Converting a JS object to an array using jQuery
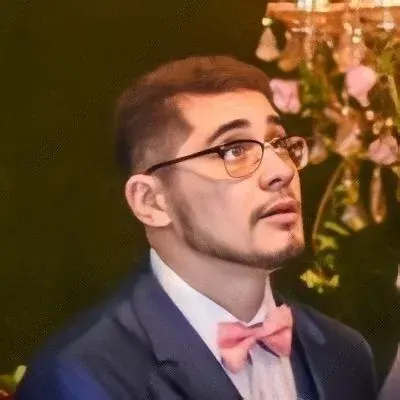
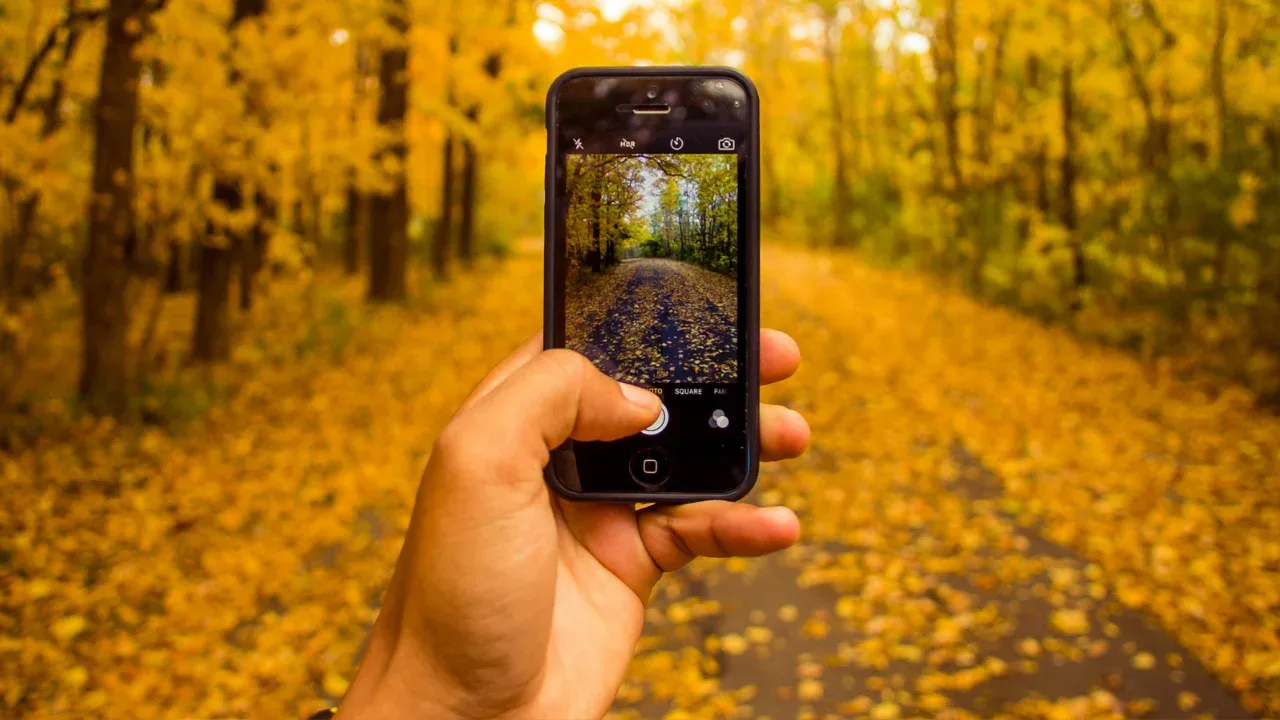
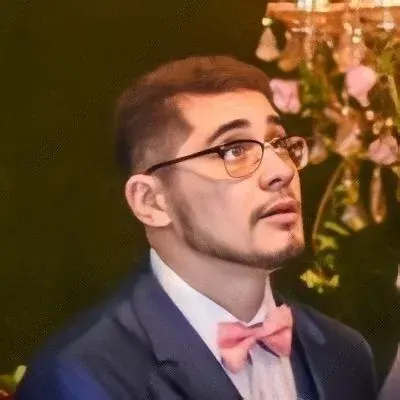
Converting a JavaScript Object to an Array using jQuery 🔄
So, you have a JavaScript object but you need it to be transformed into an array. No worries, I got your back! 💪
Let's start with the problem at hand. You have this JavaScript object:
myObj = {1: [Array-Data], 2: [Array-Data]}
But what you actually need is an array in this format:
array[1]: [Array-Data]
array[2]: [Array-Data]
Your initial attempt was to iterate through the object using $.each
and push each element into an empty array. 🔄 While this method does work, there's a more elegant and concise solution available!
Solution using Object.values()
method 💡
The easiest and most efficient way to convert an object to an array is by using the Object.values()
method. This method returns an array of the object's own property values, in the same order as provided by a for...in loop.
Here's how you can do it:
const myArray = Object.values(myObj);
That's it! 🎉 myArray
now contains the values from myObj
as an array. You can access the individual elements using their respective indices.
Using a Dedicated Function 📚
If you find yourself needing to perform this object-to-array conversion frequently, you might consider creating a reusable function to simplify the process.
Here's an example of how you can create a function called convertObjectToArray
that handles this conversion:
function convertObjectToArray(obj) {
return Object.values(obj);
}
Now, whenever you need to convert an object to an array, simply call this function, passing in your desired object. Easy peasy! 😄
Closing Thoughts and a Call-to-Action 🚀
By leveraging the power of the Object.values()
method, converting a JavaScript object to an array has never been easier! Save time and simplify your code with just one line of code.
So go ahead, try out this new approach and let us know how it works for you! If you have any further questions or want to share your experience, feel free to leave a comment below. Happy coding! 💻💡
Have you encountered any other tricky JavaScript problems? Check out our blog for more helpful tips and tutorials!