Convert object array to hash map, indexed by an attribute value of the Object
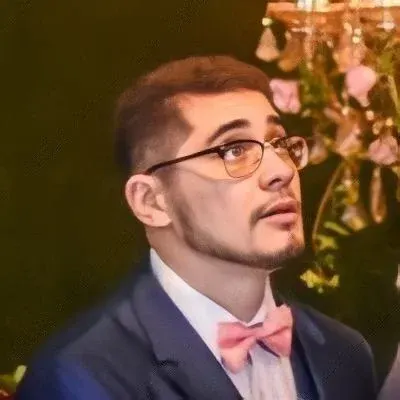
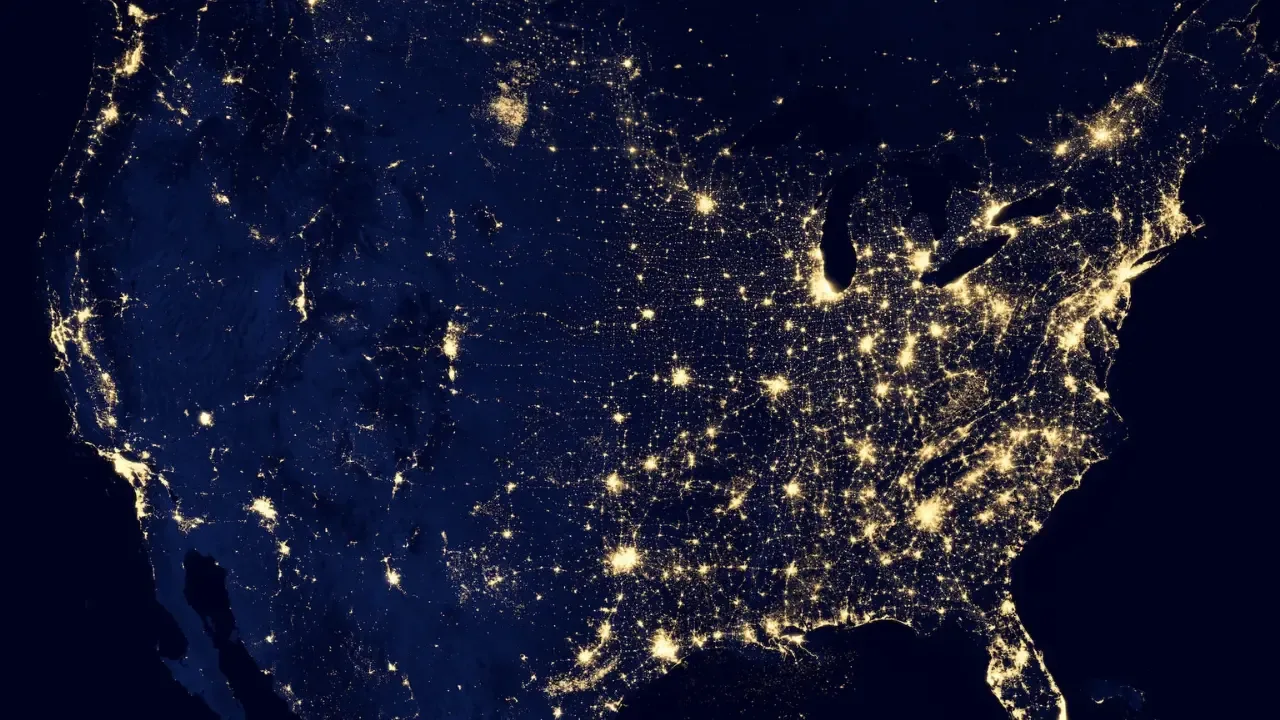
Convert object array to hash map with πJavaScript! πππΊοΈ
Are you tired of dealing with arrays of objects and want to convert them into a more organized and efficient data structure? π€ Well, look no further because we have a solution for you! π‘ In this blog post, we will guide you through the process of converting an array of objects into a hash map, indexed by a specific attribute value of the object. ππ
Use Case
Before we dive into the code, let's understand the use case for converting an array of objects into a hash map. π The main purpose of this conversion is to create a more easily searchable and retrievable data structure. This becomes especially handy when you need to access specific objects based on a unique identifier. π§©
For example, let's say you have an array of objects representing users, with each object having a unique id
attribute. By converting this array into a hash map indexed by the id
attribute, you can quickly retrieve user objects by their id
without iterating through the entire array. π
The Code
Here's a snippet of JavaScript code that allows you to convert an array of objects into a hash map. ππ’
function isFunction(func) {
return Object.prototype.toString.call(func) === '[object Function]';
}
/**
* This function converts an array to a hash map
* @param {String | function} key - describes the key to be evaluated in each object to use as the key for the hash map
* @returns Object - the hash map
* @Example
* [{id:123, name:'naveen'}, {id:345, name:'kumar'}].toHashMap('id')
* Returns: Object {123: Object, 345: Object}
*
* [{id:123, name:'naveen'}, {id:345, name:'kumar'}].toHashMap(function(obj){return obj.id + 1;})
* Returns: Object {124: Object, 346: Object}
*/
