Convert JS object to JSON string
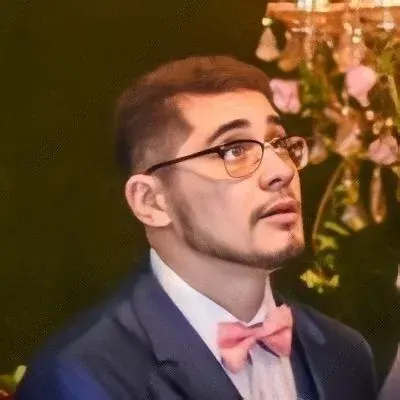
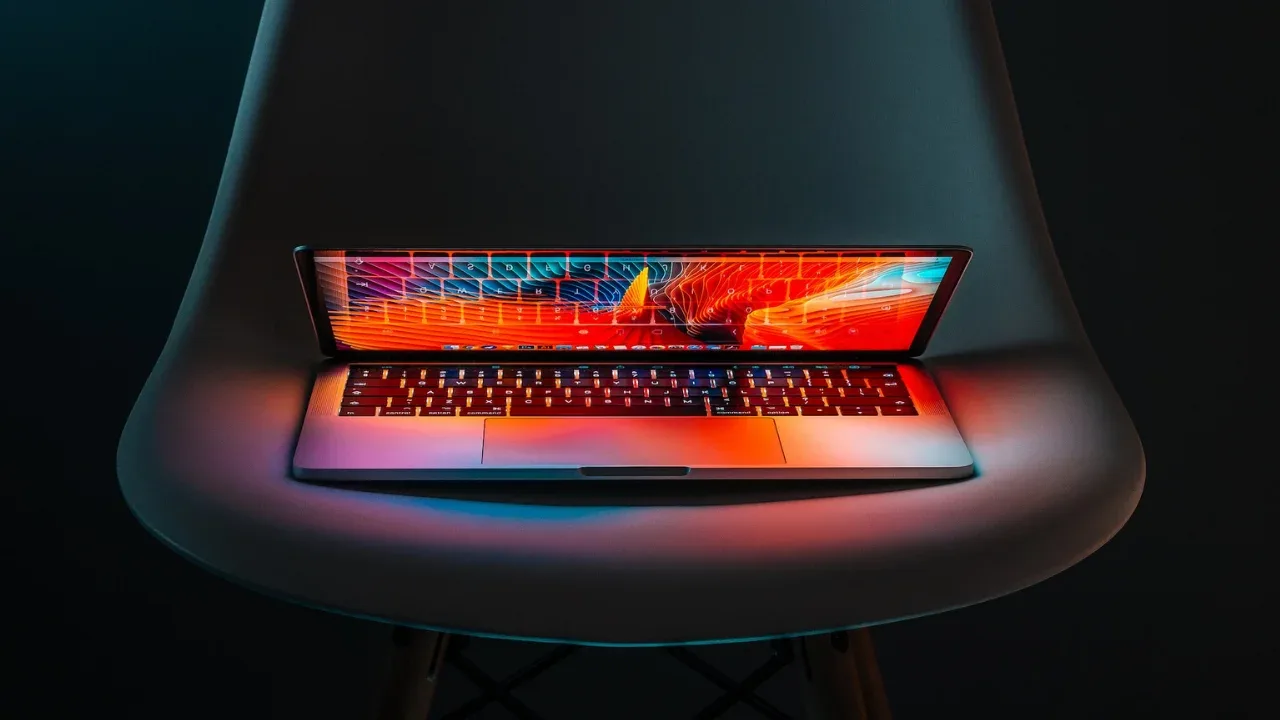
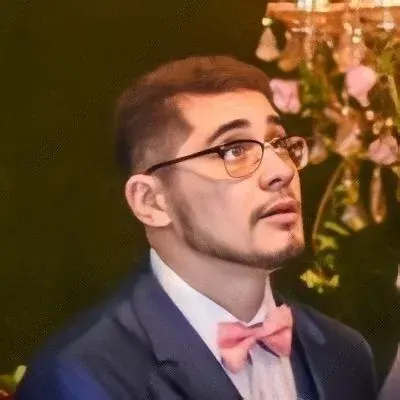
💡 Converting JS Object to JSON String: A Simple Guide
So, you've been working with JavaScript and stumbled upon the need to convert a JavaScript object to a JSON string, huh? Don't fret! We're here to demystify this process for you. In this guide, we'll address common issues and provide you with easy solutions to help you get the JSON string you desire. Let's dive in! 🏊♀️
Understanding the Problem
Let's start by understanding the context. Suppose we have the following JavaScript object defined:
var j = { "name": "binchen" };
Our goal is to convert this object to a JSON string with the following output: '{"name":"binchen"}'
.
Solution: JSON.stringify()
Our knight in shining armor is the JSON.stringify()
function. This function allows us to convert JavaScript objects to JSON strings effortlessly. 🌟
Here's how we can apply it to our example:
var j = { "name": "binchen" };
var jsonString = JSON.stringify(j);
That's it! 🎉 By invoking JSON.stringify()
, we obtain the desired JSON string.
Handling Complex Objects
Great job so far! But what if our JavaScript object is nested or contains complex data types like Date objects or functions? How do we handle those cases? Let's explore some additional scenarios.
Handling Nested Objects
Suppose we have the following JavaScript object with nested properties:
var person = {
"name": "John",
"age": 30,
"address": {
"street": "123 Main St",
"city": "New York"
}
};
To convert this nested object to a JSON string, we can simply use JSON.stringify()
:
var jsonString = JSON.stringify(person);
The resulting JSON string will look like this:
{
"name": "John",
"age": 30,
"address": {
"street": "123 Main St",
"city": "New York"
}
}
Handling Complex Data Types
If our object contains complex data types like Dates or functions, JSON.stringify()
will treat them in a special way:
var obj = {
"date": new Date(),
"sayHello": function() {
console.log("Hello!");
}
};
var jsonString = JSON.stringify(obj);
When we convert this object to a JSON string, the Date object will be represented as a string, and the function will be excluded:
{"date":"2022-02-18T09:30:00.000Z"}
Call-to-Action: Share Your Thoughts!
Congratulations! Now you know how to convert JavaScript objects to JSON strings using JSON.stringify()
. We hope this guide has been helpful to you. If you have any other questions or tips on this topic, we'd love to hear from you! Share your thoughts in the comments section below, and don't forget to share this post with your fellow developers. Happy coding! 😄👋