Correct modification of state arrays in React.js
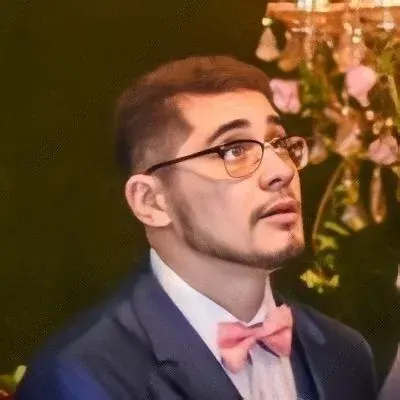
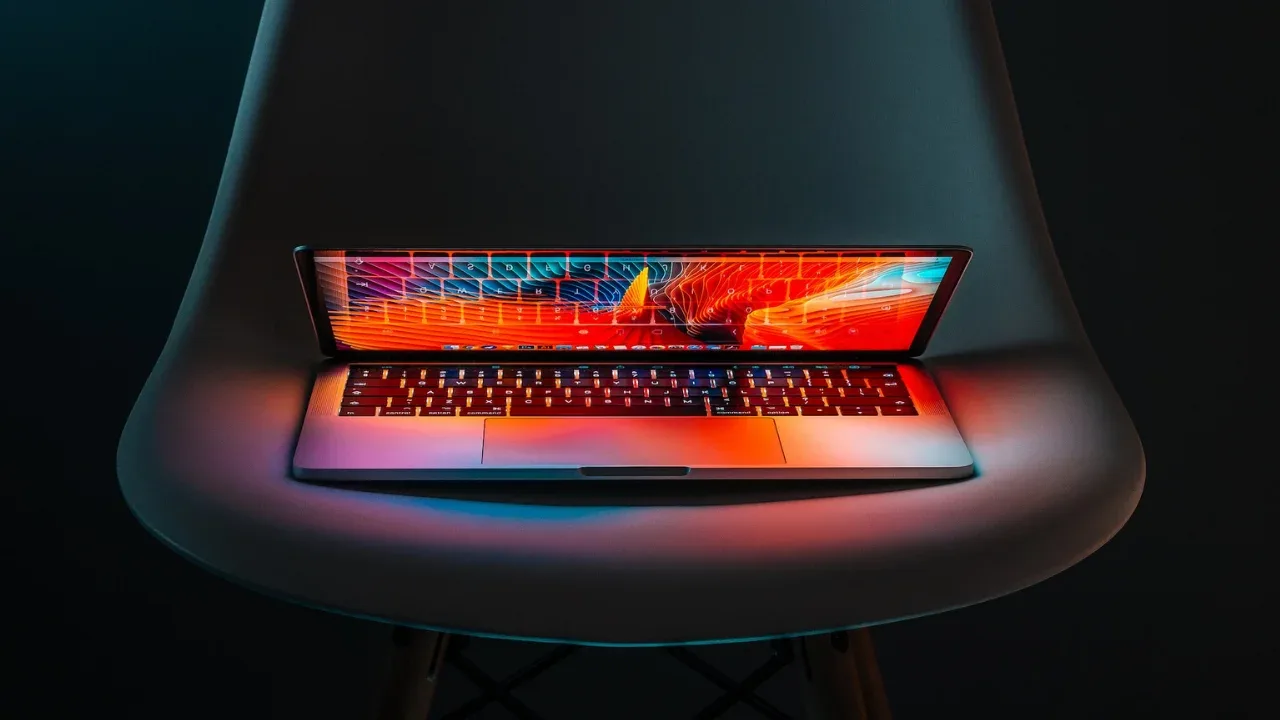
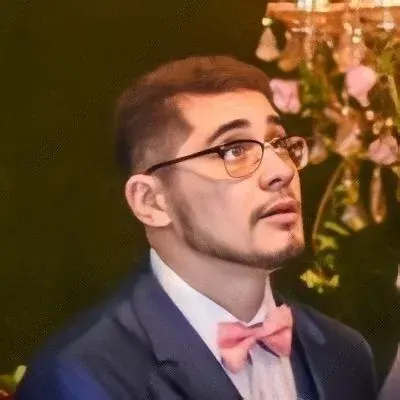
๐ Title: Modifying State Arrays in React.js: Best Practices for a Smooth Experience ๐ป๐
Introduction
Welcome, React enthusiasts! Today, we tackle a common dilemma many of us face when it comes to modifying state arrays in React.js. We'll explore the correctness and safety of using push()
to add elements to an array and discuss an alternative approach that avoids potential issues. Let's dive right in! ๐โโ๏ธ
The "push()" Predicament
So, you want to add an element to the end of a state
array. You might be tempted to use push()
to quickly achieve this, like so:
this.state.arrayvar.push(newelement);
this.setState({ arrayvar: this.state.arrayvar });
The Safety Concern
While this approach may initially work and seem convenient, it can lead to trouble down the line. React's state management relies on immutability to efficiently detect and propagate changes. When you directly modify an array with push()
, you breach this immutability principle, potentially causing bugs and unpredictable behavior. ๐จ
๐ Say No to Direct Modification!
To ensure a reliable and bug-free React experience, it's best to avoid modifying arrays directly within your state. But fear not, we have an elegant solution that will keep your code clean, efficient, and error-free. ๐ช
The Immutable Solution
To add an element to the end of a state array without compromising React's immutability principle, we'll employ the mighty JavaScript spread operator (...
). ๐
const newArrayVar = [...this.state.arrayvar, newElement];
this.setState({ arrayvar: newArrayVar });
By creating a new array (newArrayVar
), we spread the existing elements of this.state.arrayvar
and append the newElement
. We then setState with the new array, ensuring immutability and React's proper behavior. ๐
The Performance Conundrum
But wait, won't creating a new array every time we add an element be inefficient and memory-consuming? ๐ค
A Clever Optimization
React understands the potential performance impact of copying large arrays. It performs a smart optimization known as "shallow comparison" when you setState with a new array. If the new array's elements are the same as the old array's elements, React will not clone the entire array, saving both time and memory. Isn't that just groovy? ๐
So, fret not, friend! React's got your back, and performance won't be an issue. ๐โโ๏ธ
Conclusion and Call-to-Action
You've now unlocked the secret to correctly modifying state arrays in React.js. Remember to steer clear of direct modifications with push()
and opt for the immutable solution using the spread operator. This ensures reliability, bug-free code, and optimal performance. ๐
Now, it's your turn! Have you encountered any issues with state arrays in React? How did you handle them? Share your experiences, tips, and tricks in the comments section below! Let's build a vibrant React community together! ๐ฌ๐ก
Happy coding, and may your state arrays always remain immutable! ๐งก
๐๐ก๐๐ป