Converting an object to a string
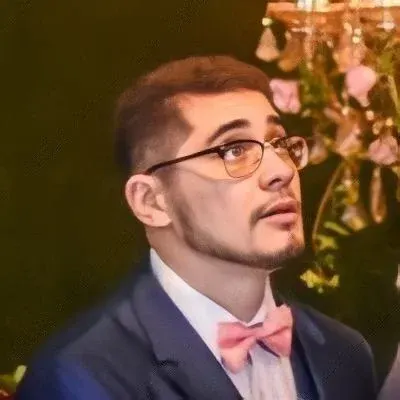
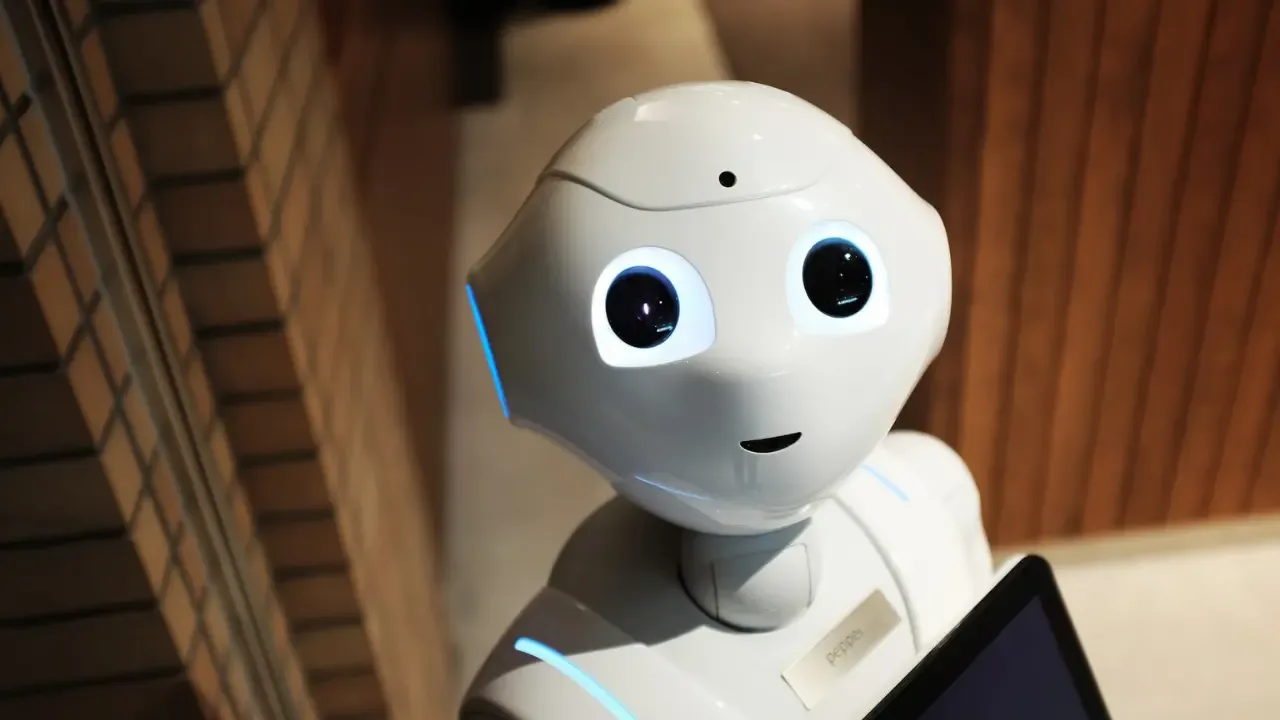
Converting an Object to a String: Say Goodbye to the [object Object] Mystery!
So, you've got a JavaScript object and you want to convert it into a string. Whether you're a seasoned developer or just starting out, this question can leave you scratching your head.
But fear not! In this blog post, we'll explore common issues and provide easy solutions to help you convert objects to strings like a pro. ππ₯
The Mystery of [object Object]
Have you ever tried concatenating a string with your object, just to be greeted by the infamous [object Object]? π€
Let's take a look at the example provided:
var o = {a:1, b:2};
console.log(o);
console.log('Item: ' + o);
The output might surprise you:
Object { a=1, b=2} // very nice readable output :)
Item: [object Object] // no idea what's inside :(
Yikes! That's not what we expected, right? It seems like JavaScript is not able to magically understand how we want to represent our object as a string.
