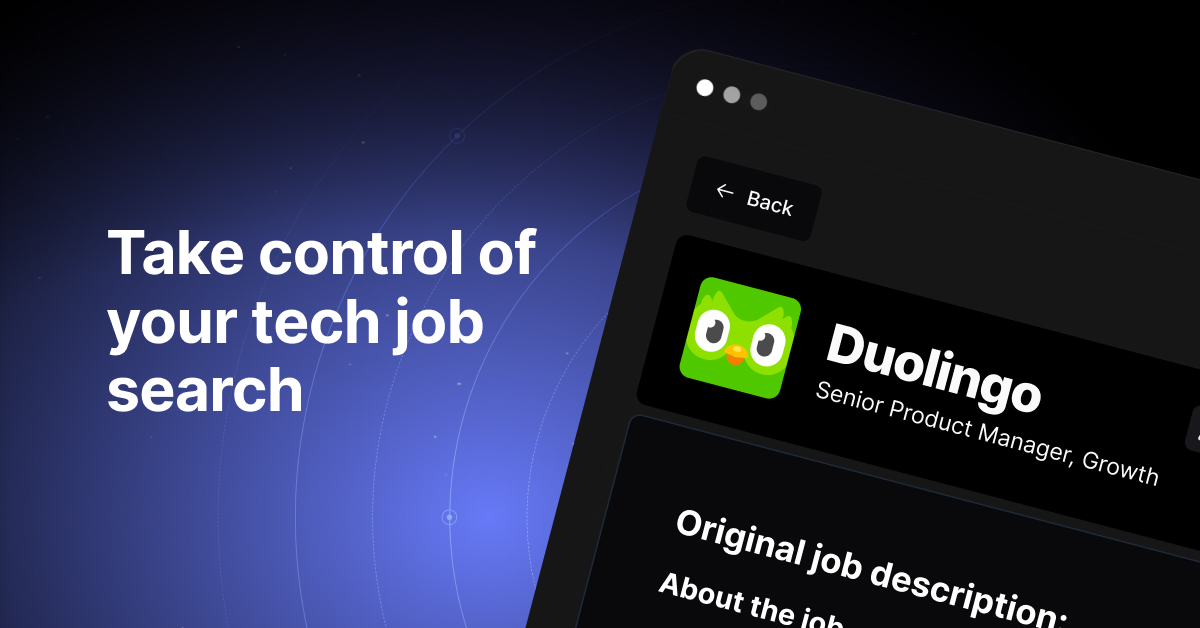