Converting a string to JSON object
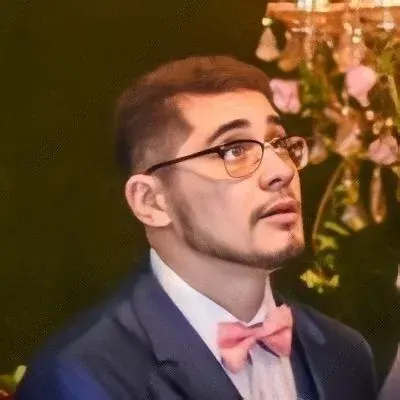
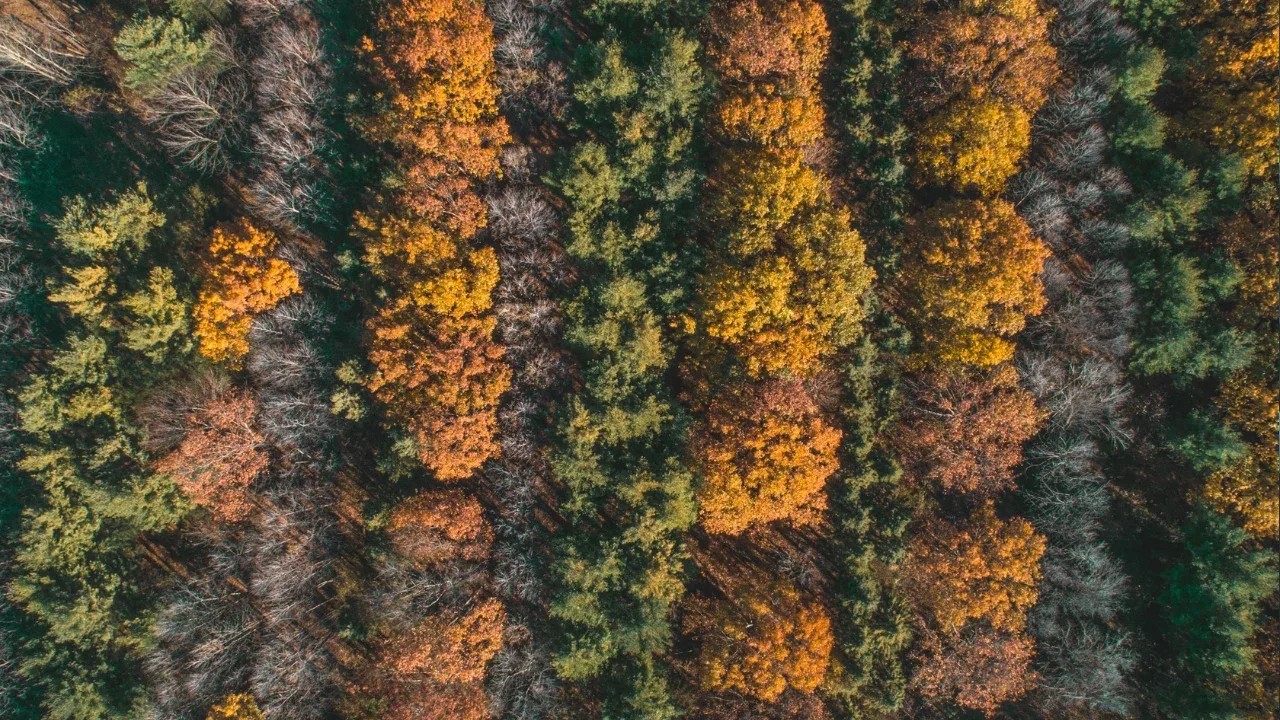
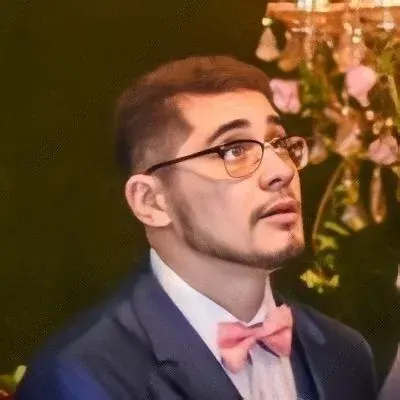
Converting a String to JSON: A Simple Guide 🔄
Are you struggling with converting a string to a JSON object in JavaScript? 🤔 Don't worry! We've got you covered. In this guide, we'll address common issues and provide you with easy solutions to tackle this problem. Let's dive in and make JavaScript believe that your string is a JSON object! 💪
The Problem: Making JS Think Your String is JSON 🤷♀️
So, you have a function that only works if a JSON object is passed to it. However, when you pass a string with the same JSON format, it fails to work. You've also noticed that sending the string through an Ajax request with the "handle as" parameter set to "JSON" solves the problem. 🌐
Possible Solution: Converting the String to JSON 🔄
To make your string compatible with your function, you need to convert it to a JSON object. Luckily, JavaScript provides the JSON.parse()
method to achieve this. This method allows you to parse a JSON-encoded string and transform it into a JavaScript object. 🚀
Here's an example of how you can convert your string to JSON using JSON.parse()
:
const jsonString = `{
"data": [
{
"id": "id1",
"fields": [
{
"id": "name1",
"label": "joker",
"unit": "year"
},
{
"id": "name2",
"label": "Quantity"
}
],
"rows": [
// data here....
]
}
]
}`;
const jsonObject = JSON.parse(jsonString);
Now, jsonObject
contains your converted string as a JSON object, and you can pass it to your function with confidence. ✨
The "String to JSON" Compatibility Check 🔄💻
Sometimes, your string might not be in the correct JSON format, leading to parsing errors. To ensure compatibility, you can validate your string using the try...catch
block. Wrap the JSON.parse()
method in a try
block and catch any errors that might occur. This way, you can handle any invalid strings gracefully. ⚡
Here's an example:
let jsonString = ''; // Your string here
try {
const jsonObject = JSON.parse(jsonString);
// Pass the JSON object to your function
} catch (error) {
console.error('Invalid JSON string:', error);
// Handle the error gracefully
}
Call-to-Action: Share Your Experience and Engage! 🙌
There you have it! Converting a string to JSON object is as easy as pie. 🍰 Now that you know how to make JavaScript think your string is JSON, why not put it into practice and try it out yourself?
If you found this guide helpful, make sure to share it with your fellow developers. Also, feel free to comment below and let us know how you successfully converted your string to JSON. We'd love to hear your experiences and learn from you! 💬
Happy coding! 💻✨