Convert JavaScript String to be all lowercase
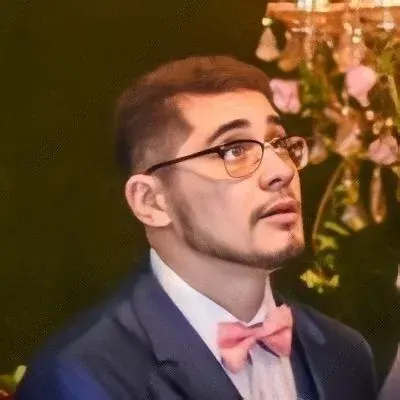
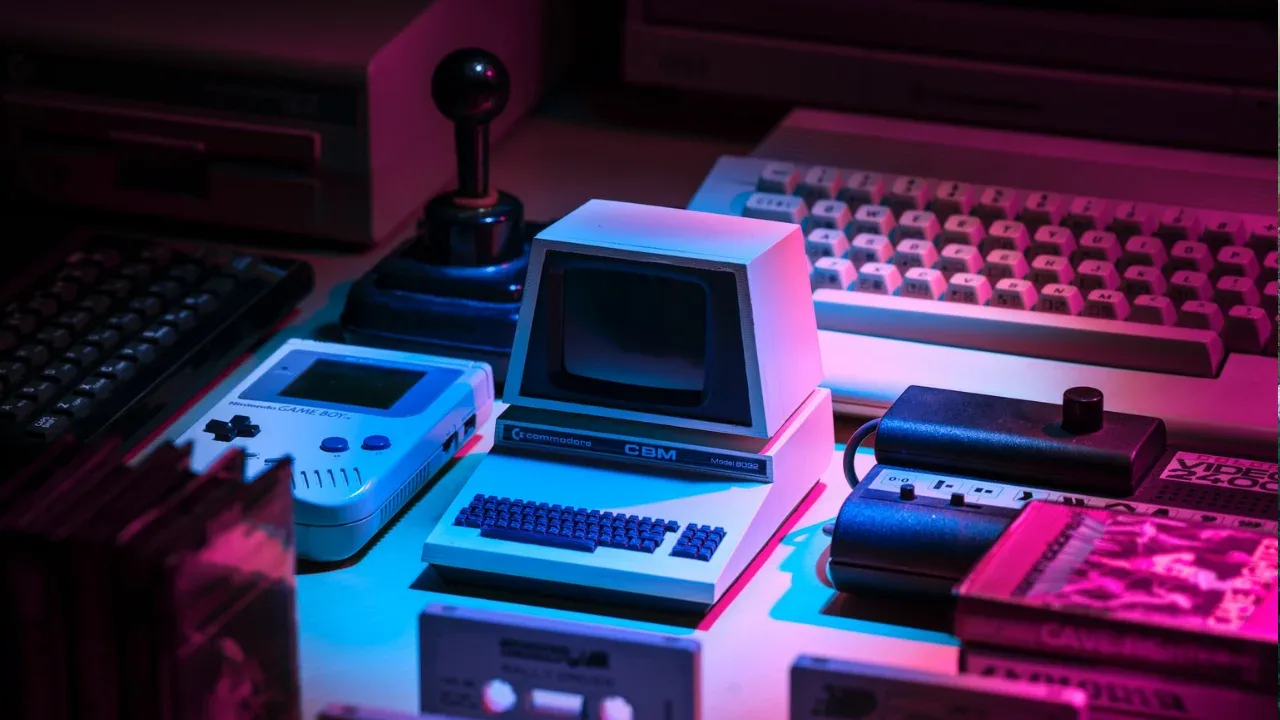
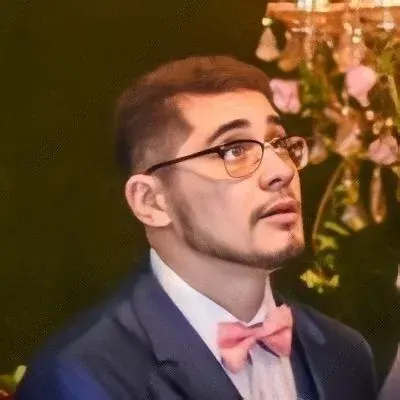
Convert JavaScript String to All Lowercase: Easy Solutions and Fun Examples! ππ€
So you want to transform a JavaScript string to all lowercase letters, huh? No worries, my friend! In this blog post, Iβve got you covered with simple solutions and some cool examples to make things super easy for you! Let's jump right in! π
The Common Issues and the Problem Statement π€
The main issue here is that JavaScript doesn't provide a built-in method to convert strings to lowercase. However, fear not! We have some easy-peasy workarounds that will get the job done and make your string lowercase in no time! πͺ
Easy Solutions to the Rescue! π¦ΈββοΈ
Here are a few ways you can convert a JavaScript string to all lowercase letters:
Solution 1: Using the toLowerCase()
method
The toLowerCase()
method is a JavaScript function that returns the calling string value converted to lowercase. It's as simple as that! Let me show you an example:
const originalString = "Your Name";
const lowercaseString = originalString.toLowerCase();
console.log(lowercaseString); // Output: "your name"
As you can see, calling toLowerCase()
on the original string gives you the desired lowercase output. Easy, right? π
Solution 2: Using the replace()
method with a regular expression
If you're feeling a bit adventurous, you can also use the replace()
method with a regular expression to replace all uppercase letters with their lowercase versions. Here's how it works:
const originalString = "Your Name";
const lowercaseString = originalString.replace(/[A-Z]/g, (match) => match.toLowerCase());
console.log(lowercaseString); // Output: "your name"
In this example, we're using the regular expression /[A-Z]/g
to match all uppercase letters in the string and then converting them to lowercase using the (match) => match.toLowerCase()
callback function. Voila! Your string is now lowercase. π
Examples to Make It Fun! π
Let's brighten up your learning experience with some fun examples! Imagine you have a variable called superheroName
with different uppercase superhero names. We can convert them to lowercase using the solutions we just discussed:
const superheroName = "SUPERMAN";
console.log(superheroName.toLowerCase()); // Output: "superman"
const superheroName2 = "WONDER WOMAN";
console.log(superheroName2.replace(/[A-Z]/g, (match) => match.toLowerCase())); // Output: "wonder woman"
Feel the power of JavaScript as it transforms those uppercase superhero names into lowercase awesomeness! π¦ΈββοΈ
Join the Coding Adventure! π»
Now that you have learned the easiest ways to convert a JavaScript string to all lowercase, it's your turn to explore and have fun with it! Try it on your own strings and see what cool transformations you can make! Share your awesome code snippets and outcomes in the comments below! π€©
Remember, coding is not just about finding solutions but also about unleashing your creativity! Let's conquer the world of lowercase strings together! πͺπ»
Keep coding and happy lowercase converting! πβ¨