Creating multiline strings in JavaScript
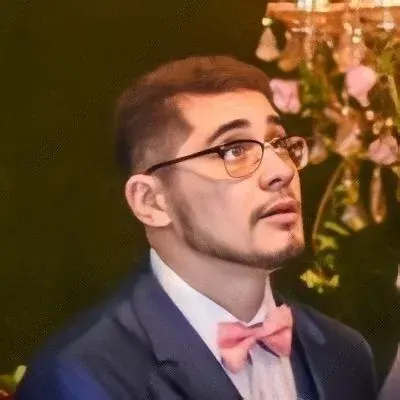
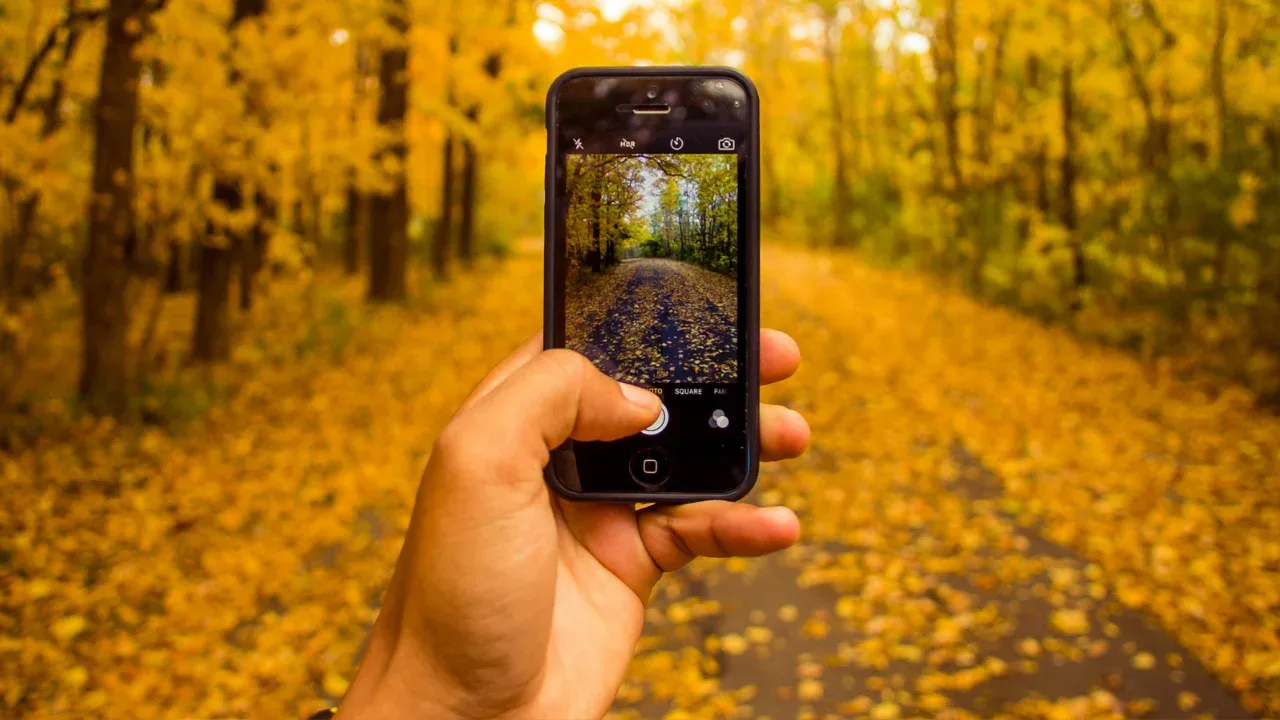
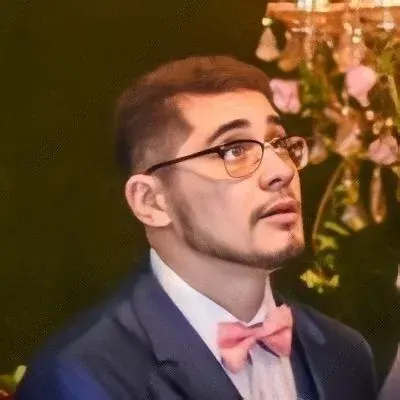
Creating Multiline Strings in JavaScript: Simplified! 😎
So, a friend of mine recently came to me with a piece of code written in Ruby and asked for its JavaScript equivalent. The code included a multiline string. After some thought, I realized that this might be a common issue many developers face while working with JavaScript. So, in this blog post, I'll guide you through creating multiline strings in JavaScript and provide you with easy solutions. Let's dive right in! 💪
The Problem 🧐
Before we move forward, let's first understand the problem at hand. Our friend's Ruby code looked like this:
text = <<HERE
This
Is
A
Multiline
String
HERE
Here, the code utilizes <<HERE
and HERE
to indicate the start and end of a multiline string. This is a handy and readable way to write multiline strings in Ruby. However, JavaScript doesn't have a built-in syntax for multiline strings, so we need to think of an alternative solution. 😤
The Solution 🚀
Thankfully, there are a few ways to address this issue in JavaScript. Here are three easy-to-implement solutions:
Solution 1: Using the Plus Operator (+
) 🤝
One way to create multiline strings in JavaScript is by using the plus operator (+
) to concatenate multiple strings:
var text = "This\n" +
"Is\n" +
"A\n" +
"Multiline\n" +
"String";
By using the plus operator, we can join the individual lines of the multiline string into one continuous string. Just make sure to add a newline character (\n
) at the end of each line to maintain the formatting.
Solution 2: Backticks (```) for Template Literals 🎭
Another approach is to use backticks (```) to create template literals. Template literals can span multiple lines without the need for explicit concatenation:
var text = `This
Is
A
Multiline
String`;
Using backticks, you can create a multiline string by simply separating your lines with line breaks.
Solution 3: Array Join Method (join()
) ⛓️
If you prefer a more organized approach, you can store each line of the multiline string in an array and then join them using the join()
method:
var lines = [
"This",
"Is",
"A",
"Multiline",
"String"
];
var text = lines.join('\n');
This solution allows you to modify or add lines more easily, as you can simply update the array elements as needed before joining them together with the desired separator.
The Call-to-Action 💬
Now that you know different ways of solving the multiline string problem in JavaScript, it's time to put your knowledge into practice! Experiment with these solutions and see which one works best for your specific use case.
If you found this blog post helpful, don't hesitate to share it with your fellow programmers and spread the word. 📣 Also, feel free to leave a comment below sharing any additional tips or alternative solutions you've come across.
Happy coding! 😄