Delete first character of string if it is 0
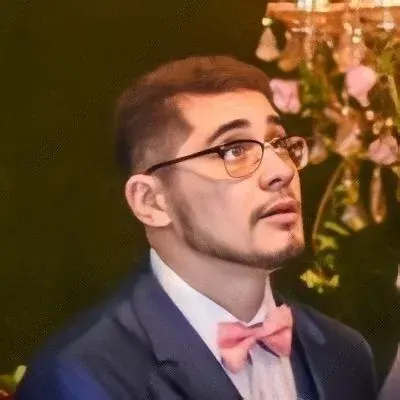
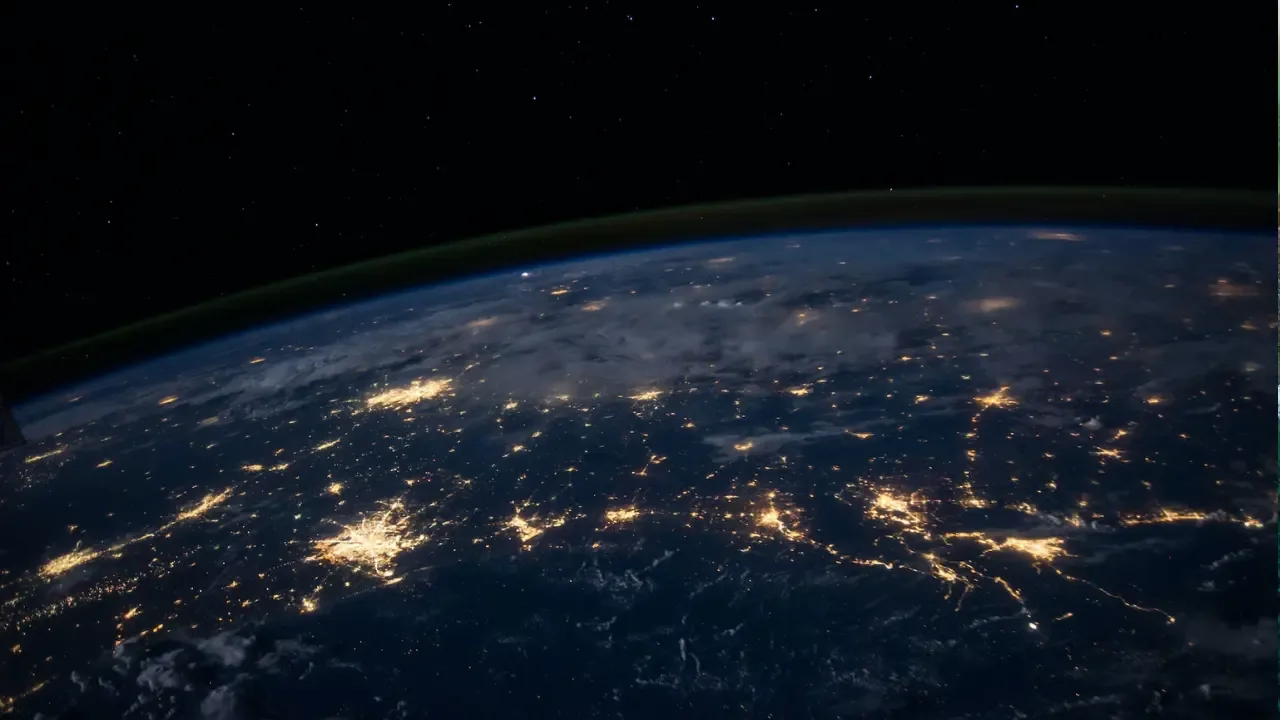
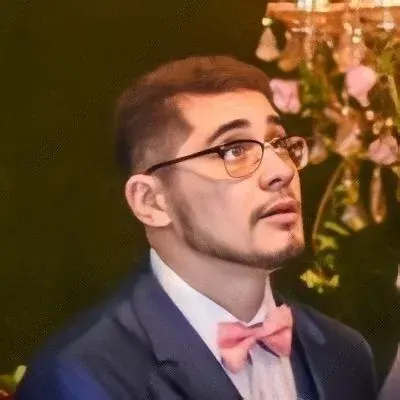
🚀 Deleting the First Character of a String if it's 0: The Ultimate Guide
Do you need to delete the first character of a string, but only if that character is a 0? We've got you covered! In this guide, we'll walk you through the common issues you might encounter and provide you with easy solutions using JavaScript. 🎉
The Problem
So, you have a string and you want to remove the first character if and only if it's a 0. No worries, we've all been there! Let's dive into the solutions.
The Awkward Approach
In the context you provided, you mentioned trying the slice()
function. While this function can indeed be used, there are simpler and more elegant alternatives. Let's explore them.
Solution 1: Regular Expressions to the Rescue
One of the easiest ways to solve this problem is by using regular expressions. Specifically, we'll use the replace()
method along with a regular expression pattern to remove the leading 0.
const originalString = "01234";
const trimmedString = originalString.replace(/^0/, "");
console.log(trimmedString); // Output: "1234"
✨ Explanation:
The regular expression pattern /^0/
matches only the leading 0 in the string. The ^
symbol indicates that the 0 should be at the beginning of the string. By replacing this 0 with an empty string, we effectively remove it. Easy, right?
Solution 2: Conditional Slicing
If regular expressions feel intimidating or you prefer a more straightforward approach, you can still use the slice()
function. Here's a neat trick to simplify the process:
const originalString = "01234";
const trimmedString = originalString[0] === "0" ? originalString.slice(1) : originalString;
console.log(trimmedString); // Output: "1234"
✨ Explanation:
We use a ternary operator (condition ? expressionIfTrue : expressionIfFalse
) to check if the first character of originalString
is equal to "0". If it is, we use slice(1)
to extract everything except the first character. If not, we simply keep the original string. Pretty cool, huh?
Your Turn to Shine! ✨
Now that you have learned two awesome ways to delete the first character from a string if it's a 0, it's time to put your new knowledge into action! Give it a go with your own strings, experiment, and have fun with it.
If you encounter any issues or have questions, leave a comment below. We'd love to hear from you and help you out.
Happy coding! 💻
Continue your coding journey with us at YourTechBlog.com, where we unleash the power of technology through engaging content! ✨