Copy array by value
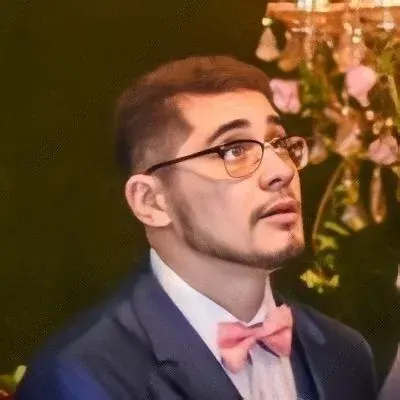
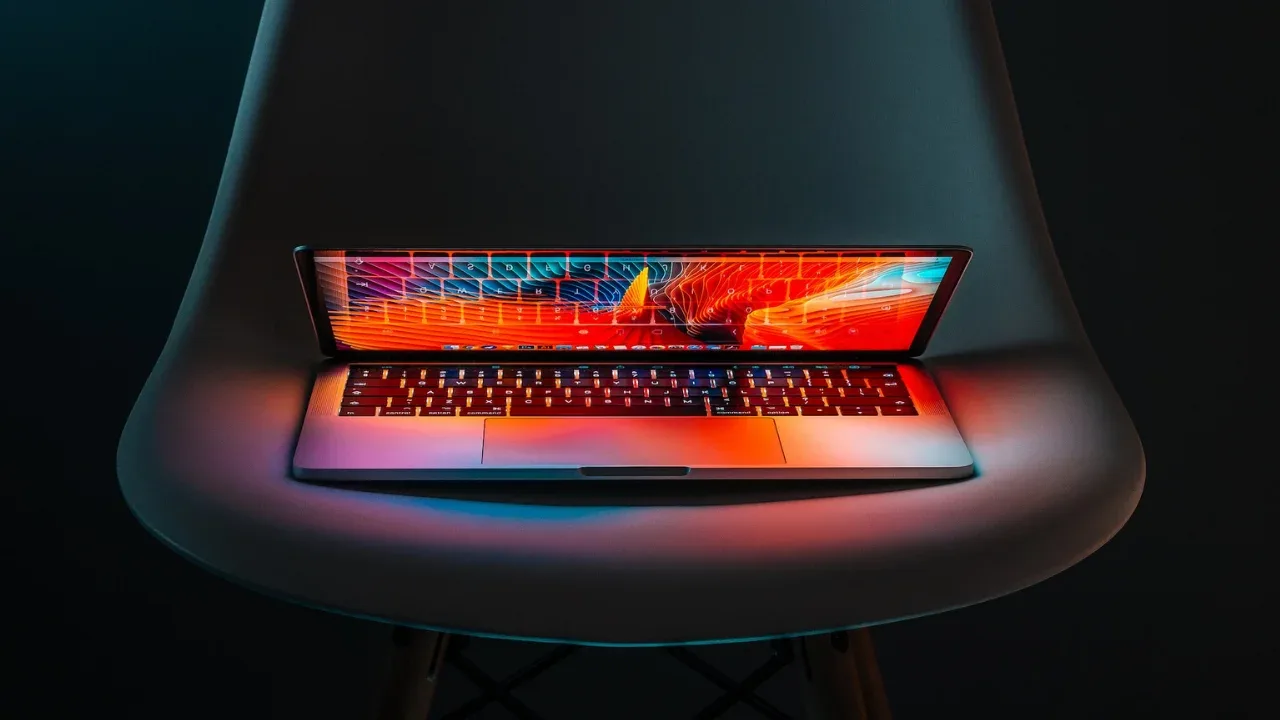
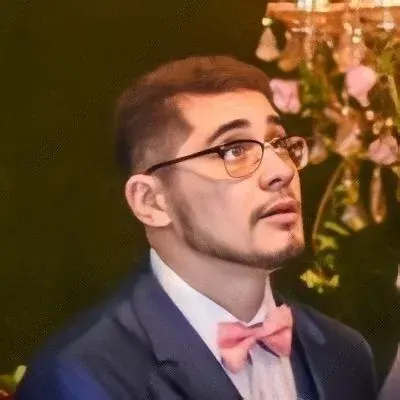
💡 Copy Array By Value in JavaScript
Copying an array in JavaScript seems like a straightforward task, but it can often lead to unexpected results. The default behavior of assigning an array to a new variable is that both variables reference the same underlying array. This means that any changes made to one variable will affect the other.
Let's take a look at an example:
var arr1 = ['a','b','c'];
var arr2 = arr1;
arr2.push('d'); // Now, arr1 = ['a','b','c','d']
In this code snippet, we expect arr2
to be an independent copy of arr1
, but that's not the case. Pushing a new element to arr2
also modifies arr1
. This behavior can lead to unexpected bugs and make it hard to work with arrays that need to be separate.
🚩 The Problem: Reference Types
To understand why this happens, we need to talk a bit about how JavaScript handles arrays and other reference types. In JavaScript, arrays, objects, and functions are reference types. When assigned to a variable or passed as an argument to a function, reference types are not copied by value, but rather by reference. This means that the variable actually holds a reference to the original array in memory.
🛠️ Solution 1: The Spread Operator
One way to copy an array in JavaScript is to use the spread operator. The spread operator allows us to expand an array or any iterable into individual elements. By using the spread operator, we can create a new array with the same values as the original one.
var arr1 = ['a','b','c'];
var arr2 = [...arr1]; // Using the spread operator
arr2.push('d'); // Now, arr1 = ['a','b','c'] and arr2 = ['a','b','c','d']
By spreading arr1
into arr2
, we create a new array with the same values. Modifying arr2
will no longer affect arr1
. The spread operator is supported in modern browsers and is a concise way to achieve array copying.
🛠️ Solution 2: Array.from()
Another way to create a copy of an array is by using the Array.from()
method. This method creates a new array from an iterable object, such as an array or a string.
var arr1 = ['a','b','c'];
var arr2 = Array.from(arr1); // Using Array.from()
arr2.push('d'); // Now, arr1 = ['a','b','c'] and arr2 = ['a','b','c','d']
The Array.from()
method creates a new array with the same values as arr1
. Once again, modifying arr2
will not affect arr1
. This method provides a more explicit way to create a copy of an array and is supported in modern browsers.
📢 Conclusion and Call-to-Action
Copying an array by value is an important concept to understand in JavaScript, especially when working with reference types. By using the spread operator or the Array.from()
method, you can create independent copies of an array, avoiding unwanted side effects.
The next time you need to work with arrays that need to be separate, remember these techniques to create copies that won't affect each other.
If you found this guide helpful, share it with your JavaScript developer friends 🤝. Have any questions or other ways to copy arrays? Let's discuss in the comments below!