Convert form data to JavaScript object with jQuery
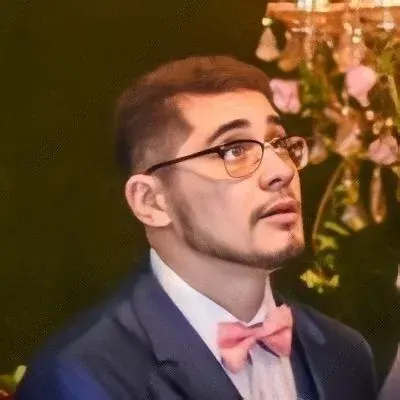
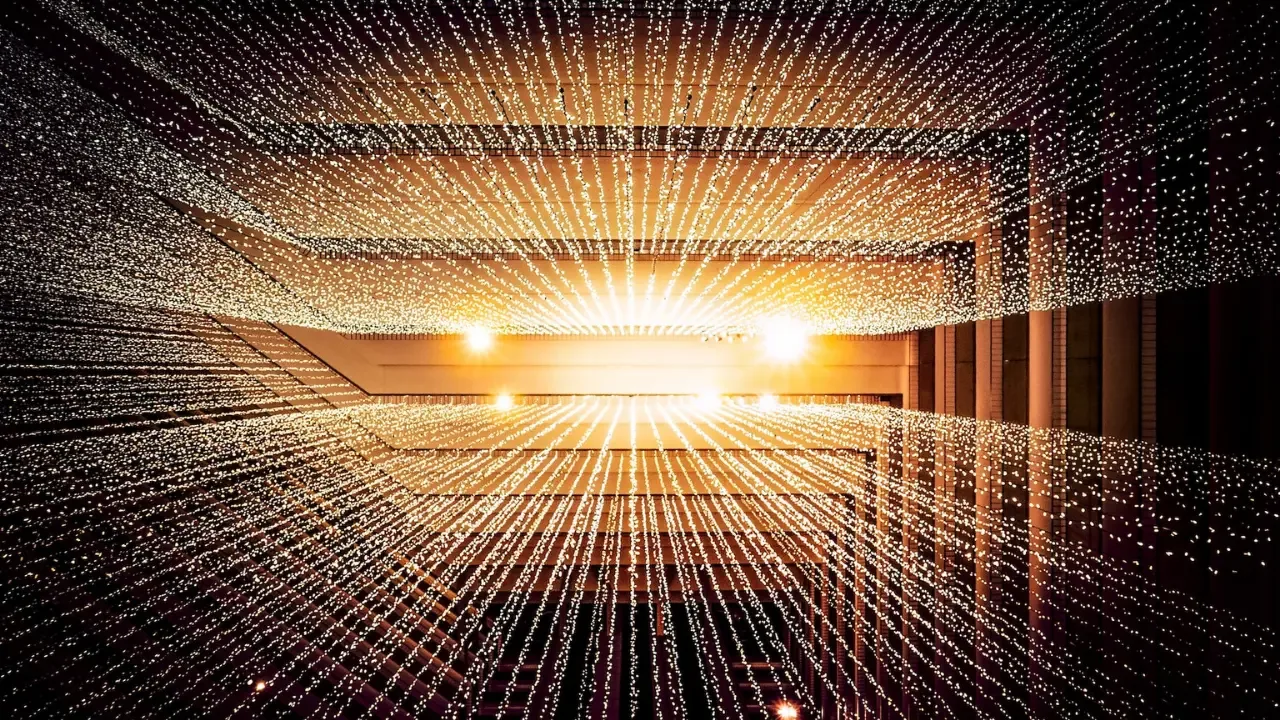
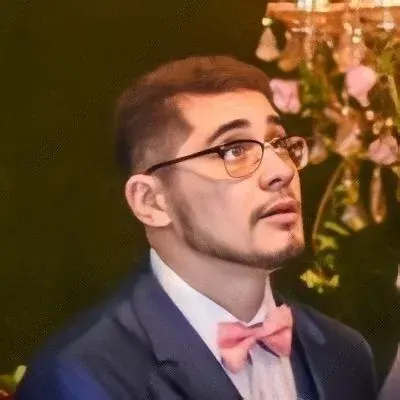
📝 Blog Post: Convert Form Data to JavaScript Object with jQuery
Are you tired of manually converting form data to a JavaScript object? 😩 Have you been searching for an easy solution that doesn't involve cumbersome loops and complex functions? 🤔 Well, you're in luck! In this blog post, we'll explore how you can convert all elements of your form into a JavaScript object using jQuery. 🚀
The Problem: Converting Form Data
The question at hand is: How do I convert all elements of my form to a JavaScript object? 🧐 We want to achieve this without using the string returned by $('#formid').serialize();
or the map returned by $('#formid').serializeArray();
.
The Solution: jQuery's .serializeArray()
and .reduce()
Method
Fear not, because there is an elegant solution that will save you time and effort! 😌 We can leverage jQuery's .serializeArray()
method to get the form data as an array of objects. Then, using JavaScript's .reduce()
method, we can convert this array into a single JavaScript object. 💪
Here's how you can do it:
// Get the form data as an array of objects
var formArray = $('#formid').serializeArray();
// Convert the array to a JavaScript object
var formData = formArray.reduce(function(obj, item) {
obj[item.name] = item.value;
return obj;
}, {});
console.log(formData);
In this code snippet, we first use $('#formid').serializeArray();
to get the form data as an array of objects. Then, we use the .reduce()
method to iterate over each element of the array and build our JavaScript object. Finally, we log the resulting object, formData
, to the console.
Common Issues: Validation and Nested Forms
While this solution works great for simple forms, there are a couple of common issues you may encounter. 🤷♀️
1. Validation:
If your form includes any validation rules or required fields, you'll need to handle those separately. The code snippet above assumes that all form elements have valid values. 🚫
2. Nested Forms:
If your form contains nested forms or form elements with the same name, the resulting JavaScript object may not represent the structure you desire. In such cases, you may need to modify the code snippet to handle these specific scenarios. 🔀
Call-to-Action: Share Your Thoughts and Experiences! 🗣️
Now that you have a solution to convert form data to a JavaScript object with ease, give it a try and let us know how it works for you! Do you have any additional tips or techniques? Did you encounter any unique challenges? Share your thoughts and experiences in the comments below. We can't wait to hear from you! 😄
Remember, sharing is caring! If you found this blog post helpful, don't forget to share it with your friends and colleagues who might also find it valuable. Together, we can make web development easier and more enjoyable for everyone! 🌐💻
Happy coding! 💙
[Insert your blog's signature and social media links here]