Detect a finger swipe through JavaScript on the iPhone and Android
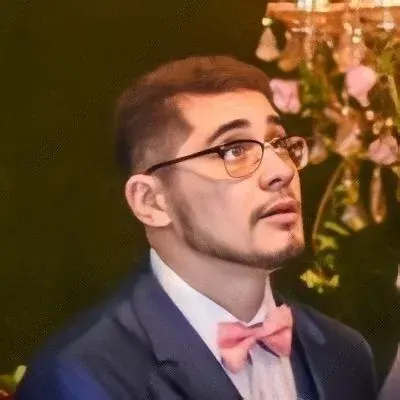
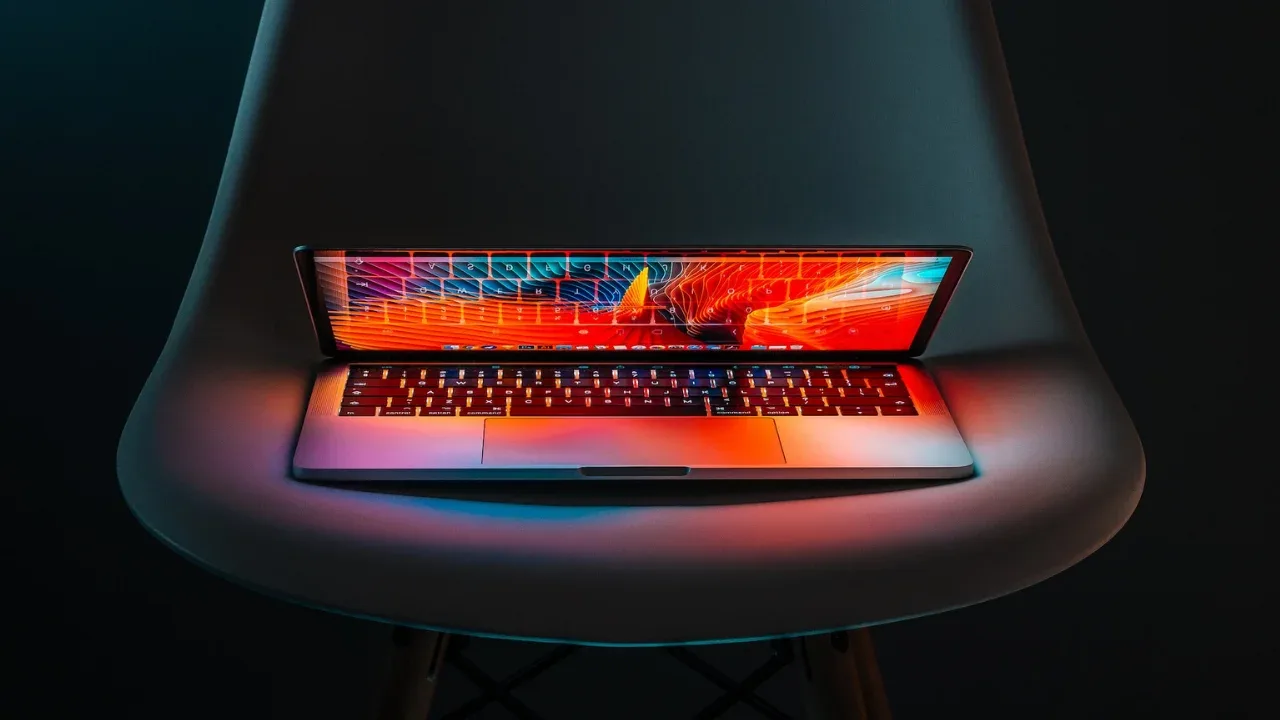
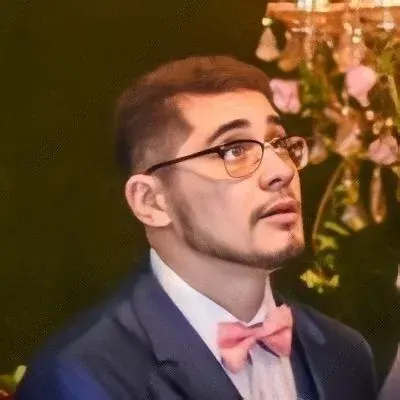
How to Detect a Finger Swipe using JavaScript on the iPhone and Android 📱🔍
Welcome back, tech enthusiasts! 👋 Today, we will explore the fascinating world of detecting finger swipes on both the iPhone and Android smartphones using JavaScript. 🌐 Whether you're a web developer or an eager learner, this guide will equip you with the necessary knowledge and easy solutions to conquer this challenge. So, let's dive in! 💪
The Challenge 🤔
Picture this: you have a fantastic web page, and you want to enhance the user experience by detecting when a user swipes their finger in any direction. However, you're faced with the dilemma of ensuring your solution works seamlessly across both iPhone and Android devices. Fear not, for we have you covered! 😎
The Solution 💡
1. Touch Events API 🖐️
To detect finger swipes across both iPhone and Android devices, we can utilize the Touch Events API. This API provides us with a set of events that allow us to tap into the touch interactions made by users on the page.
Example Code:
document.addEventListener('touchstart', handleTouchStart, false);
document.addEventListener('touchmove', handleTouchMove, false);
let startX, startY;
function handleTouchStart(event) {
const touch = event.touches[0];
startX = touch.clientX;
startY = touch.clientY;
}
function handleTouchMove(event) {
if (!startX || !startY) {
return;
}
const touch = event.touches[0];
const deltaX = touch.clientX - startX;
const deltaY = touch.clientY - startY;
// Custom logic for your swipe detection
if (Math.abs(deltaX) > Math.abs(deltaY)) {
// Swiped horizontally
} else {
// Swiped vertically
}
startX = null;
startY = null;
}
In the above code snippet, we register the touchstart
and touchmove
events on the document object. These events allow us to track the initial touch position and the subsequent touch movement on the screen.
