Copy array items into another array
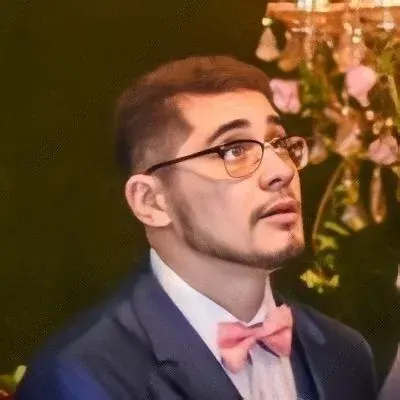
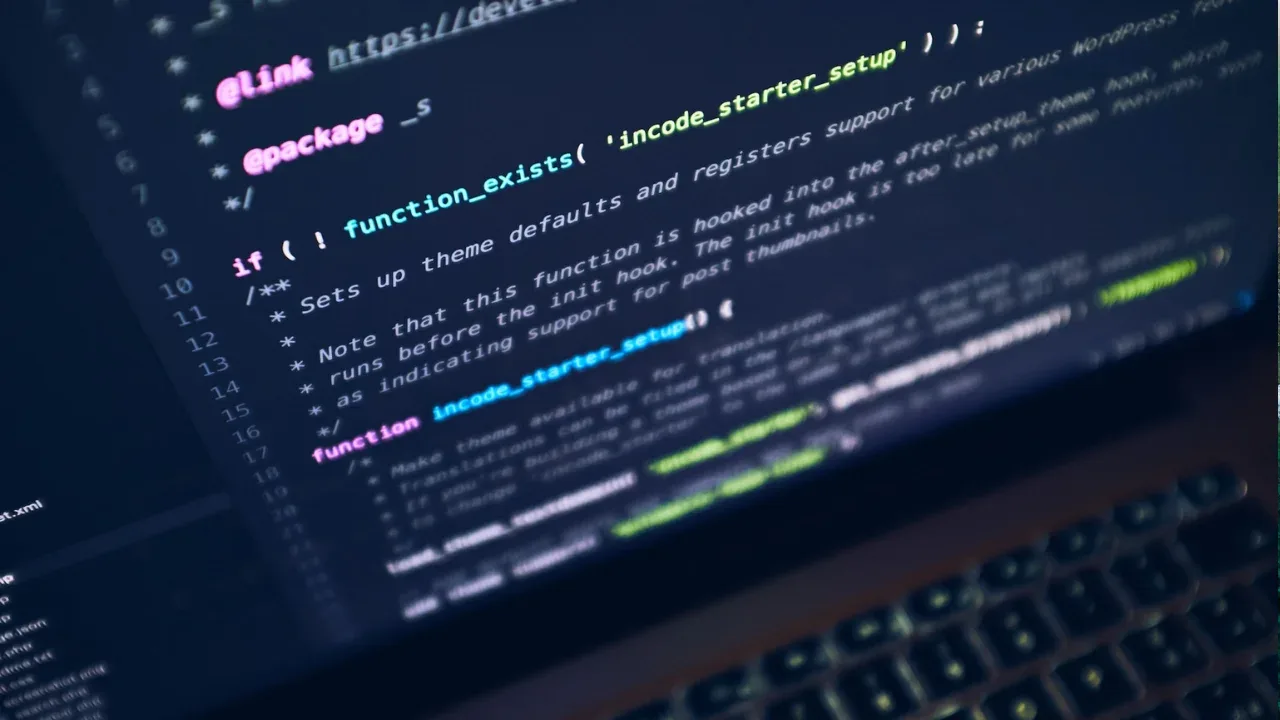
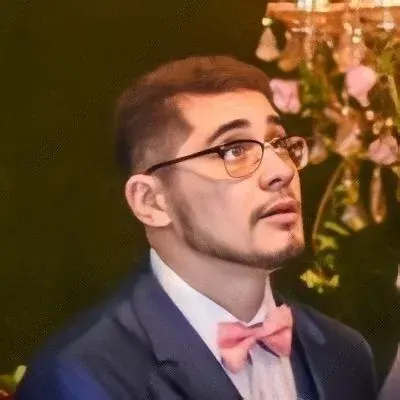
📝 Tech Blog: Copy Array Items like a Pro! 💪
Are you tired of manually iterating over arrays to push their items into a new array? 😫 Well, my friend, worry no more! In this blog post, I'll show you some easy solutions to swiftly copy array items into another array. 💨💥
The Challenge: Copying All Array Items
Imagine you have a JavaScript array called dataArray
, and you want to push all its items into a new array called newArray
. But hey, you don't want dataArray
itself as the first item in newArray
. You want to push in each individual item! 🙌
Here's the code snippet that represents the challenge:
var newArray = [];
newArray.pushValues(dataArray1);
newArray.pushValues(dataArray2);
// ...
Or, if you prefer a more compact approach:
var newArray = new Array(
dataArray1.values(),
dataArray2.values(),
// ...
);
With this code, newArray
should contain all the values from the individual data arrays. But how can you achieve this without resorting to manually iterating over each dataArray
? 🤔
Easy Solution: Using the Spread Operator
Ah, the spread operator! It's like magic in JavaScript! 🎩✨ To solve our problem, we can leverage this handy operator to copy array items into a new array effortlessly.
Here's how you can do it:
var newArray = [...dataArray1, ...dataArray2, ...dataArray3];
With this ingenious one-liner, you can combine the items from multiple arrays into a single newArray
. The spread operator expands each individual array and adds its items to the new array. Beautiful, isn't it? 😍
A Shorthand Alternative: Using concat() Method
If you prefer a more traditional approach, fear not! JavaScript has a built-in method called concat()
that can help us out.
Let's take a look at the code:
var newArray = dataArray1.concat(dataArray2, dataArray3);
With the concat()
method, you can pass in any number of arrays as arguments, and it will concatenate them together, creating a new array with all the items. 🎉
Call to Action: Have fun coding! 🚀
Now that you have two awesome methods in your coding arsenal, you can copy array items into another array like a pro! Say goodbye to laborious manual iterations! 😎
Give the spread operator and concat()
method a try in your next JavaScript project. Embrace the power of simplicity in your code! 💪 And if you have any other cool tips or tricks, share them in the comments below. Let's help each other become better developers! 🙌💻
Happy coding! ✨
Note: This blog post assumes a basic understanding of JavaScript. If you're new to the language, don't worry! Check out some beginner-friendly JavaScript tutorials and start your coding journey!