Detect click outside React component
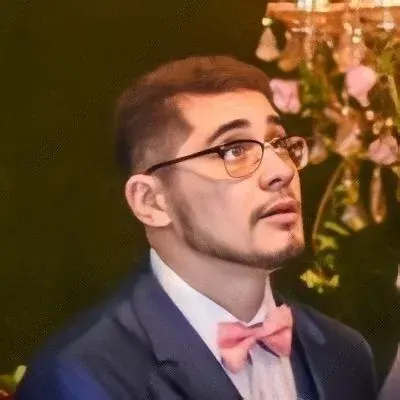
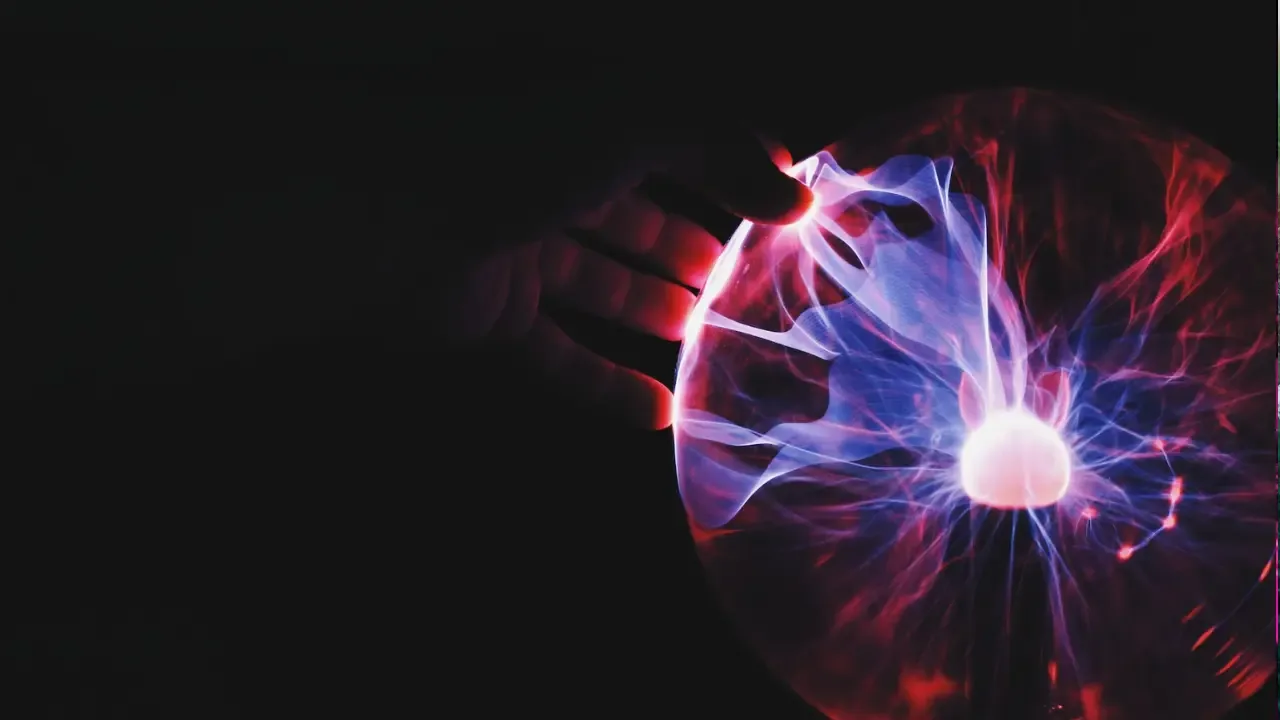
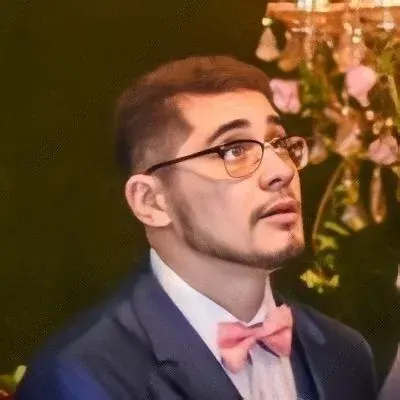
Detecting Click Outside React Component: A Complete Guide
Introduction
Do you find yourself in a situation where you need to detect if a click event has happened outside of a React component? You're not alone! This common problem often arises when we want to perform certain actions or hide certain elements when a user clicks outside a specific component. In this blog post, we will explore the issue in detail and provide easy-to-implement solutions.
Understanding the Challenge
To better understand the problem, let's break down the scenario you described:
You want to detect if a click event occurs outside of a React component.
You are familiar with the concept of jQuery's
closest()
method, which can help determine if the click event's target has the component's DOM element as one of its parents.You would like to attach a click handler to the
window
object and compare the event's target with the DOM children of your component.
Solution 1: Using Event Bubbling and Event Delegation
One popular approach to solving this problem is by utilizing event bubbling and event delegation. Here's how you can implement it:
Attach a click event listener to the
window
object within your React component.In the event handler function, access the event's
target
property to determine the element that was clicked.Use the
closest()
method, available in modern browsers, to check if the clicked element or any of its parents match your component's DOM element.If the clicked element is within your component, the event occurred inside; otherwise, it occurred outside.
componentDidMount() {
window.addEventListener("click", this.handleClickOutside);
}
componentWillUnmount() {
window.removeEventListener("click", this.handleClickOutside);
}
handleClickOutside = (event) => {
const { target } = event;
const componentNode = this.componentRef.current;
if (componentNode && !componentNode.contains(target)) {
// The click event occurred outside the component
// Perform your desired actions here
}
};
render() {
return <div ref={this.componentRef}>Your React component</div>;
}
In the above example, we attach the handleClickOutside
function as a click event listener to the window
object. We then check if the componentNode
(referring to the DOM element of your React component) contains the clicked target
element. If not, it means the click happened outside the component.
Solution 2: Using Event Propagation and Event Target
Another approach, similar to the one mentioned in the article you shared, involves using event propagation and the target element of the event. Here's how you can go about it:
Attach a click event listener to the
window
object within your React component.In the event handler function, access the event's
target
property to determine the element that was clicked.Traverse the event's
path
property, which represents the DOM path the event has traveled, to check if any of the elements match your component's DOM element.If none of the elements match, it means the click event occurred outside of the component.
componentDidMount() {
window.addEventListener("click", this.handleClickOutside);
}
componentWillUnmount() {
window.removeEventListener("click", this.handleClickOutside);
}
handleClickOutside = (event) => {
const { path, target } = event;
const componentNode = this.componentRef.current;
if (!path.includes(componentNode) && target !== componentNode) {
// The click event occurred outside the component
// Perform your desired actions here
}
};
render() {
return <div ref={this.componentRef}>Your React component</div>;
}
In the above example, we once again attach the handleClickOutside
function as a click event listener to the window
object. We check if the path
array (representing the event's path) includes our componentNode
, and also verify that the clicked target
element is not our component itself. If both conditions are true, it means the click occurred outside the component.
Conclusion
Detecting clicks outside a React component is a common challenge, but it can be easily overcome with the right approach. In this blog post, we explored two solutions:
Using event bubbling and event delegation, which checks if the clicked element or its parents match your component's DOM element.
Leveraging event propagation and the event's target element to traverse the event's path and determine if the click occurred outside the component.
Choose the solution that best fits your requirements and implement it in your React project. Happy coding!
Do you have any other questions or need further clarification on this topic? Share your thoughts in the comments below and let's discuss!