Create an array with same element repeated multiple times
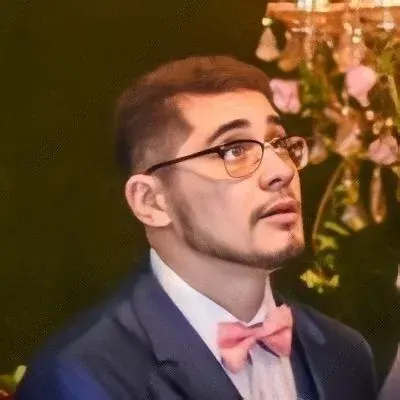
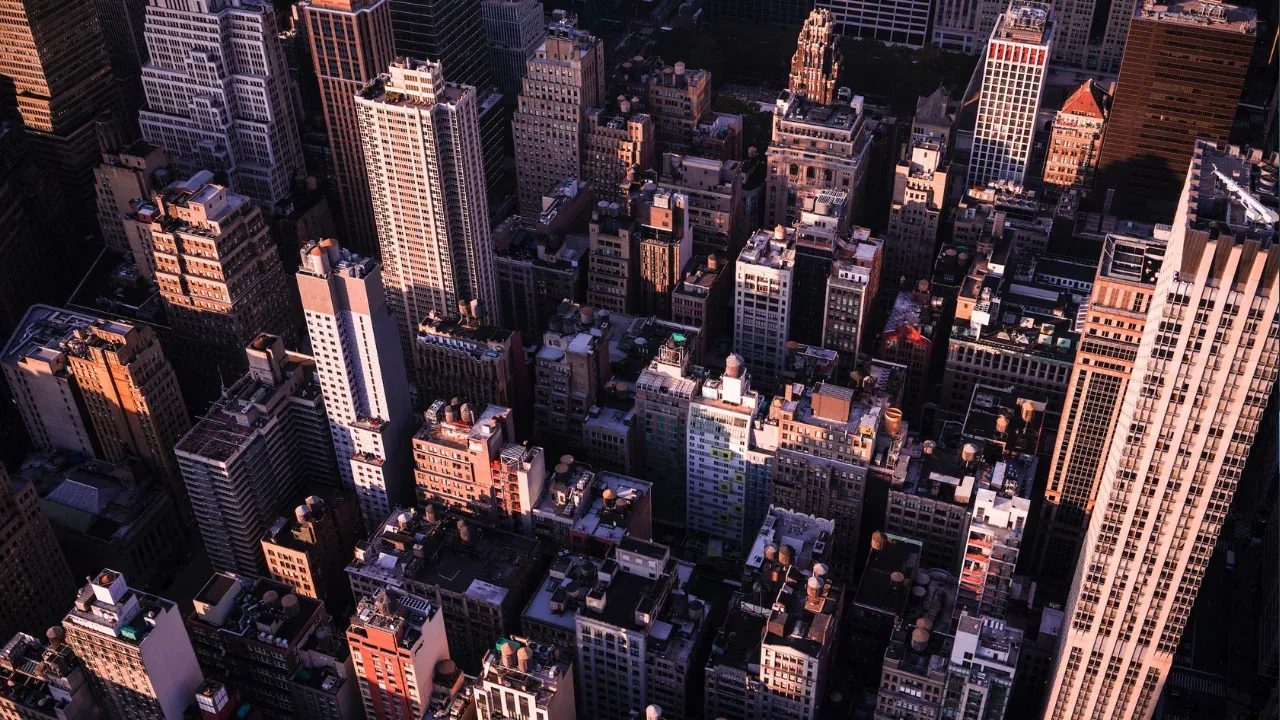
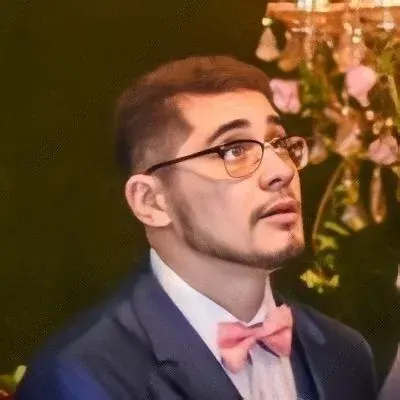
📝 Creating an Array with Repeated Elements in JavaScript
Do you often find yourself needing to create an array with the same element repeated multiple times? You're not alone! This is a common task in JavaScript, and fortunately, there are easy solutions to achieve it.
The Problem
In Python, you can easily create a list with repeated elements using the multiplication operator. For example:
[2] * 5 # Outputs: [2, 2, 2, 2, 2]
But what about JavaScript? The original poster wrote a function to solve the problem, but is there a shorter or better way?
The Solution
Yes, there is! JavaScript provides a handy method called Array.fill()
that allows us to achieve the desired result in a concise manner. Here's an example:
const repeatedArray = Array(5).fill(2);
console.log(repeatedArray); // Outputs: [2, 2, 2, 2, 2]
In the code above, we're creating a new array with a length of 5
, and then using the fill()
method to fill all the elements with the value 2
. Voila! We have our array with repeated elements.
Why is this better?
Compared to the original function provided, using Array.fill()
is simpler, more readable, and reduces the number of lines of code. It also leverages native JavaScript functionality, making it more efficient.
🚀 Your Turn to Try!
Now that you know the easy way to create an array with repeated elements, why not give it a go yourself? See if you can create an array with your favorite fruit repeated 10
times.
const repeatedArray = Array(10).fill('🍎');
console.log(repeatedArray); // Outputs: ['🍎', '🍎', '🍎', '🍎', '🍎', '🍎', '🍎', '🍎', '🍎', '🍎']
Feel free to replace '🍎'
with any other fruit emoji or element of your choice!
🌟 Share Your Creations
We hope you found this guide helpful in solving the problem of creating arrays with repeated elements in JavaScript. Now, we want to hear from you! Share your creations using the method we discussed or any other interesting solutions you come up with. Leave a comment below, and let's have some fun with arrays!
Happy coding! 💻🎉