Convert XML to JSON (and back) using Javascript
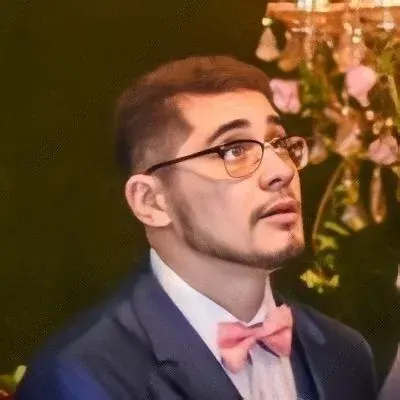
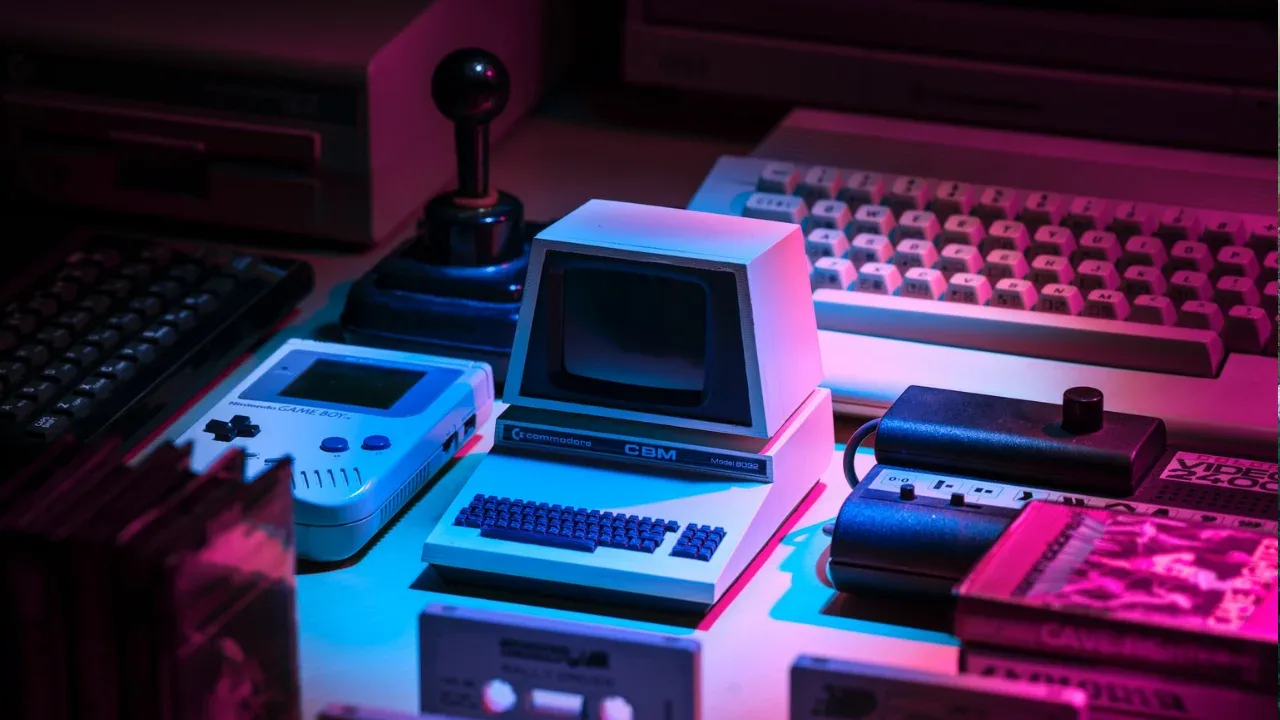
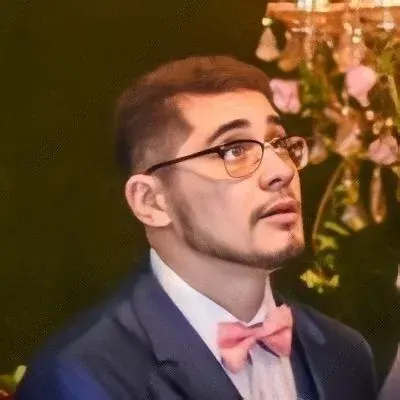
Convert XML to JSON (and back) using JavaScript: An Easy Guide 🔄
Have you ever found yourself needing to convert XML to JSON and then back to XML? 🔄 It can be a tricky task that requires some knowledge of JavaScript. In this blog post, we will explore some common issues and provide easy solutions to help you tackle this problem. So, let's dive in!
The XML to JSON Challenge 🧩
Converting XML to JSON and vice versa is not always straightforward. One common issue is finding a consistent and reliable tool that can handle this conversion seamlessly. While there are several tools available, they might not always deliver the desired results.
✨ xml2json ✨ is one such tool that can help you convert XML to JSON. You can find it here. However, keep in mind that it might not be completely consistent.
The Solution: JavaScript Magic 🎩✨
To convert XML to JSON in JavaScript, you can leverage the power of built-in methods. Here's an example:
const xmlString = `<root>
<name>John Doe</name>
<age>25</age>
</root>`;
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(xmlString, 'text/xml');
const jsonString = JSON.stringify(xml2json(xmlDoc));
console.log(jsonString);
In the code snippet above, we:
Define the XML string that we want to convert.
Create a new
DOMParser
object to parse the XML string into an XML document.Use the
JSON.stringify
method to convert the XML document to a JSON string.Log the resulting JSON string to the console.
By executing this code, you should see the JSON representation of your XML data. 🎉
JSON to XML: The Reverse Journey ⬅️🔄
Now that we have successfully converted XML to JSON, how can we go back to XML? Fear not, we've got you covered!
To convert JSON back to XML, you can use the following code snippet:
const jsonObject = {
"root": {
"name": "John Doe",
"age": "25"
}
};
const jsonString = JSON.stringify(jsonObject);
const xmlDoc = xml2json.toXml(jsonString);
console.log(xmlDoc);
In the code example above, we:
Define a JavaScript object representing the JSON data.
Use
JSON.stringify
to convert the JavaScript object to a JSON string.Call the
toXml
method provided by xml2json to convert the JSON string back to an XML document.Log the resulting XML document to the console.
Voilà! You have successfully converted JSON back to XML. 🥳
Conclusion and Call-to-Action 💡🤩
Converting XML to JSON and vice versa using JavaScript doesn't have to be a daunting task. By following the examples and solutions provided in this blog post, you can confidently tackle this challenge. Remember to explore different tools and libraries, and find the one that works best for your specific requirements.
If you have any other tips, tricks, or experiences related to XML to JSON conversion, feel free to share them in the comments below. Let's help each other out! 👇💬
Now, it's your turn to give it a try! Take some XML data, convert it to JSON, then back to XML using the code snippets provided. Share your results and let us know how it goes. Happy coding! 😄🚀