Convert UTC date time to local date time
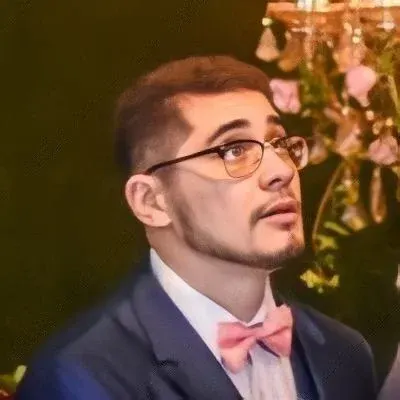
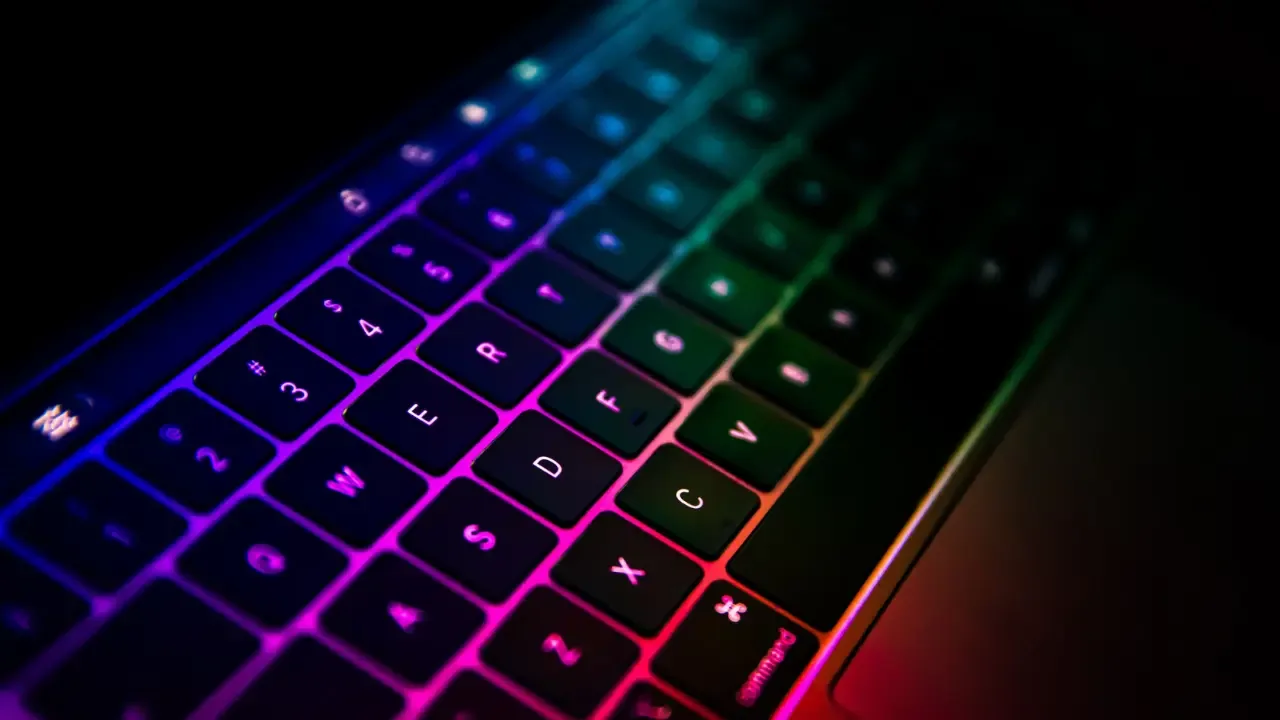
🌎 Convert UTC date time to local date time 📅
Ever come across a situation where you had to deal with date and time conversions between different time zones? It can be a tricky task, especially when you need to convert a UTC (Coordinated Universal Time) date and time to the local date and time of a user's browser. In this blog post, we will walk you through common issues you might face and provide easy solutions using JavaScript or jQuery. Let's dive in! 💦
The Challenge ⚔️
Imagine you have a server-side application that returns a datetime variable in UTC format, like this: 6/29/2011 4:52:48 PM
. However, you want to display this date and time in the current user's browser time zone. How can you achieve this using JavaScript or jQuery?
