Converting user input string to regular expression
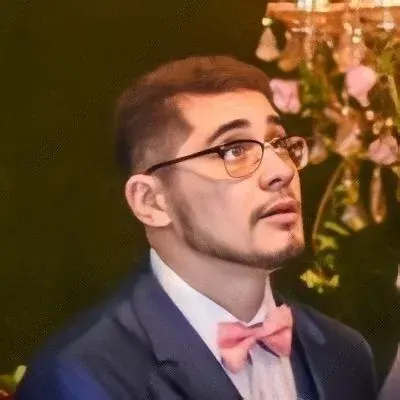
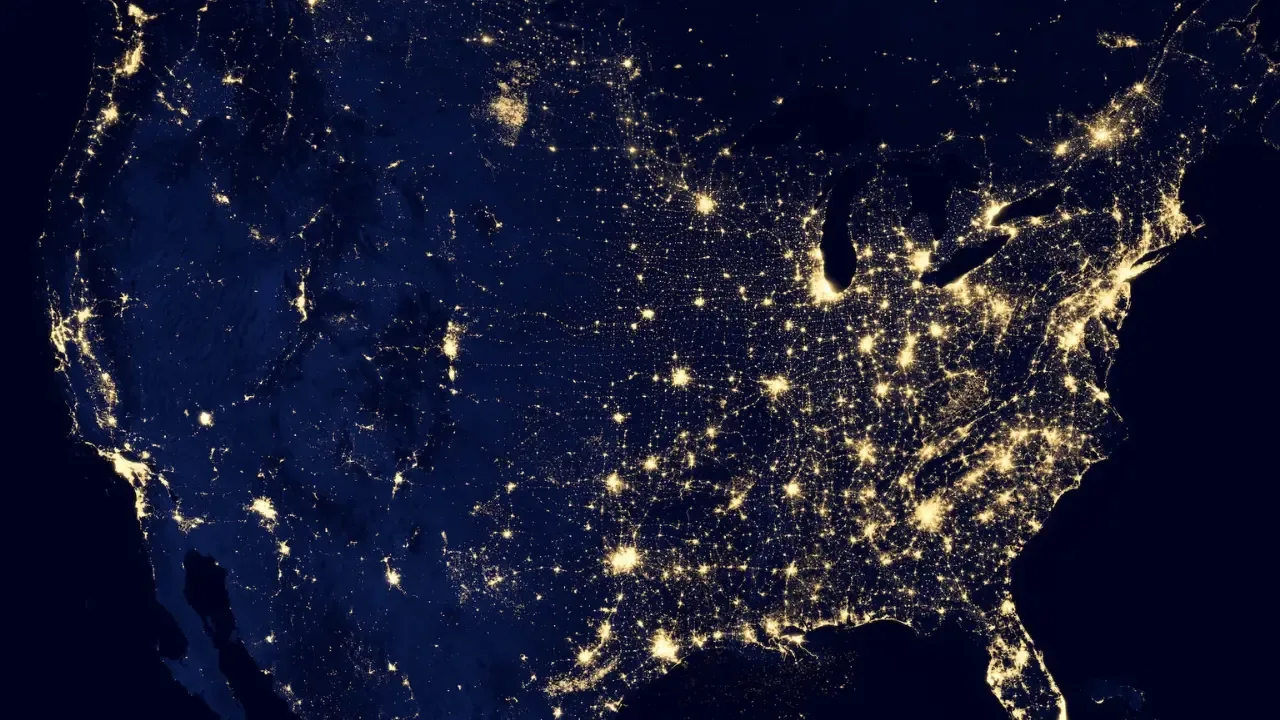
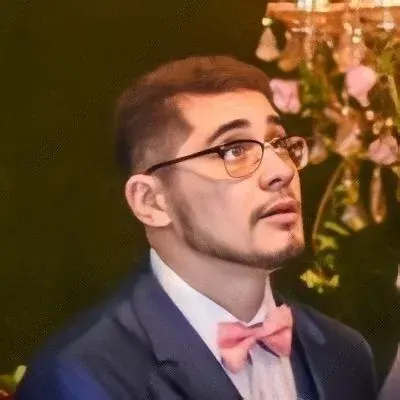
Converting User Input String to Regular Expression: A Beginner's Guide 👨💻🔀🔍
So you're building a regular expression tester and you're facing the age-old challenge of converting a user's input string into a proper regular expression. Fear not! In this blog post, we will address this common issue and provide you with easy solutions to tackle it like a pro. 💪
Understanding the Problem 🤔
Let's begin by breaking down the problem. As you mentioned, the user will input their desired regular expression but might not include the necessary forward slashes (//
) or need to set flags. This presents two challenges:
Missing Forward Slashes: Without the forward slashes, the user's input would be treated as a literal string rather than a regular expression.
Flags: The user may want to specify flags like
g
for global ori
for case-insensitive matching, but how do we incorporate those into the regular expression?
Solution 1: Parsing the User's Input 📝
One way to overcome this challenge is by parsing the user's input and construct the regular expression object separately. Here's how you can do it:
Ask the user to provide the regular expression as a string without the forward slashes.
Ask the user to provide the flags separately, if any.
Using JavaScript, parse the user's input and create a regular expression object using the
RegExp
constructor.
Let's take an example to clarify the process. Suppose the user's input is blog
, and they want a case-insensitive search. They would enter:
Regular Expression:
blog
Flags:
i
In JavaScript, you can convert this input to a regular expression like this:
const regexString = "blog"; // User's input for the regex
const flags = "i"; // User's input for the flags
const regex = new RegExp(regexString, flags);
By separating the regular expression and flags, you have the flexibility to handle various scenarios without relying on the presence of forward slashes.
Solution 2: Validate and Format User's Input ✅📏
Another approach is to validate the user's input and ensure they provide the regular expression in the correct format. This way, you can guide them to input the string accurately without the need for additional parsing. Here's how you can accomplish this:
Inform the user about the expected format, mentioning the need for forward slashes (
//
) around the regular expression.Validate the user's input to ensure they follow the required format.
If necessary, prompt the user to provide flags separately.
For example, let's assume the user wants to search for the word "hello" case-insensitively. You can guide them to enter the regular expression as /hello/i
.
This approach simplifies the process for the user as they only need to provide the regular expression in the prescribed format.
Conclusion and Call-to-Action 🎉🔃
Converting user input strings into regular expressions doesn't have to be a daunting task. By using either of the two solutions we discussed, you can handle this challenge effortlessly in your regular expression tester.
Now it's your turn to implement these techniques in your own projects! Let us know in the comments below which solution you prefer or if you have any questions. Join the discussion and share your experiences with other tech enthusiasts like yourself! 🗣️🚀
Remember, mastering regular expressions can greatly enhance your coding skills and open up a new world of possibilities in text processing. Happy coding! 💻💥