Solution 1: JSON.stringify() to the Rescue π¦ΈββοΈ
One popular solution is to use the JSON.stringify()
method. This method takes an object as a parameter and returns a string representation of that object. Let's see it in action with the same example:
var o = {a:1, b:2};
console.log(o);
console.log('Item: ' + JSON.stringify(o));
And here's the new output:
Object { a=1, b=2} // still nice and readable :)
Item: {"a":1,"b":2} // a string representation of our object! π
Whoa! JSON.stringify() did the trick. It successfully converted our object to a string representation, allowing us to see the actual contents.
But wait, there's more! JSON.stringify() also accepts additional parameters to control the formatting, such as adding line breaks and indentation. Check out the MDN documentation for more details on these options.
Solution 2: The toString() Method π‘
Another solution worth mentioning is using the toString()
method. Yep, just like that!
Let's modify our example to utilize the toString()
method:
var o = {a:1, b:2};
console.log(o);
console.log('Item: ' + o.toString());
And voilΓ , we have the output we desired:
Object { a=1, b=2} // still nice and readable :)
Item: [object Object] // no more mystery π
While toString()
might not provide a detailed string representation like JSON.stringify(), it can be handy for simple object conversions, especially if you want to eliminate that pesky [object Object].
Time to Put Your Skills to the Test! πͺ
Now that you know how to convert objects to strings, why not try it out on your own? Experiment with different objects and see the magic happen.
And remember, there's always more to learn and explore in the world of JavaScript!
If you have any questions or cool conversion techniques you'd like to share, leave a comment below. Let's keep the conversation going! π£οΈπ¬
Happy coding! ππ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
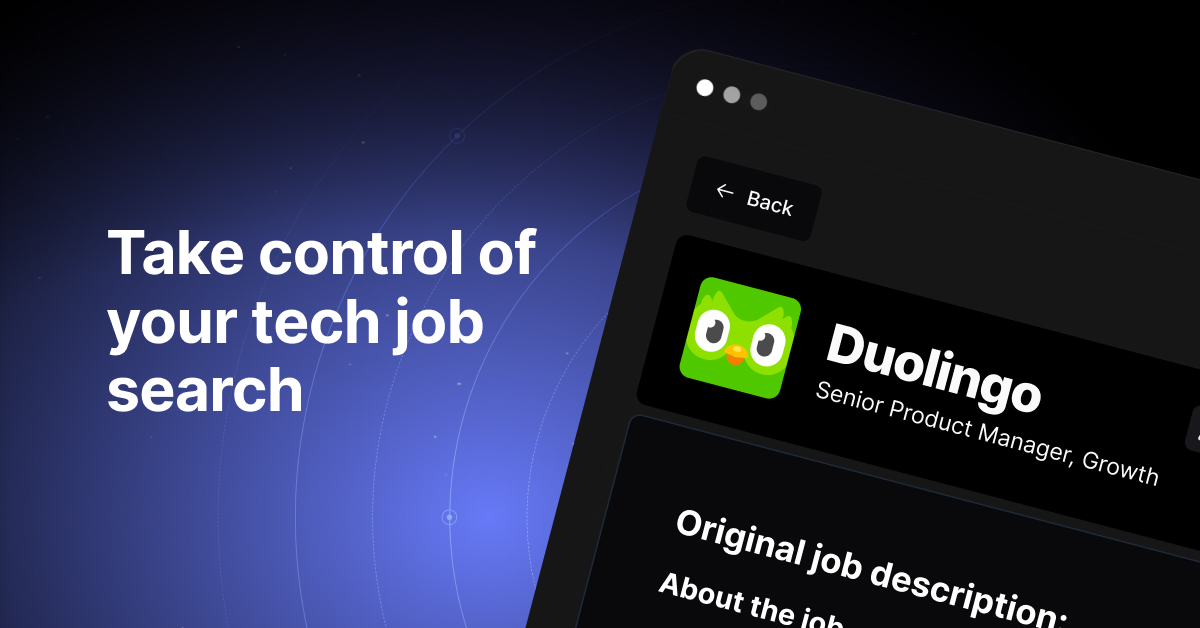