Array.prototype.toHashMap = function(key) {
var _hashMap = {}, getKey = isFunction(key) ? key : function(_obj) { return _obj[key]; };
this.forEach(function(obj) {
_hashMap[getKey(obj)] = obj;
});
return _hashMap;
};
Explanation
Let's break down the code together. π§©
We start by defining a helper function
isFunction
that checks if a given variable is a function. This will come in handy when we allow thekey
parameter to be either a string or a function.Next, we define the
toHashMap
function as a prototype method of theArray
object. This allows us to easily call this function on any array.The
toHashMap
function takes akey
parameter, which can be either a string or a function. This defines the attribute value of the object that will be used as the key for the hash map.Inside the function, we initialize an empty object
_hashMap
to store the key-value pairs of the hash map.We also define a variable
getKey
that is either set to thekey
parameter itself (if it is a function) or a function that retrieves the attribute value from the object.Using the
forEach
method, we iterate over each object in the array.For each object, we evaluate the key using the
getKey
function and assign the object itself as the value in the hash map.Finally, we return the completed hash map.
Conclusion
Congratulations! π You now know how to easily convert an array of objects into a hash map using JavaScript. This simple and efficient solution will save you time and make your code more readable and maintainable. ππ€©
Feel free to check out the complete code snippet on GitHub Gist for easy reference and implementation.
So go ahead, give it a try and level up your code organization with hash maps! Don't forget to share your thoughts and experiences in the comments below. We can't wait to hear from you! π¬β€οΈ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
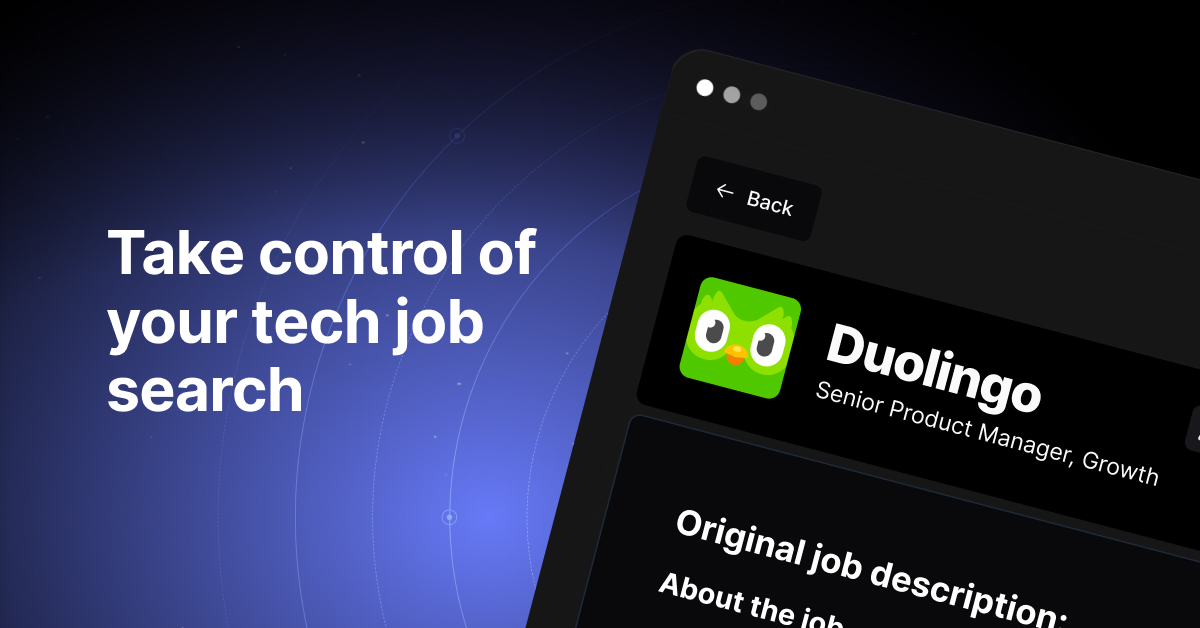