Converting any string into camel case
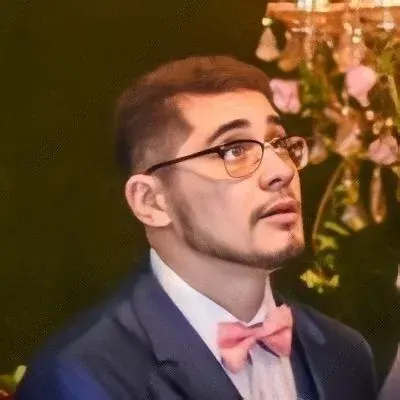
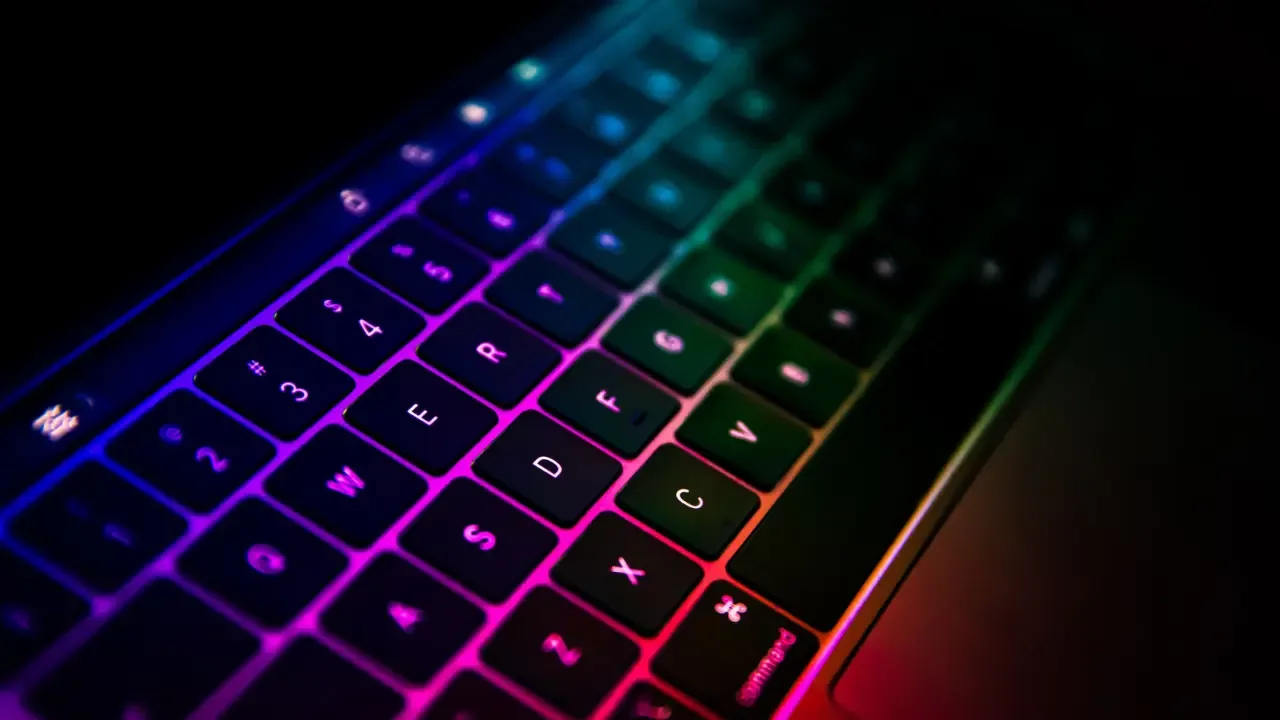
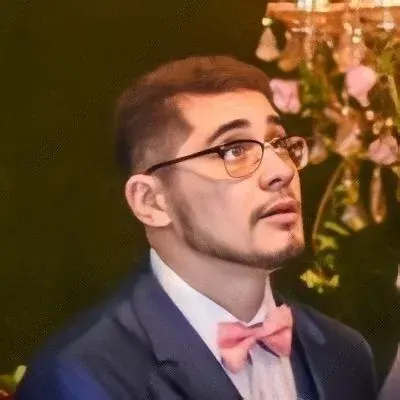
Converting any string into camel case using JavaScript regex 🐫
Have you ever encountered the problem of needing to convert a string into camel case in your JavaScript project? If so, fear not! In this blog post, we will address this common issue and provide you with easy solutions using regular expressions.
The Problem
Let's say you have a string that represents a class name or a variable name, and you want to convert it into camel case. For example, you may encounter variations like:
EquipmentClass name
Equipment className
equipment class name
Equipment Class Name
And you want to transform all of them into: equipmentClassName
.
The Solution
To achieve the desired result, we can use JavaScript's powerful regular expressions to convert any given string into camel case. Here's a simple solution using regex:
function toCamelCase(str) {
return str.replace(/(?:^\w|[A-Z]|\b\w)/g, function(word, index) {
return index === 0 ? word.toLowerCase() : word.toUpperCase();
}).replace(/\s+/g, '');
}
// Usage example
const input = 'Equipment Class Name';
const output = toCamelCase(input);
console.log(output); // Output: equipmentClassName
In this solution, we use the replace
method with a regular expression pattern and a callback function. Let's break it down:
The first
replace
function uses the regex(?:^\w|[A-Z]|\b\w)
.(?:^\w|[A-Z]|\b\w)
matches the start of the string^\w
, any uppercase character[A-Z]
, or a word boundary followed by any word character\b\w
.The callback function determines whether the match is at the beginning of the string or not. If it is, it converts the word to lowercase; otherwise, it converts it to uppercase.
The second
replace
function is used to remove any remaining whitespace by matching the regex\s+
and replacing it with an empty string.
🎉 Take It Further!
Now that you've learned how to convert strings into camel case, you can enhance this function to handle additional scenarios, such as handling special characters or acronyms. You can also encapsulate the functionality in a utility library or create an npm package to share with others. The possibilities are endless!
Don't be shy; experiment with the code and adapt it to your specific needs. And if you come up with something cool, don't forget to share it with the community!
Wrapping Up
Converting any string into camel case using JavaScript regex is no longer a challenging task. By leveraging the power of regular expressions, we can transform various string variations into the desired camel case format. The provided solution serves as a starting point for your own implementation, allowing you to handle different cases and add extra functionality if needed.
So go ahead, give it a try, and transform your strings into beautiful camel case! 🌟
If you have any questions or feedback, feel free to leave a comment below. Happy coding! 💻💪