Once we have the touch information, we can compare the initial touch position with the current touch position to determine the swipe direction (horizontal or vertical). Feel free to modify the // Custom logic for your swipe detection
section to match your specific requirements.
2. Hammer.js Library 🛠️
If you're looking for a more comprehensive solution with additional features like multi-touch support, gesture recognition, and more, then Hammer.js is the library for you. This JavaScript library simplifies touch gestures by abstracting away the complexities and providing an easy-to-use API.
Example Code:
First, include the Hammer.js library in your project:
<script src="https://cdnjs.cloudflare.com/ajax/libs/hammer.js/2.0.8/hammer.min.js"></script>
Next, utilize Hammer.js to detect the swipe gesture:
const element = document.getElementById('yourElement');
const hammertime = new Hammer(element);
hammertime.on('swipeleft swiperight swipeup swipedown', function(event) {
switch (event.type) {
case 'swipeleft':
// Handle left swipe
break;
case 'swiperight':
// Handle right swipe
break;
case 'swipeup':
// Handle up swipe
break;
case 'swipedown':
// Handle down swipe
break;
}
});
In this code snippet, we create a new Hammer
instance and attach it to the desired element. We can then listen for specific swipe events (swipeleft
, swiperight
, swipeup
, swipedown
) and perform the necessary actions accordingly.
Call-to-Action 📣
Congratulations! You've just unlocked the secrets behind detecting finger swipes using JavaScript on both the iPhone and Android devices. 🎉 Now unleash your creativity and enhance your web pages with engaging and intuitive swipe interactions. Share your awesome projects and ideas in the comments below! Let's inspire each other! 💡💬
Remember, the possibilities are endless when it comes to touch-based interactions. Experiment, explore, and never stop learning! Happy swiping! 🌟😄
Disclaimer: Please ensure your code is compatible with different browsers and versions to guarantee a smooth user experience for all.