Count the number of occurrences of a character in a string in Javascript
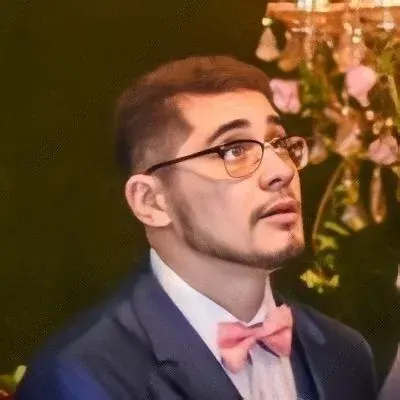
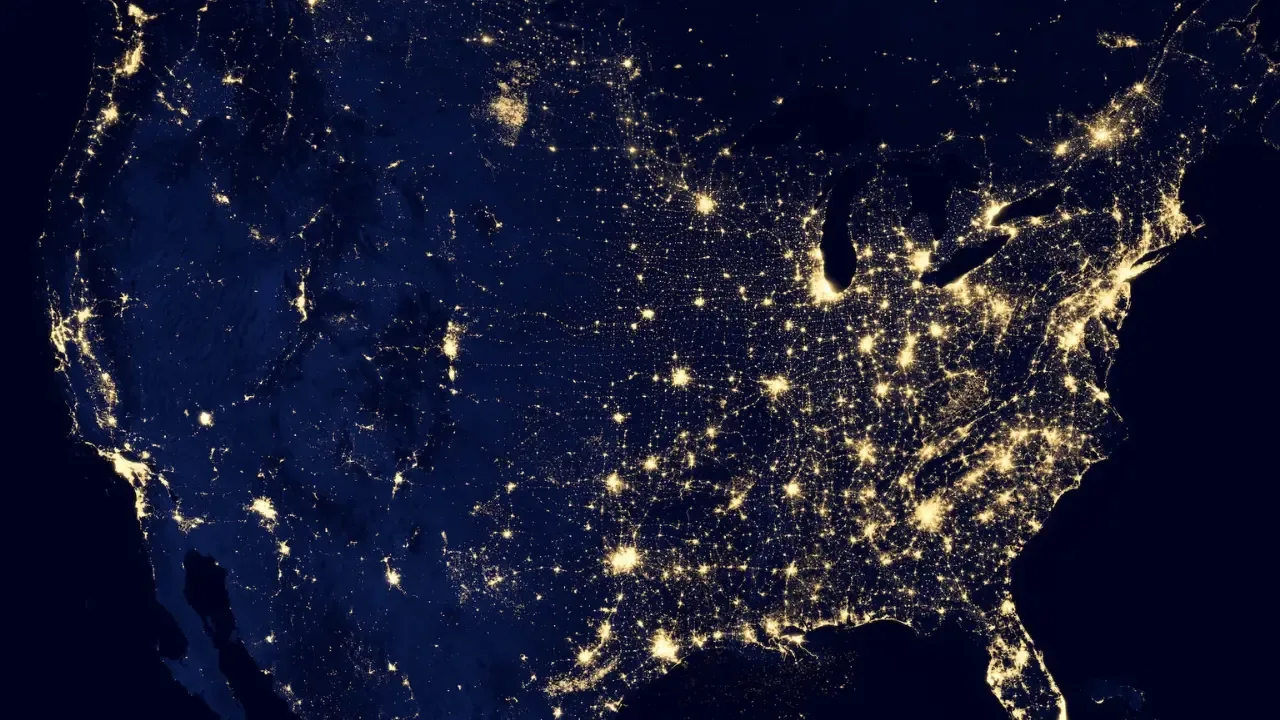
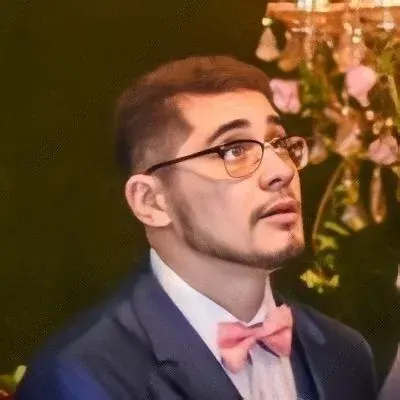
Counting the Occurrences of a Character in a String in JavaScript 😎
So, you need to count the number of occurrences of a specific character within a string in JavaScript? No worries, I've got you covered! 🙌 In this blog post, we'll explore a simple yet effective solution to this problem, address common issues, and provide some bonus tips along the way. Let's dive right in! 💪
The Problem 😕
Imagine you have a string like this:
var mainStr = "str1,str2,str3,str4";
And you want to find out how many times the comma (",") character appears in the string. In this case, the expected result would be 3. Additionally, you also want to count the number of individual strings after splitting them along the comma, and validate that each of these strings does not exceed 15 characters.
The Solution 🎉
To count the occurrences of a character within a string, we can make use of the split()
function combined with the length
property in JavaScript. Here's how you can do it in just a few lines of code:
var mainStr = "str1,str2,str3,str4";
var commaCount = mainStr.split(",").length - 1;
console.log("Occurrences of comma: " + commaCount);
And that's it! You've successfully counted the occurrences of the comma character in the mainStr
string.
But wait, there's more! 🌟
Bonus Tip: Validating String Length 💡
To validate the length of each individual string after splitting them along the comma, we can enhance our solution as follows:
var mainStr = "str1,str2,str3,str4";
var stringsArray = mainStr.split(",");
var validCount = 0;
stringsArray.forEach(function(str) {
if (str.length <= 15) {
validCount++;
}
});
console.log("Valid strings count: " + validCount);
Now, you'll get the count of valid strings (i.e., those with a maximum length of 15 characters) printed on the console.
Let's Engage! 🚀
Congratulations on mastering your JavaScript skills and successfully counting character occurrences within a string! I hope this guide was helpful on your coding journey. Now, show off your knowledge and put it into practice! 🎓
Challenge: Try applying this solution to a different string with a different character. Share your code and results in the comments section below. I can't wait to see what you come up with! ✨
Remember, the key to improving your coding skills is practice, so keep coding, keep learning, and keep rocking those JavaScript projects! 💪🔥
Stay tuned for more exciting tech tips and guides on our blog. If you found this post helpful, don't forget to share it with your fellow developers. Sharing is caring! ❤️
Happy coding! ✌️😃