Deleting array elements in JavaScript - delete vs splice
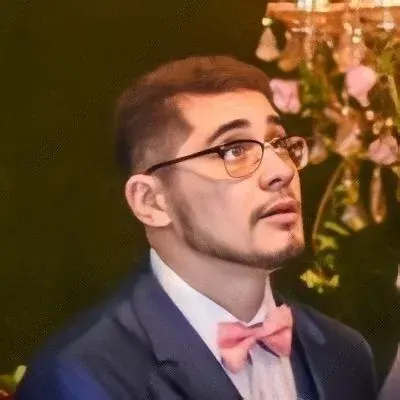
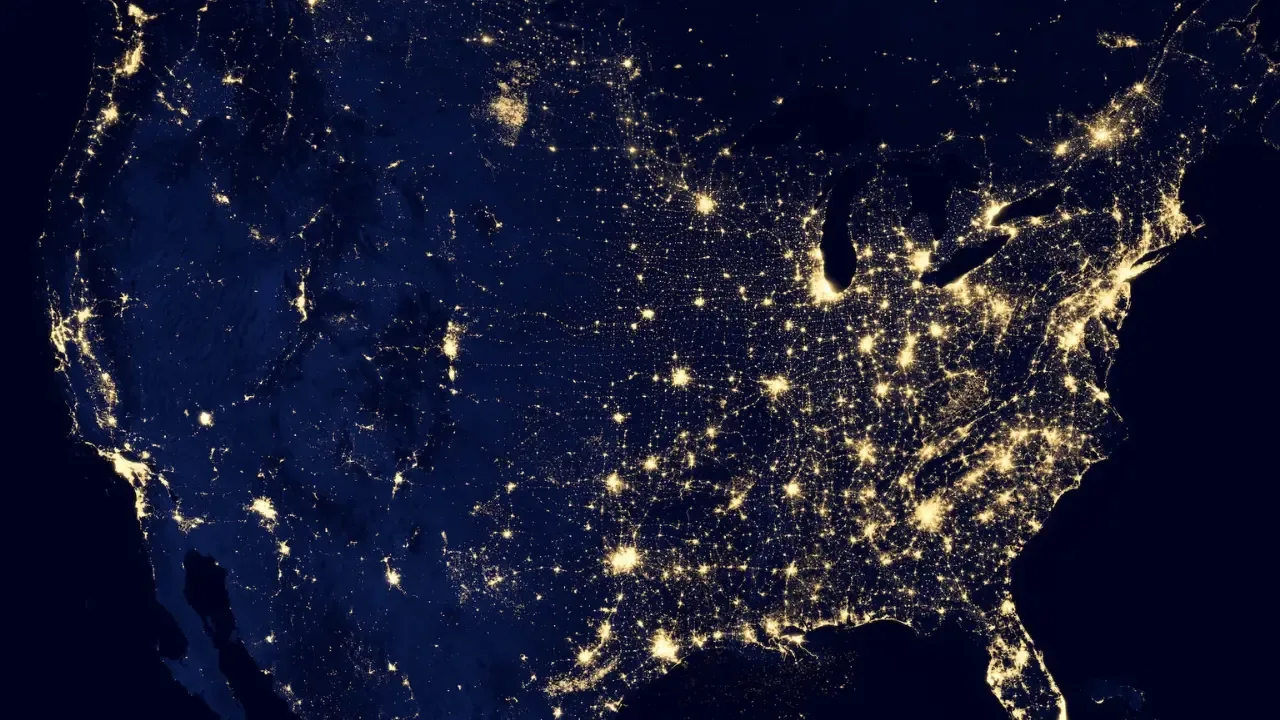
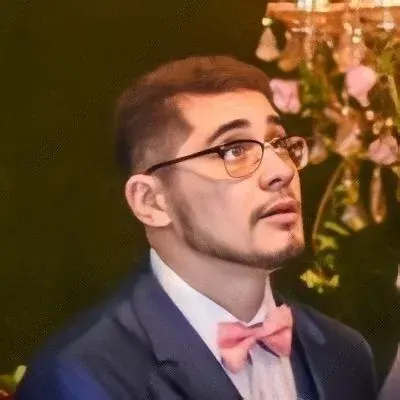
🚀 Deleting Array Elements in JavaScript: delete vs splice
Have you ever wondered what's the difference between using the delete
operator and the Array.splice
method to remove elements from an array in JavaScript? 🤔 Don't worry, you're not alone! In this blog post, we will dive deep into this question and break down common issues, provide easy solutions, and help you understand when to use each approach. Let's get started!
The Context
Let's consider the following scenario:
myArray = ['a', 'b', 'c', 'd'];
delete myArray[1];
// or
myArray.splice(1, 1);
At first glance, both approaches seem to achieve the same result, right? But the real question is: Why even have the splice
method if I can delete array elements like I can with objects? 🤷♂️
Let's break it down!
Understanding the Difference
Using the delete
Operator
The delete
operator is primarily used to remove a property from an object. While it can also be used to delete an array element, it doesn't actually remove the element or resize the array. Instead, it replaces the deleted element with the value undefined
. Here's an example:
const myArray = ['a', 'b', 'c', 'd'];
delete myArray[1];
console.log(myArray); // Output: ['a', undefined, 'c', 'd']
As you can see, the element at index 1
is replaced with undefined
, but the array's length remains the same. This might not always be the behavior you desire, especially if you want to remove the element and adjust the array accordingly.
Using the Array.splice
Method
On the other hand, the Array.splice
method is specifically designed to remove elements from an array and modify its length. It allows you to specify the index at which to start, the number of elements to remove, and, optionally, insert new elements in their place. Take a look at this example:
const myArray = ['a', 'b', 'c', 'd'];
myArray.splice(1, 1);
console.log(myArray); // Output: ['a', 'c', 'd']
In this case, splice
removes the element at index 1
and adjusts the array's length accordingly. The resulting array only contains the elements 'a'
, 'c'
, and 'd'
.
Choosing the Right Approach
Now that we understand the difference between delete
and splice
, let's discuss when to use each method.
Use delete
operator when:
You want to remove properties from an object, not necessarily elements from an array.
You don't need to resize or modify the array's length.
Use Array.splice
method when:
You explicitly want to remove elements from an array and resize it.
You need precise control over the removal process, including the ability to insert new elements.
Conclusion
In summary, the delete
operator and the Array.splice
method serve different purposes when it comes to removing elements from an array in JavaScript. While delete
replaces the element with undefined
, splice
actually removes the element and adjusts the array's length.
So, the next time you need to remove an element from an array, consider your specific requirements and choose the appropriate method accordingly.
We hope this guide has shed some light on the difference between delete
and splice
, and helped you make informed decisions in your JavaScript projects! 💡
If you found this blog post helpful, feel free to share it and leave a comment with your thoughts or any further questions. Let's keep the conversation going! 👇