The Solution 🎉
JavaScript Solution ✨
JavaScript provides the toLocalString()
method to convert a UTC date and time to the local date and time of the user's browser. Here's an example code snippet to illustrate the conversion:
// Get the UTC date and time from the server
const utcDateTimeString = '6/29/2011 4:52:48 PM';
// Create a new Date object with the UTC date and time
const utcDateTime = new Date(utcDateTimeString);
// Convert the UTC date and time to local date and time
const localDateTime = utcDateTime.toLocaleString();
// Display the local date and time
console.log(localDateTime);
By using the toLocaleString()
method, JavaScript automatically converts the UTC date and time to the user's local date and time format based on their browser settings. 🌐
jQuery Solution 🌟
If you're using jQuery in your project, you can leverage the power of its Moment.js library for date and time manipulations. Here's an example using Moment.js to convert UTC date and time to the user's local date and time:
// Get the UTC date and time from the server
const utcDateTimeString = '6/29/2011 4:52:48 PM';
// Convert the UTC date and time to the user's local date and time using Moment.js
const localDateTime = moment.utc(utcDateTimeString).local().format('YYYY-MM-DD HH:mm:ss');
// Display the local date and time
console.log(localDateTime);
By utilizing moment.utc()
with local()
and format()
functions, Moment.js takes care of the UTC to local date and time conversion effortlessly. 🕰️
Call to Action ✋
Now that you know how to convert UTC date and time to the local date and time of a user's browser using JavaScript or jQuery, it's time to put your newfound knowledge into action! Experiment with the code snippets provided and see the magic happen. Feel free to share your insights and any additional tips or solutions you have in the comments section below. Happy coding! 💻✨
Did you find this blog post helpful? Share it with your fellow developers and spread the knowledge! 🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
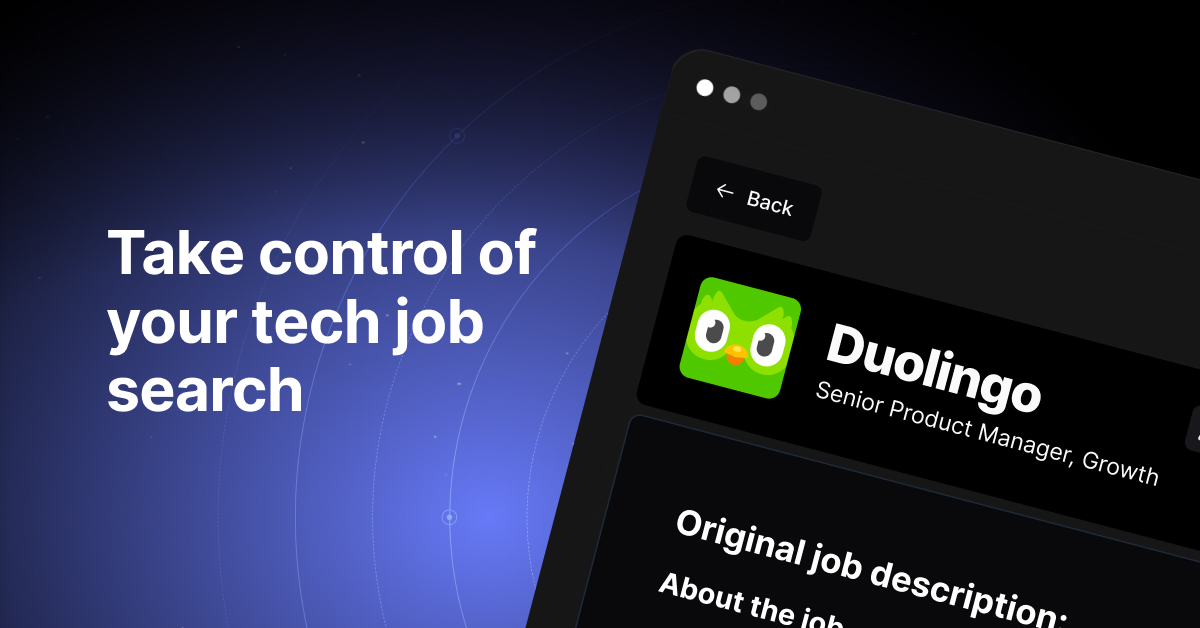